Question
DateClient.java: //A java client program to request a response from DateServer through a Socket // connection (host=localhost, port=6013) import java.net.*; import java.io.*; public class DateClient
DateClient.java:
//A java client program to request a response from DateServer through a Socket
// connection (host="localhost", port=6013)
import java.net.*;
import java.io.*;
public class DateClient
{
// the default port
public static final int PORT = 6013;
// this could be replaced with an IP address or IP name
public static final String host = "localhost";
public static void main(String[] args) throws IOException {
BufferedReader fromServer = null;
Socket server = null;
try {
// create socket and connect to default port
server = new Socket(host, PORT);
// read the date and close the socket
fromServer = new BufferedReader(new InputStreamReader(server.getInputStream()));
String line;
while ( (line = fromServer.readLine()) != null)
System.out.print(line);
// closing the input stream closes the socket as well
fromServer.close();
} catch (IOException ioe) {
System.err.println(ioe);
}
finally {
// let's close streams and sockets
if (fromServer!= null)
fromServer.close();
if (server != null)
server.close();
}
}
}
DateServer.java
// A java server to respond to any client connection with the date information
import java.net.*;
import java.io.*;
public class DateServer
{
// the default port
public static final int PORT = 6013;
public static void main(String[] args) throws java.io.IOException {
Socket client = null;
ServerSocket sock = null;
byte [] bbuffer = new byte [1024];
String line;
int numbytes = 0;
DataOutputStream toClient = null;
try {
// create a server socket and bind it to default port
sock = new ServerSocket(PORT);
while (true) {
// await client connections
client = sock.accept();
// obtain the output stream and deliver the date
toClient = new DataOutputStream(client.getOutputStream());
java.util.Date theDate = new java.util.Date();
toClient.writeBytes(theDate.toString() + " ");
// closing the output stream closes the socket as well
toClient.close();
}
} catch (java.io.IOException ioe) {
System.err.println(ioe);
}
finally {
// let's close streams and sockets
if (toClient!= null)
toClient.close();
if (client != null)
client.close();
if (sock != null)
sock.close();
}
}
}
l. 120%) Modify the DateClient-Server program without changing its functionality as specified below: DateClient uses a BufferedInputStream (instead of a BufferedlnputReader) for the input from the server, and DateServer uses a PrintWriter (instead of a DataOutputStream) for the output to the clientStep by Step Solution
There are 3 Steps involved in it
Step: 1
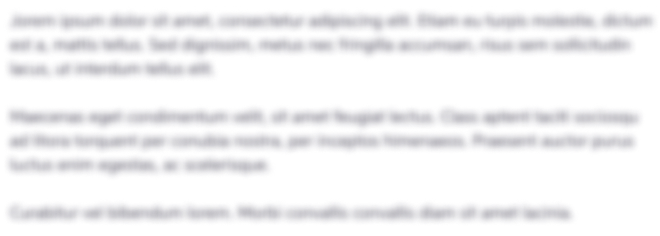
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started