Question
Debug assertion failed. Can someone help? Can someone change the code for me? The problem is on the bottom and I will attach my code.
Debug assertion failed. Can someone help? Can someone change the code for me? The problem is on the bottom and I will attach my code.
#include
#include
#include
#include
#include
using namespace std;
void accept(int intArray[], long int s);
void display(int intArray[], long int s);
void insertionSort(int intArray[], long int s);
void ssort(int intArray[], long int s);
void bubbleSort(int intArray[], long int s);
void quickSort(int intArray[], int low, long int high);
int partition(int intArray[], int low, long int high);
void swap(int* a, int* b);
void mergeSort(int *a, int low, long int high);
void merge(int *a, int low, long int high, long int mid);
void findMaxHeap(int a[], int i, long int n);
void heapSort(int a[], long int n);
void generateMaxHeap(int a[], long int n);
int main(int argc, char *argv[]) {
int a, i = 0;
long int n;
int *intArray;
string sortType, sortName;
string inputFileName, outputFileName;
sortType = argv[1];
inputFileName = argv[2];
outputFileName = argv[3];
std::fstream inputFile(inputFileName, std::ios_base::in);
inputFile >> n;
intArray = new int[n];
while (inputFile >> a) {
intArray[i] = a;
i++;
}
int start_s = clock();
if (sortType == "B") {
bubbleSort(intArray, n);
sortName = "Bubble Sort";
}
else if (sortType == "I") {
insertionSort(intArray, n);
sortName = "Insertion Sort";
}
else if (sortType == "M") {
mergeSort(intArray, 0, n);
sortName = "Merge Sort";
}
else if (sortType == "H") {
heapSort(intArray, n - 1);
sortName = "Heap Sort";
}
else if (sortType == "Q") {
quickSort(intArray, 0, n);
sortName = "Quick Sort";
}
else{
cout
}
int stop_s = clock();
cout
return 0;
}
// Method to perform insertion sort
void insertionSort(int intArray[], long int s){
int I, J, Temp;
for (I = 1; I
Temp = intArray[I];
J = I - 1;
while ((Temp
intArray[J + 1] = intArray[J];
J--;
}
intArray[J + 1] = Temp;
}
}
// Method to perform bubble sort
void bubbleSort(int intArray[], long int s){
int I, J, Temp;
for (I = 0; I
for (J = 0; J
if (intArray[J]>intArray[J + 1]){
Temp = intArray[J];
intArray[J] = intArray[J + 1];
intArray[J + 1] = Temp;
}
}
}
// Method to provide partiotion for quick sort
int partition(int intArray[], int low, long int high){
int pivot = intArray[high];
int i = (low - 1);
for (int j = low; j
if (intArray[j]
i++;
swap(&intArray[i], &intArray[j]);
}
}
swap(&intArray[i + 1], &intArray[high]);
return (i + 1);
}
// Method to perform quick sort
void quickSort(int intArray[], int low, int long high){
if (low
int part = partition(intArray, low, high);
quickSort(intArray, low, part - 1);
quickSort(intArray, part + 1, high);
}
}
// Method to swap positions
void swap(int* a, int* b){
int t = *a;
*a = *b;
*b = t;
}
// Method to perform merge sort via merging divided array
void merge(int *a, int low, long int high, long int mid){
long int i, j, k;
long int temp[10000000];
i = low;
k = 0;
j = mid + 1;
while (i
if (a[i]
temp[k] = a[i];
k++;
i++;
}
else{
temp[k] = a[j];
k++;
j++;
}
}
while (i
temp[k] = a[i];
k++;
i++;
}
while (j
temp[k] = a[j];
k++;
j++;
}
for (i = low; i
a[i] = temp[i - low];
}
}
// Mergesort method to divide array
void mergeSort(int *a, int low, long int high){
long int mid;
if (low
mid = (low + high) / 2;
mergeSort(a, low, mid);
mergeSort(a, mid + 1, high);
merge(a, low, high, mid);
}
}
// Method to find max heap
void findMaxHeap(int a[], int i, long int n){
long int j, temp;
temp = a[i];
j = 2 * i;
while (j
if (j a[j])
j = j + 1;
if (temp > a[j])
break;
else if (temp
a[j / 2] = a[j];
j = 2 * j;
}
}
a[j / 2] = temp;
return;
}
// Method to perform heap sort
void heapSort(int a[], long int n){
long int i, temp;
for (i = n; i >= 2; i--){
temp = a[i];
a[i] = a[1];
a[1] = temp;
findMaxHeap(a, 1, i - 1);
}
}
// Method to generate max heap
void generateMaxHeap(int a[], long int n){
long int i;
for (i = n / 2; i >= 1; i--)
findMaxHeap(a, i, n);
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
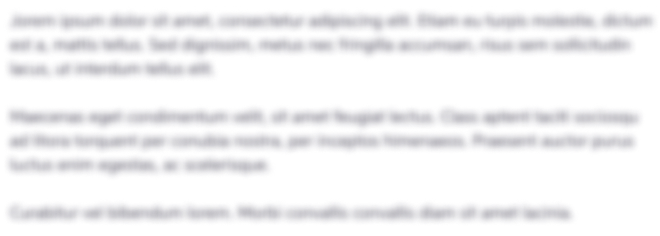
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started