Question
/* Debugging Exercise, based on AT&T Network Bug */ /* See handout associated with this program for more information */ /* Note: this is not
/* Debugging Exercise, based on AT&T Network Bug */ /* See handout associated with this program for more information */ /* Note: this is not intended to be a good example of coding style. */ /* Last edited: 31/8/2011. */
#include
void make_unitialized(void); int phone_network(int i, int x, int y); void init_values_x1(void); void init_values_part1A(void); void init_values_part1B(void); void init_values_part2(void); void init_default(void);
/* global variables that control phone network */ int val1, val2;
/*--------------------------------------------------------- main */ /* main -- a simple driver function that reads three values, which are passed to the function phone_network, which succeeds of fails. Certain combinations of values will call phone_network to fail. */
int main (void) { int i, x, y;
cout << "Enter 3 integers: i, x and y: ";
while ( cin >> i >> x >> y ) {
/* call this to fake that variables are unitialized */ make_unitialized();
if ( phone_network(i, x, y) == 1 ) cout << "Phone network OK! " << endl; else cout << "Phone network crashes! " << endl;
cout << "Enter 3 integers: i, x and y: "; } cout << "You didn't enter 3 valid integers -- program exiting." << endl;
return 0; }
/*------------------------------------------------------route_that_call */ /* returns 1 if system intialized OK, 0 if not */ int route_that_call(void) { if ( val1 >= 0 && val2 >= 0 ) return 1; else return 0; /* Note: you should never write the above logic as an if statement; instead write: return ( val1 >= 0 && val2 >= 0 ); This does the same thing in one line. See why? */
}
/*-------------------------------------------------------phone_network */ /* phone_network -- initialize global control values, then route call. * This function takes 3 values: the first, i, is used to select * which initialization function is called. The 2nd and 3rd * values, x and y, are used to calculate values for the global * control values. (They're also used in calculating initial values * for the control values. * After doing the initialization, function route_that_call is * called to do the "real" work of the phone network system. */ int phone_network(int i, int x, int y) {
const int i1=1, i2=2, val1=3, val2=5;
switch (i) {
case i1: init_values_x1(); break;
case i2: /* for this case, intialization split between several functions */ if (x == val1) { init_values_part1A();
if (y == val2) break; /* drop out of the if and do init_values_part2() */
init_values_part1B(); }
init_values_part2(); break;
default: init_default(); }
/* call function to make the phone call -- it returns 1 for success */ return route_that_call(); }
/*-------------------------------------------------------make_unitialized */ /* For this exercise, we'll pretend negative values mean the system is not initialized properly. */ void make_unitialized(void) { val1 = val2 = -999999; }
/*---------------------------------------------------------init_values_x1 */ void init_values_x1(void) { val1 = 3; val2 = 5; }
/*-----------------------------------------------------init_values_part1A */ void init_values_part1A(void) { val1 = 1; }
/*-------------------------------------------------------init_values_part1B */ void init_values_part1B(void) { ++val1; }
/*-------------------------------------------------------init_values_part2 */ void init_values_part2(void) { val1 = 6; val2 = 7; }
/*-------------------------------------------------------init_default */ void init_default(void) { val1 = 1; val2 = 1; }
_______________________________________________________________________________________________________
LAB: Advanced MSVS Debugger example using a real-life network failure Some of the text and code used in this exercise was borrowed Deep C Programming: Deep C Secrets, by Peter Van Der Linden (SunSoft Press, Prentice Hall, 1984), pp. 38-39. The program that will be used in this exercise is called "att_bug.cpp", which is an included document for the experiments of this lab. The code is based on a program that caused a major disruption of AT&T phone service throughout the U.S. This failure made national news at the time and is one of the best-known software failures. AT&T's nationwide network was mostly unusable for about nine hours starting on the afternoon of January 15, 1990. Telephone exchanges, also known as "switching systems", are computer systems that allow multiple phone calls to be transferred concurrently over one wire. This type of software was running on the hardware controlling the AT&T network. The failure of the network was due to the bug that will be illustrated in this example; The bug led to the first major network problem in AT&T's 114-year history. The bug caused a problem that led to a chain-reaction that spread across the network, bringing down AT&T's entire long distance network. More information on the bug can be found in the January 22, 1990 issue of Telephony magazine. You should be able to understand the logic of the code (att_bug.cpp). Certain values are used to call various functions which initialize certain variables to make the system execute correctly. Different initialization functions are called depending on the values of the initialized variables. A switch statement, if statements, and breakstatements are used to implement the correct initialization logic. In this simplified example, all you need to know is that both global integer variables, val1 and val2, in the program must be set to non-negative values before the function route_that_call() is called.
Testing Section: To use the code in this exercise, simply compile and execute it, and enter 3 integer values. The program will print a message stating whether "the phone system" is OK or crashes for the 3 integer values you entered. The program will repeatedly ask for 3 more values until it gets to the end of file or invalid inputs. Step 1: Compile and execute the program, att-bug.cpp, with various inputs and see what happens. Step 2: Study the logic of the code. Answer the following questions: Question 1: For what values does the function init_values_x1() get invoked? Question 2: For what values does init_values_part1A() get called? Question 3: For what values does init_values_part1B() get called? Question 4: For what values does init_values_part2() get called? Question 5: For what values does init_default()get called? Step 3: Find values for i, x and y that make the phone network crash? Hint: Try each set of data values you identified in Step 2 to make the program perform each of the possible initializations. Together these sets of values are good test data, since each one causes the program to behavior differently. They cover all the behavior caused by the different initialization values. Question 6: What set of 3 values (i,x,y) caused the phone network to crash?
Debugger Section: Now that you know what data is causing the crash, you may still not know why those data values cause the problem. In the steps that follow you will use the MSVS 2010 debugger and answer the following questions. Build the program with the Build Solution option and then carry out the following steps. Step 4: Put a breakpoint at the start of the function, phone_network(). Execute the program, by choosing "Debug" and then "Start Debugging".
Question 7: After entering the 3 values, what is displayed when the execution of the program stops at the breakpoint you set at the line that contain the name of the function? Step 5: Type "Ctrl-F10" to resume execution. Question 8: Does the program stop at the breakpoint again?
Question 9: How do you remove the breakpoint you set? Step 6: If you removed the breakpoint, put it back. Execute the program again. When you reach the breakpoint, choose "F11". This executes the program one line at a time. Execute "F11" a few times to see how "F11" works. Type "Ctrl-F10" to continue on until the breakpoint is reached again. Now that you are back at the initial breakpoint again, do the same thing but this time choose "F10" instead of "F11" to go to the next line. Do this a few times to observe what happens. Question 10: What differences in the execution of the MSVS commands, "F11" and "F10", did you observe? Step 7: Using the same breakpoint from Step 6, run the "F11" command to step "into" a function. Now enter "Shift-F11". The "Shift-F11" command terminates the execution of the function and pauses.
Question 11: Where does execution stop when you enter "Shift-F11"? Step 8: Using the same breakpoint, stop the execution process by choosing "Stop Debugging" and then restart the execution process by choosing "Start Debugging". Now, in the "Watch" window underneath the code window, enter the variables "val1" and "val2" per each row under Name. Once you've done this, run the "F10"command a few times by typing "F10" repeatedly. Then type "Ctrl-F10" to run the "continue" command. Question 12: What does MSVS do with "Watch" variables? Step 9: OK, about that bug.... Set a breakpoint at the line that contains phone_network() or at the switch statement. Enter the 3 data values for i, x, and ythat caused the phone network to crash. From the breakpoint, use the "F10" command to step through the program. Question 13: Did you observe anything that surprises you about the behavior of the program? Was the execution of any lines skipped that you thought should have been executed?
Question 14: Can you explain the behavior you observed?
Step by Step Solution
There are 3 Steps involved in it
Step: 1
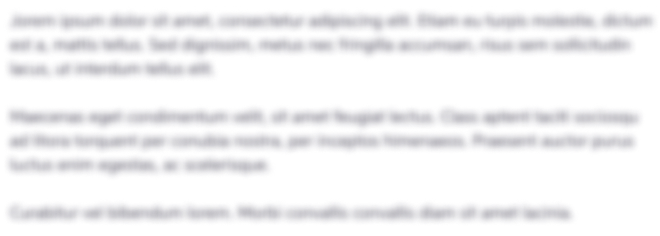
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started