Question
Declare and assign these variables as shown (all your code must appear in the body of the main method): String firstName = Blueberry; String lastName
- Declare and assign these variables as shown (all your code must appear in the body of the main method):
String firstName = "Blueberry";
String lastName = "Strawberry";
In addition, declare and assign to a String reference variable middleName Apple-Sauce.
- In the main() method, make a method call statement to call a method named display () which prints (i.e., displays) to the console, using print( ) and println( ) methods. These System.out.print( ) and System.out.println() are method call statements, which pass the string values as arguments to the methods print(... ) and println(... ), that are provided in an object out of the class System. These arguments are also called actual parameters. (Use + as a concatenation operator. Use an escape sequence for advancing the cursor to the next line for subsequent printing. A space is a character, and therefore, " " is referred to a string containing a space).
The method nameDisplay() must contain the following tasks. This method must be appeared between the "}//end of main()" and "}//end of PracticeLab". Then execute the program and observe their displayed results.
System.out.print("Blueberry");
System.out.print("Strawberry");
System.out.print("Blueberry ");
System.out.print("Strawberry");
System.out.print("Blueberry" + " ");
System.out.print("Strawberry");
System.out.println("Blueberry" + "Strawberry);
System.out.println("Blueberry Strawberry");
System.out.println("Blueberry" + " " + "Strawberry");
System.out.println("Blueberry " + "Apple-Sauce" + " " + "Strawberry");
The program code is as follows:
public class PracticeLab
{
public static void main(String [] args)
{
//declare variables
String firstName = "Blueberry";
String lastName = "Strawberry";
//declare here the middleName to be Apple-Sauce.
Write a method
nameDisplay(); //method call statement, without passing a value or a variable
//as an argument. The argument is also called the actual parameter.
...
}//end of main
public static void nameDisplay()
{
System.out.print("Blueberry");
System.out.print("Strawberry");
System.out.print("Blueberry ");
System.out.print("Strawberry");
System.out.print("Blueberry" + " ");
System.out.print("Strawberry");
System.out.println("Blueberry" + "Strawberry);
System.out.println("Blueberry Strawberry");
System.out.println("Blueberry" + " " + "Strawberry");
System.out.println("Blueberry " + "Apple-Sauce" + " " + "Strawberry");
}//end of nameDisplay( )
}//end of PracticeLab
- In the main(), make another method call statement
nameDisplay(firstName, middleName, lastName);
to call a method
nameDisplay(String fName, String mName, String lastName)
which prints (or displays) to the console using print(), println() and printf() methods. This method call statement passes the string values of three variables as actual arguments (also called actual parameters) to the method nameDisplay(String fName, String mName, String lastName), which has three formal parameters (also called formal arguments). When passing the arguments to the method with parameters, the argument's data type must be compatible with the parameter's data type. Java will automatically perform a widening conversion if the argument's data type is ranked lower than the parameter variable's data type.
make the method with three formal parameters fName, mName, and lastName:
nameDisplay(String fName, String mName, String lastName)
which will carry out the following tasks: (Use %s as a string format.)
//observe the outputs from the print, println, and printf methods.
System.out.println( ); //leave a line space.
System.out.print(fName);
System.out.print(mName);
System.out.print(" " + lastName + "");
System.out.print(fName + " " + mName + " " + lastName + "");
System.out.println(fName + mName + lastName);
System.out.println(lastName + ", " + fName + " " + mName);
System.out.printf("My full name is %s %s %s.", fName, mName, lastName);
System.out.printf("My name is %s, %s %s.", lastName, fName, mName):
Execute the program and then observe the displayed results. Errors of improper spacing between first name, middle name, and last name. Correct these errors according to the following:
Add to the second print statement such that a space is inserted in the output between the first and the middle name.
Add to the sixth print statement to print (display) to the console the first name, middle name, and then last name with proper space in between.
Add to the ninth print statement to print (display) to the console
My name is "Strawberry, Blueberry Apple-Sauce."
- In the main(), make another method call statement
System.out.println("Check whether the name is Blueberry Asian-Pear Strawberry?");
nameDisplay(firstName, "Asian-Pear", lastName);
to call the method
public static void nameDisplay(String fName, String mName, String lastName){ }
which prints (i.e., displays) to the console. Execute it and observe the displayed results.
- In the main(), make another method call statement
nameDisplay(lastName);
to call the method
public static void nameDisplay(String fName){ ... }
Add to the called method the following declaration statement in its declaration block:
String mName = "W.M.";
and the following println() statement.
System.out.println(fName + " " + mName + " " + "Appleseed" + "!");
which displays to the console:
Strawberry W.M. Appleseed!
- In the main method, make a method call statement,
divideDisplay();
Before the method call statement, write a print statement to yield a line space on the console. The called method is
public static void divideDisplay() { ... }
which prints the following expressions to the console in a single line with a tab (\t) between them.
1/2 11/3 4/5 1/2. 11/3. 4./5
See Figure 1 showing the output.
(leave a line space here)
0 3 0 0.5 3.6666666666666665 0.8
Figure 1
Then use the print() method
System.out.print();
to make a comment block that describes your experience with the above printing. As well as using the string concatenation operator +, use both and \t escape sequences to advance the cursor to the next line for subsequent printing and to cause the cursor to skip over the next tab stop, respectively.
(Hints for stating your comment block: What are types of division? Why some of them are zero? Why some of the results are of floating points? What is the escape sequence character for a tab? Use the + operator to display multiple items in your print statement; It is known as the string concatenation operator.)
The output is:
My experience:
The first three are integer divisions, since both operands of
divisions are integers. Thus, the fractional part of the result
is discarded.
For the last three, each of the division operations returns a
floating-point value of double data type, since 2., 3., and 4.
are floating-point numbers of double data type. Furthermore,
before the division operator takes place, the compiler converts
1, 11, and 5 into floating-point values of double data type.
Such a conversion is called a widening conversion.
The + here is a concatenation operation. The symbol \t is an escape sequence,
whichuses the cursor to skip over to the next tab stop.
- Declare and assign the following variables in the variable declaration block of the main() method: (Require to enter the following declaration of variables with assigned values right after the declaration of the three variables of problem 1.)
int iEleven = 11;
int iThree = 3;
double dEleven = 11;
double dThree = 3;
- In the main method, make a method call statement,
divideDisplay(iEleven, dEleven, iThree, dThree);
the called method
public static void divideDisplay(int i11, double d11, int i3, double d3) { }
make ONE print statement that prints/displays to the console the values of the following expressions in three single lines with a tab (or three spaces) between them. The first line contains the values of the first five expressions, the second line contains the values of the second four expressions, and the third line contains the values of the last four expressions.
iEleven/iThree iThree/iEleven dThree/iEleven dEleven/iThree iThree/dEleven
iEleven/dThree dEleven/dThree iEleven/(double)iThree (double)iEleven/iThree (double)(iEleven/iThree) (double) (iThree/iEleven) (int)(dEleven/dThree) (int)(dThree/dEleven)
Note that (double) and (int) are cast operators.
The console displays the result which is as follows:
Solution for problem 8
3 0 0.2727272727272727 3.6666666666666665 0.2727272727272727
3.6666666666666665 3.6666666666666665 3.6666666666666665 3.6666666666666665
3.0 0.0 3 0
- Using again the same variables as above and the same divideDisplay method of problem 8,
make ONE print statement to display to the console the values of the following expressions, each in a new line:
(int)iEleven/dThree (int)(iEleven/dThree) (int)(iEleven/dThree *1000)/1000.
The console displays the result which is as follows:
Solution for problem 9
(int)iEleven/dThree = 3.6666666666666665
(int)(iEleven/dThree) = 3
(int)(iEleven/dThree *1000)/1000. = 3.666
- In the main method, make a method call statement,
squareCubeRoot(7);
and the called method
public static void squareCubeRoot(int num){ }
Write two statements to calculate and print/display the output of the expression, the square root of 7 to the console; and then, to calculate and print/display the output of the expression, the cubic root of 7 to the console:
Math.sqrt(7) Math.cbrt(7)
For your output, it has to be in the following format, as shown in Figure 2:
sqrt(7) = 2.6457513110645907
cbrt(7) = 1.9129311827723892
Figure 2
An example is:
System.out.println("sqrt(" + num + ") = " + Math.sqrt(num));
- In the same called method
private static void squareCubeRoot(int num){ }
repeat the printing of the two expressions above such that both numbers are chopped (i.e., truncated by the program) to two digits after the point. See Figure 3 for the output: [Hints: use printf method with format %.2f for the floating-point value, or format %d for the integer value.]
sqrt(7) = 2.65
cbrt(7) = 1.91
Figure 3
Again, print the two expressions Math.sqrt(7) and Math.cbrt(7) to the console to four digits after the point.
An example is:
System.out.printf("sqrt(%d) = %.2f.", num, Math.sqrt(num));
- Back to the main method, continue to declare and assign the variables as shown: (Require to enter the following variable declarations right after the two variable declarations of problems 1 and 5.)
int iNumber = 8;
int iPrime = 5;
- In the main method, write a method call statement
computeDisplay(iEleven, iThree, iNumber, iPrime);
which passes the values of iThree, iEleven, iNumber, and iPrime to the called method
private static void computeDisplay(int ill, int i13, int iNumber, int iPrime){ }
which compute the following arithmetic expressions, and then print/display their values to the console. Compare the output to your hand calculations:
iEleven / 2 * 2
iThree * iNumber + iEleven / iPrime
iThree / iEleven - iNumber + iThree * iPrime
For displaying your output to the console, it has to be in the following format as shown in Figure 4.
iEleven / 2 * 2 = 10
iThree * iNumber + iEleven / iPrime = ...
iThree / iEleven - iNumber + iThree * iPrime = ...
Figure 4.
- Using the called method in problem 11, continue to compute the following expressions:
iEleven / (2 * 2)
iThree * (iNumber + iEleven) / iPrime
iThree / (iEleven - iNumber) + iThree * iPrime
iThree / (iEleven - iNumber + iThree) * iPrime
iThree / iEleven - (iNumber + iThree) * iPrime
For displaying your output to the console, it has to be in the following format as shown in Figure 5.
Use the printf() method to get the tasks done.
iEleven / (2 * 2) = ...
iThree * (iNumber + iEleven) / iPrime = ...
iThree / (iEleven - iNumber) + iThree * iPrime = ...
iThree / (iEleven - iNumber + iThree) * iPrime = ...
iThree / iEleven - (iNumber + iThree) * iPrime = ...
Figure 5.
- For each of the called methods, make a documentation comment by entering /** and ending with */ before the method header of the called method. The documentation comments can be read and processed by the program Javadoc, which comes with the JDK. You describe each parameter by using a @param tag. For example, you can provide the parameter fName with a description that it is a reference variable of the String class and is referred to as the first name.
/**
* @param fName String class, first name
* @param mName String class, middle name
* @param lastName String class
*/
6 7 9 10 1 12 13 4 15 ) 11 14 16 17 18 19 20 21 22 23 24 25 26 27 280 29 30 31 32 33 34 35 36 37 38 39 40 11 public class Practicelab { public static void main(String[] args) { } // TODO Auto-generated method stub //declare variables } String firstName = "Blueberry"; String lastName = "Strawberry"; String middleName="Apple-Sauce"; int iEleven 11; double dEleven = 11; = //method nameDisplay(); name Display (firstName, middleName, lastName); name Display (firstName, "Asian-Pear", lastName); //method public static void name Display (String fName, String mName, String lastName) { //observe the outputs from the print, println, and printf methods. System.out.println( ); //leave a line space. System.out.print (fName); System.out.print (mName); System.out.print(" " + lastName + " "); System.out.print (fName + "" + mName + "" + lastName + " "); System.out.println (fName + mName + lastName); System.out.println (lastName + "," + fName + " + mName); System.out.printf("My full name is %s %s %s. ", fName, mName, lastName); System.out. printf ("My name is %s, %s %s. ", lastName, fName, mName);
Step by Step Solution
3.51 Rating (151 Votes )
There are 3 Steps involved in it
Step: 1
Heres the modified code that includes the method call to nameDisplay and the im...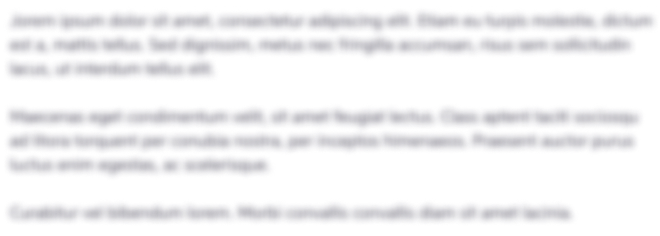
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started