Question
def count_coastline(map): Given a map, how many spots are adjacent to water? Assume: map is a map as defined above, and r and c are
def count_coastline(map): Given a map, how many spots are adjacent to water? Assume: map is a map as defined above, and r and c are int values.
count_coastline(map1) 9
count_coastline(map2) 11
count_coastline([[1,1,1],[1,0,1],[1,1,1]]) 8
def on_ridge(map, r, c): Given a map and a location, is that spot on a ridge? We define a ridge as a place that has two neighbors on opposite side that are both land and both lower than the spot itself. We consider sideto-side, above-and-below, as well as opposing diagonal neighbors as ways to show we are on a ridge. When the adjacent spot is off the map, it can't be used to claim we're on a ridge. If (r,c) isn't on the map, the answer is False. Assume: map is a map as defined above, and r and c are int values.
on_ridge(map1,2,2) True
on_ridge(map6,1,3) False
on_ridge(map6,1,4) True
def is_peak(map, r, c): Given a map and a location, is it land and are all neighboring land spots lower than it? If the spot isn't on the map, it is not a peak. It's possible to have peaks on the border. Assume: map is a map as defined above, and r and c are int values.
is_peak(map2,1,3) True
is_peak(map2,2,3) False
is_peak(map5,3,3) True
def join_map_side(map1, map2): Given two maps, create and return a new map by deep-copying map1 and map2 side by side for a longer map of the same height. If their heights don't match, return None. Assume: map1 and map2 are maps as defined above. Remember, you must not modify the original maps.
join_map_side([[1,2],[5,6]], [[3,4],[7,8]]) [[1,2,3,4],[5,6,7,8]]
join_map_side([[1],[2],[3],[4]], [[5],[6],[7],[8]]) [[1,5],[2,6],[3,7],[4,8]]
join_map_side(m1,m2) None
def join_map_below(map1, map2): Given two maps, create and return a new map by deep-copying map1 and map2 one above the other for a taller map of the same width. If their widths don't match, return None. Assume: map1 and map2 are maps as defined above. Remember, you must not modify the original maps.
join_map_below([[1,1],[2,2]], [[3,3],[4,4]]) [[1,1],[2,2],[3,3],[4,4]]
join_map_below([[1]], [[2]]) [[1],[2]]
join_map_below([[1,2],[3,4]], [[5,6,7,8]]) None
def crop(map, r1, c1, r2, c2): Given a map and two locations, create and return the map that only contains rows from r1 to r2 (inclusive), and columns from c1 to c2 (inclusive). If r1>r2 or c1>c2, the answer has no rows/columns (we return []). Similar to how slicing works, if more rows or columns are requested than exist, just return the parts that do exist. Remember, you must not modify the original map. Assume: map is a map as defined above, and r1, r2, c1, and c2 are int values.
crop(map1,1,1,3,3) [[1,1,1],[1,3,1],[1,3,1]]
crop(map5,3,1,3,4) [[3,5,12,4]]
crop(map2,1,1,90,90) [[0,0,10,0,0,1],[0,1,3,1,1,1]]
crop(m1,2,2,1,3) []
def flooded_map(map, rise): Given a map and a non-negative amount of water rise as an int, create and return a new map where the sea level is that much higher. Each land spot is effectively lowered by rise to keep zero representing sea level; any land that goes underwater ends up as a zero to represent water, regardless of whether it was adjacent to water or not. Assume: map is a map as defined above, and rise is a non-negative int. Restriction: You must not modify the original map in this function.
flooded_map(map1,2) [[0,0,0,0,0],[0,0,0,0,0],[0,0,1,0,0],[0,0,1,0,0],[0,0,1,0,0]]
flooded_map(map2,3) [[1,0,0,0,0,0,0],[0,0,0,7,0,0,0],[0,0,0,0,0,0,0]]
flooded_map([[5,5,5],[4,4,4],[3,3,3],[0,0,0]],3) [[2,2,2],[1,1,1],[0,0,0],[0,0,0]]
def flood_map(map, rise): Given a map and a non-negative amount of water rise as an int, modify the given map (returning None), such that the sea level is that much higher. Each land spot is effectively lowered by rise to keep zero representing the (new) sea level; any land that goes underwater ends up as a zero to represent water, regardless of whether it was adjacent to water or not. Assume: map is a map as defined above, and rise is a non-negative int. Restriction: You must modify the original map in this function.
>>> m = [[10,10,4],[9,9,5],[8,8,6]]
>>> flood_map(m,5)
>>> print(show(m)) 5 5 0 4 4 0 3 3 1 >>>
def find_land(map, r, c, dir): Given a map and location, as well as a direction to look (one of ["N","NE","E","SE","S","SW","W","NW"]), indicate how many spaces we must travel on the map to reach land. If we're on land, the answer is zero. If there's no land in that direction on this map, we return None. Assume: map is a map as defined above, r and c are int values, and dir is one of the strings above.
find_land(map4,2,2,"S") 2
find_land(map4,4,1,"NE") None
find_land(map5,7,0,"N") 5
def reorient(map): Given a map, we want to rotate it clockwise one quarter turn. This means that the left column becomes the top row, the top row becomes the right column, and so on. Assume: map is a map as defined above.
reorient([[1,2],[3,4]]) [[3,1],[4,2]]
reorient([[1,2,3],[4,5,6],[7,8,9],[10,11,12]]) [[10, 7, 4, 1], [11, 8, 5, 2], [12, 9, 6, 3]]
reorient(reorient([[1,2],[3,4]]) [[3,4],[2,1]]
Step by Step Solution
There are 3 Steps involved in it
Step: 1
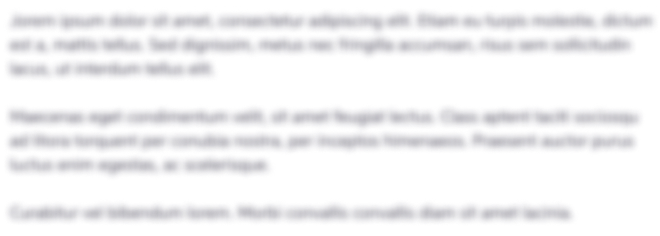
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started