Question
Define a class for set elements. A set class is used to represent sets of integers. It supports most of the standard set operations. The
Define a class for set elements. A set class is used to represent sets of integers. It supports most of the standard set operations. The program will demonstrate overloaded operators, member functions, friend functions and random number generation.
****************************************************************************************
Set data structure:
A set is a collection of objects need not to be in any particular order. Elements
should not be repeated.
UNION: Combine two or more sets (here two sets)
INTERSECTION: Gathering common elements in both the sets together as a single set
DIFFERENCE: Forming a set with elements which are in first set and not in second set
A-B= {x| for all x belongs to A but not in B}
***************************************************************************************
Sample class declaration:
You class should have all of the followings.
Can be defined as friend functions
class Set {
public:
//default constructor
Set();
//add element to set
void addElement (int element);
//remove element from set
void removeElement(int element);
//check for membership
bool isMember(int element);
//set union, modifies curremtn set
void Union (Set s);
//set difference modifiers current set
void difference (Set s);
//size of set
int size();
//get element i
int getElement (int i);
private:
//binary search for element, returns index
bool search(int element, int& index);
//set members
int elements[maxElements];
//next empty position in elements
int next;
};
Overload the following operators the class Set:
A suitable overloading of the quality operator ==
A suitable overloading of the inquality operator !=
A suitable overloading of the add element operator +
A suitable overloading of the set union operator +
A suitable overloading of the remove operator -
A suitable overloading of the set difference operator -
Hints: some regular functions might helpful
void printSet(Set s);
void generateSample(int sample[], int n);
Generate a set of value of a certain size. Produce an array that is used to initialize the sets in our experiment.
void run(int sample[], int n);
Step by Step Solution
There are 3 Steps involved in it
Step: 1
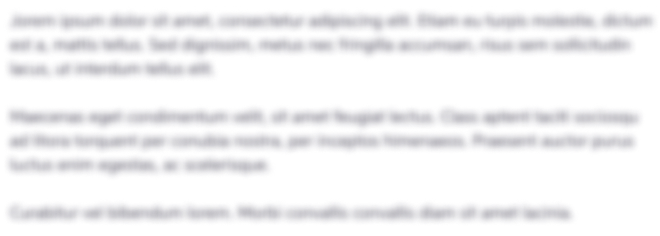
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started