Question
Define a class 'Rectange' in 'Rectangle.java'. The class need a contructor that takes width and height as double type. Adds methods equals(), compareTo(), toString() so
Define a class 'Rectange' in 'Rectangle.java'. The class need a contructor that takes width and height as double type. Adds methods equals(), compareTo(), toString() so that it works as shown in 'MyArrayObjDemo.java'. Ignore the difference by rotation and consider a Rectange(2,5) and a Rectangle(5,2) are equal. Use their areas for comparison.
CODE:
package apps; public class Rectangle implements Comparable{ private double width; private double height; public Rectangle(double width, double height) { this.width = width; this.height = height; } public double getArea() { return width * height; } public double getPerimeter() { return 2 * (width + height); } @Override public boolean equals(Object obj) { if (obj == null || getClass() != obj.getClass()) { return false; } Rectangle other = (Rectangle) obj; return (width == other.width && height == other.height) || (width == other.height && height == other.width); } @Override public int compareTo(Rectangle other) { return Double.compare(getArea(), other.getArea()); } @Override public String toString() { return String.format("Rect(%.1f,%.1f)", width, height); } }
Define a class 'RightTriangle' in 'RightTriangle.java'. The class need a contructor that takes two sides next to the right angle as double type. Adds methods equals(), compareTo(), toString() so that it works as shown in 'MyArrayObjDemo.java'. Ignore the difference by rotation and consider a RightTriangle(3,4) and a RightTriangle(4,3) are equal Use their perimeters for comparison.
CODE:
package apps; public class RightTriangle implements Comparable{ private double side1; private double side2; public RightTriangle(double side1, double side2) { this.side1 = side1; this.side2 = side2; } public double getArea() { return side1 * side2 / 2; } public double getPerimeter() { return side1 + side2 + Math.sqrt(side1 * side1 + side2 * side2); } public int compareTo(RightTriangle rt) { return Double.compare(getPerimeter(), rt.getPerimeter()); } public String toString() { return "RT(" + side1 + "," + side2 + ")"; } public boolean equals(Object obj) { if (this == obj) return true; if (!(obj instanceof RightTriangle)) return false; RightTriangle rt = (RightTriangle) obj; return (this.side1 == rt.side1 && this.side2 == rt.side2) || (this.side1 == rt.side2 && this.side2 == rt.side1); } }
How do I get them to work with MyArrayObjDemo.java
public class MyArrayObjDemo { public static void main(String[] args) { MyArrayrectangles = new MyArray (); Rectangle r1 = new Rectangle(2, 5); Rectangle r2 = new Rectangle(2, 4.9); System.out.println(r1.getArea() + ", " + r1.getPerimeter()); // 10.0, 14.0 rectangles.add(r1); rectangles.add(r2); rectangles.add(new Rectangle(1,9)); rectangles.printArray(); // printArray(3,5): Rect(2.0,5.0) Rect(2.0,4.9) Rect(1.0,9.0) System.out.println(rectangles.search(r2)); // 1 System.out.println(rectangles.search(new Rectangle(5.0,2.0))); // 0 rectangles.sort(); rectangles.printArray(); // printArray(3,5): Rect(1.0,9.0) Rect(2.0,4.9) Rect(2.0,5.0) MyArray rTriagles = new MyArray (); RightTriangle rt1 = new RightTriangle(3, 4); RightTriangle rt2 = new RightTriangle(1, 1); System.out.println(rt1.getArea() + ", " + rt1.getPerimeter()); // 6.0, 12.0 rTriagles.add(rt1); rTriagles.add(rt2); rTriagles.add(new RightTriangle(3.1, 3.9)); rTriagles.printArray(); // printArray(3,5): RT(3.0,4.0) RT(1.0,1.0) RT(3.1,3.9) System.out.println(rTriagles.search(rt2)); // 1 System.out.println(rTriagles.search(new RightTriangle(4,3))); // 0 rTriagles.sort(); rTriagles.printArray(); // printArray(3,5): RT(1.0,1.0) RT(3.1,3.9) RT(3.0,4.0) } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
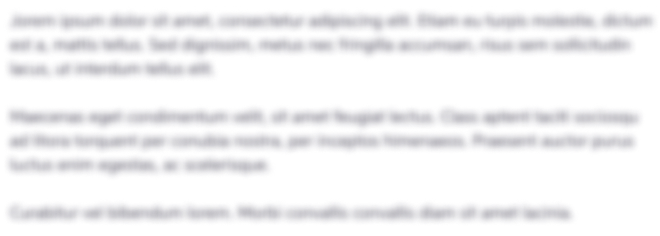
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started