Question
Define a class Shares as an ADT that uses separate compilation (i.e separate interface and implementation files). The class represents shares for a shareholder in
Define a class Shares as an ADT that uses separate compilation (i.e separate interface and implementation files). The class represents shares for a shareholder in a specific company on the JSE. This class has four member variables:
company, a string that holds the name of the company
nrShares, an integer value that indicates the number of shares this shareholder owns
unitValue, a float value that indicates the current value of one share and
pastUnitValues, an array to keep five double values.
In addition, the class has the following member functions:
A default constructor that initializes company to an empty string. nrShares and unitValue should each be initialized to 0. All elements in pastUnitValues should be initialized to 0.
An overloaded constructor that represents shares bought in a new company and sets company, nrShares and unitValue to specified values, while all elements in pastUnitValues are initialized to 0.
A destructor that does not perform any action.
Accessor functions for member variables nrShares and unitValues.
An overloaded equality operator== to compare two Shares objects. The == operator is implemented as a friend function with the following prototype:
bool operator==(const Shares & s1, const Shares & s2)
This function returns true if shares1 and shares2 have the same company name; and false if not.
A member function updateShares() that adds the number of shares bought to nrShares; update the value of unitValue and also update pastUnitValues to include the unit value of the current purchase as the last element in array pastUnitValues. Hint: move all elements in pastUnitValues one position to the left to add the current unitValue as the last element. Use the following prototype: void updateShares(const Shares & s1);
An overloaded extraction operator >> (implemented as a friend function) so that it can be used to input values of type Shares.
An overloaded insertion operator << (implemented as a friend function) that outputs all the member variables of a Shares object.
Demonstrate the class in an application program (main()). This program obtains the detail for a new purchase of shares from the user and creates an object to represent the new purchase of shares. It then extracts all existing shares in the user’s portfolio one by one from a file Portfolio.dat and checks whether the shareholder already has shares in the company. If the user already holds shares in the company, the member function updateShares() is used to update the shares in the company to include the new shares purchased. All shares (updated as well as unchanged existing shares) are output to a new portfolio file called NewPortfolio.dat. If the user does not already own shares in the company, the new purchase of shares are simply output to NewPortfolio.dat. Before outputting a shareholding to NewPortfolio.dat, the program calculates the value of the shareholding (number of shares * unit value per share) and adds it to a running total. The running total is used to calculate the total value of the shares, which is displayed on the console window at the end of the program. Be sure to use appropriate member functions in the required statements. See below for sample input and corresponding output. Submit copies of the input and output files and the console window, as well as all the files in the ADT.
Portfolio.dat
OldMutual 10 12.5 0 0 0 0 12.5
Sanlam 15 10.5 12.43 15.3 11.4 9.4 10.5
Allied 5 6.5 0 0 8.45 7.98 6.5
Output:
Enter name of company in which shares are bought: OldMutual
Enter number of shares bought: 10
Enter unit value of one share: 5
Total value of all shares: R 290.00
Process returned 0 (0x0) execution time : 11.030 s
Press any key to continue.
NewPortfolio.dat
OldMutual 20 5 0 0 0 12.5 5
Sanlam 15 10.5 12.43 15.3 11.4 9.4 10.5
Allied 5 6.5 0 0 8.45 7.98 6.5
Step by Step Solution
3.44 Rating (154 Votes )
There are 3 Steps involved in it
Step: 1
include include include include using namespace std define MAX 5 Defines a class Shares ...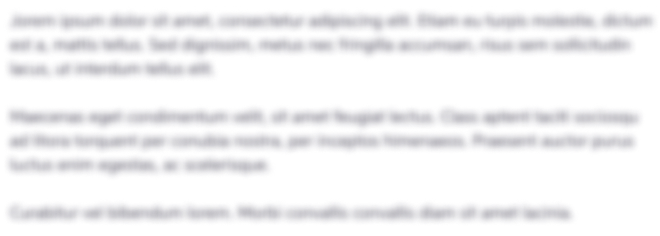
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started