Question
Define a class to model an abstract circle. A circle is defined by the value of its radius. Your class should have one instance variable
Define a class to model an abstract circle. A circle is defined by the value of its radius. Your class should have one instance variable of type double. The name of the class is Circle. In addition to writing your own default, parameterless constructor, include a constructor with a single argument that is used to set the instance variable of an object of the Circle class. Note that the radius of a circle must be greater than zero and your class should uphold this restriction. Define accessor (getter) and mutator (setter) methods. In addition, define the following accessor methods: public double getDiameter() public double getCircumference() public double getArea() public String toString() Here are the formulas you'll need: Diameter = 2r Circumference = 2r Area of a circle = r2 where r is the radius. Use the constant from the Math class of the Java API as the value of . The toString() method is used to format the instance variable as a String. It is public, has no parameters, and returns a String. It is automatically called when used as the argument in a System.out.println() statement. For example, Circle myCircle = new Circle(2.5); System.out.println(myCircle); // implicitly calls toString() The statement above displays the memory location of the object when there is no toString()method written for the class. If toString() is implemented in the class, the same statement would print out whatever String returned by the toString() method. As discussed in class, the toString() method of the Circle class should return labeled information about the internal state of the instance of the class. Download the CircleDriver.java program containing a main method that thoroughly tests all of the methods in the Circle class. Verify that this test program works with your Circle class without making any changes to the CircleDriver source code. Develop this program in stages: 1. Write the default and parameterized constructors 2. Write the toString()to display the object 3. Write getter and setter methods, then display results after setting a new radius value 4. Repeat step 3 for the remaining project requirements This class file name should be Circle.java. Following the general guidelines stated above for the Circle class, write a class to model an abstract Rectangle. A rectangle is defined by the value of its two sides. Your class should have two instance variables of type double. The name of the class is Rectangle. In addition to writing your own default, parameterless constructor, include a constructor with two arguments that is used to set the instance variables of an object of the Rectangle class. Note that the sides of a rectangle must be greater than zero and your class should uphold this restriction. Define accessor (getter) and mutator (setter) methods for each instance variable. In addition, define the following accessor methods: public double getPerimeter() public double getArea() public boolean isSquare() public String toString() This file name should be Rectangle.java. OPTIONAL: WRITE A PROGRAM CALLED RECTANGLEDRIVER.JAVA THAT CONTAINS A MAIN METHOD TO THOROUGHLY TEST ALL OF THE METHODS IN YOUR RECTANGLE CLASS. FEEL FREE TO MODEL THIS TEST CLASS AFTER CIRCLEDRIVER.JAVA. Make sure your programs are well documented - including a comment block header in all source code files with your name, the course and section numbers, the project name, the program purpose. Be sure to include appropriate comments throughout your code, choose meaningful identifiers, and use
Step by Step Solution
There are 3 Steps involved in it
Step: 1
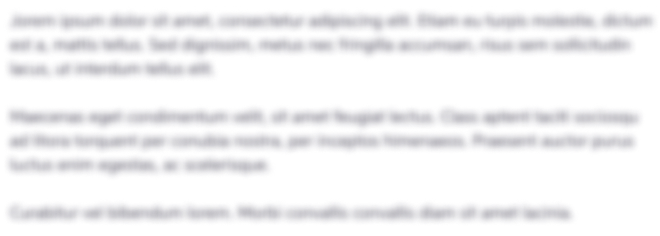
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started