Question
define a function void printArray(char ** records, int n) which takes as argument an array of char pointers, and prints first n pointees of records
define a function void printArray(char ** records, int n) which takes as argument an array of char pointers, and prints first n pointees of records on stdout, one line for each pointee of the array. Use pointer notation only, dont use array index notation. Note that the argument is declared as a pointer to pointer char **, which is what an array of char pointer char * record [] is decayed to when it is passed to a function (why?) define a function void exchange(char * records[])which takes as argument an array of char pointers, and swaps the pointee of the third element pointer (element 2) with the fourth element pointer, and swap the pointee of the fifth element with the 6th pointer. call printArray in main to display the original array. then in main, exchange pointees of the first (element 0) and the 2 nd (element 1) pointers of the array, and then send the array to function exchange() to exchange some other pointees. finally, call function printArray() again to display the (reordered) pointees of the pointer arrays.
Starter code:
#include#include main(){ char * inputs[7] = {"this is input 0, orange is orange", "this is input 1, apple is green", "this is input 2, cherry is red", "this is input 3, banana is yellow"}; char arr1 [] = "this is input 4, do you like them?"; char arr2 [] = "this is input 5, yes bye"; char arr3 [] = "this is input 6, bye"; inputs[4] = arr1; inputs[5] = arr2; inputs[6] = arr3; // display the array by calling printArr // swap pointee of first and second // call exchange() to swap some other 'rows'; printf("=========================== "); // display the array again }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
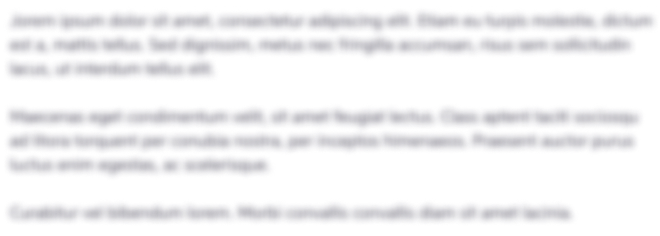
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started