Question
Define the class addressBookType.h and addressBookTypeImp.cpp using these defined classes. A main.cpp file should be created as well. Using classes, design an online address book
Define the class addressBookType.h and addressBookTypeImp.cpp using these defined classes. A main.cpp file should be created as well.
Using classes, design an online address book to keep track of the names, addresses, phone numbers, and dates of birth of family members, close friends, and certain business associates. Use the included personType.h, personTypeImp.cpp, dateType.h, dateTypeImp.cpp, addressType.h, addressTypeImp.cpp, extPersonType.h, and extPersonTypeImp.cpp files to complete the assignment.
USE C++
This is one question, please read the instructions and do not copy the other answers to this question that were already submitted, they are incorrect and or missing the rest of the program.
The program should perform the following operations:
- Provide the user a menu option with the ability to enter the personal information, the date of birth information and the address information. You will need to create an array of 10 addressBookType objects and write data to an object to the array as the user data in entered. The user should have the ability to use this menu option in the program to enter another object. You will need to keep track of the number of objects entered to make sure the user cannot enter more than 10 objects.
- Provide a menu option to search by name and print the address, phone number and date of birth if the name exists. Give an appropriate message if the name is not found.
- Provide a menu option to enter a month of the year and display the name, address, and phone number of the entries with birthdays in that month.
- Provide a menu option to enter the classification of a person (family, friend, or business) and display the name, address, and phone number of the entries with birthdays in that month.
- Provide a menu option to exit the program.
- Make sure the user does not exceed the 10-max limit of addressBookType objects at any point during the program.
BELOW ARE THE INCLUDED FILES REQUIRED TO COMPLETE THIS ASSIGNMENT
+++++++++++++++++++++++++ personType.h file +++++++++++++++++++++++++
//personType.h #ifndef H_personType #define H_personType #include
class personType { public: void print() const; void setName(string first, string last);
string getFirstName() const;
string getLastName() const;
personType(string first = "", string last = "");
private: string firstName; string lastName; };
#endif
+++++++++++++++++++++++++ personTypeImp.cpp file +++++++++++++++++++++++++
//personTypeImp.cpp
#include
void personType::print() const { cout << firstName << " " << lastName; }
void personType::setName(string first, string last) { firstName = first; lastName = last; }
string personType::getFirstName() const { return firstName; }
string personType::getLastName() const { return lastName; }
//constructor personType::personType(string first, string last) { firstName = first; lastName = last; }
+++++++++++++++++++++++++ dateType.h file +++++++++++++++++++++++++
#ifndef dateType_H #define dateType_H class dateType { public: void setDate(int month, int day, int year);
int getDay() const;
int getMonth() const;
int getYear() const;
void printDate() const;
dateType(int month = 1, int day = 1, int year = 1900);
private: int dMonth; int dDay; int dYear; };
#endif
+++++++++++++++++++++++++ dateTypeImp.cpp file +++++++++++++++++++++++++
//Implementation file date #include
using namespace std;
void dateType::setDate(int month, int day, int year) { dMonth = month; dDay = day; dYear = year; }
int dateType::getDay() const { return dDay; }
int dateType::getMonth() const { return dMonth; }
int dateType::getYear() const { return dYear; }
void dateType::printDate() const { cout << dMonth << "-" << dDay << "-" << dYear; }
//Constructor with parameters dateType::dateType(int month, int day, int year) { dMonth = month; dDay = day; dYear = year; }
+++++++++++++++++++++++++ addressType.h file +++++++++++++++++++++++++
// addressType.h
#ifndef H_addressType #define H_addressType
#include
using namespace std;
class addressType { public: void setStreet(string myStreet); void setCity(string myCity); void setState(string myState); void setZip(int myZip);
string getStreet() const; string getCity() const; string getState() const; int getZip() const;
void printAddress() const; addressType(string = "", string = "", string = "", int = 0);
private: string street; string city; string state; int zip; }; #endif
+++++++++++++++++++++++++ addressTypeImp.cpp file +++++++++++++++++++++++++
//AddressTypeImp.cpp
#include
using namespace std;
//setStreet function void addressType::setStreet(string myStreet) { street = myStreet; } //setCity function void addressType::setCity(string myCity) { city = myCity; } //setState function void addressType::setState(string myState) { state = myState; } //setZip function void addressType::setZip(int myZip) { zip = myZip; }
//getStreet function string addressType::getStreet() const { return street; } //getCity function string addressType::getCity() const { return city; } //getState function string addressType::getState() const { return state; } //getZip function int addressType::getZip() const { return zip; }
//print function void addressType::printAddress() const { cout << " \t" << getStreet() << ", " << getCity() << ", " << getState() << " - " << getZip(); }
//addressType constructor addressType::addressType(string myStreet, string myCity, string myState, int myZip) { setStreet(myStreet); setCity(myCity); setState(myState); setZip(myZip); }
+++++++++++++++++++++++++ extPersonType.h file +++++++++++++++++++++++++
//extPersonType.h file
#ifndef H_extPersonType #define H_extPersonType
#include "addressType.h" #include "personType.h" #include "dateType.h"
#include
using namespace std;
class extPersonType { public: void print() const; void setBirthDate(const dateType); void getBirthDate(dateType&) const; void setAddress(const addressType address); void getAddress(addressType& address) const; void setPerson(const string, const string); void getPerson(string&, string&) const; void setAssociation(const string); string getAssociation() const; void setPhone(const string); string getPhone() const;
void setExtPerson(string, string, int, int, int, string, string, string, int, string, string); extPersonType(string = "", string = "", int = 1, int = 1, int = 1900, string = "", string = "", string = "", int = 0, string = "", string = "");
private: personType person; dateType birthDate; addressType myAddress; string association; string phone; };
#endif
+++++++++++++++++++++++++ extPersonTypeImp.cpp file +++++++++++++++++++++++++
//extPersonTypeImp.cpp
#include "extPersonType.h"
#include
using namespace std;
//setBirthDate function void extPersonType::setBirthDate(const dateType myDate) { birthDate.setDate(myDate.getMonth(), myDate.getDay(), myDate.getYear()); } //getBirthDate function void extPersonType::getBirthDate(dateType& myDate) const { myDate.setDate(birthDate.getMonth(), birthDate.getDay(), birthDate.getYear()); }
//setAddress function void extPersonType::setAddress(const addressType address) { myAddress.setStreet(address.getStreet()); myAddress.setCity(address.getCity()); myAddress.setState(address.getState()); myAddress.setZip(address.getZip()); } //getAddress function void extPersonType::getAddress(addressType& address) const { address.setStreet(myAddress.getStreet()); address.setCity(myAddress.getCity()); address.setState(myAddress.getState()); address.setZip(myAddress.getZip()); }
//print function void extPersonType::print() const { birthDate.printDate(); person.print(); myAddress.printAddress();
cout << " \t" << association << ", " << phone; }
//setPerson first, last name function void extPersonType::setPerson(const string first, const string last) { person.setName(first, last); } //getPerson first, last name function void extPersonType::getPerson(string& first, string& last) const { first = person.getFirstName(); last = person.getLastName(); }
//setAssociation function void extPersonType::setAssociation(const string myAssociation) { association = myAssociation; } //getAssociation function string extPersonType::getAssociation() const { return association; }
//setPhone function void extPersonType::setPhone(const string myPhone) { phone = myPhone; } //getPhone function string extPersonType::getPhone()const { return phone; }
//setExtPerson function void extPersonType::setExtPerson(string first, string last, int month, int day, int year, string myStreet, string myCity, string myState, int myZip, string asso, string ph) { person.setName(first, last); birthDate.setDate(month, day, year); myAddress.setStreet(myStreet); myAddress.setCity(myCity); myAddress.setState(myState); myAddress.setZip(myZip); setAssociation(asso); setPhone(ph); }
//extPersonType constructor extPersonType::extPersonType(string first, string last, int month, int day, int year, string myStreet, string myCity, string myState, int myZip, string asso, string ph): person(first, last), birthDate(month, day, year), myAddress(myStreet, myCity, myState, myZip) { setAssociation(asso); setPhone(ph); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
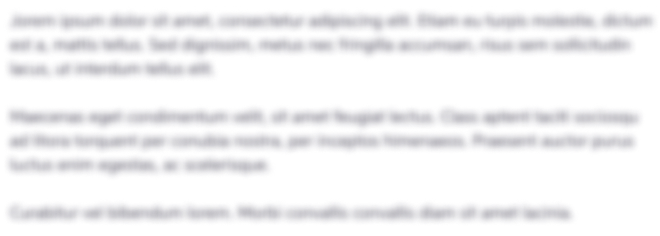
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started