Answered step by step
Verified Expert Solution
Question
1 Approved Answer
//Demonstrates the BankAccount and derived classes import java.text.*; // to use Decimal Format public class AccountDriver { public static void main(String[] args) { double put_in
//Demonstrates the BankAccount and derived classes import java.text.*; // to use Decimal Format public class AccountDriver { public static void main(String[] args) { double put_in = 500; double take_out = 1000; DecimalFormat myFormat; String money; String money_in; String money_out; boolean completed; // to get 2 decimals every time myFormat = new DecimalFormat("#.00"); //to test the Checking Account class CheckingAccount myCheckingAccount = new CheckingAccount ("Benjamin Franklin", 1000); System.out.println ("Account Number " + myCheckingAccount.getAccountNumber() + " belonging to " + myCheckingAccount.getOwner()); money = myFormat.format(myCheckingAccount.getBalance()); System.out.println ("Initial balance = $" + money); myCheckingAccount.deposit (put_in); money_in = myFormat.format(put_in); money = myFormat.format(myCheckingAccount.getBalance()); System.out.println ("After deposit of $" + money_in + ", balance = $" + money); completed = myCheckingAccount.withdraw(take_out); money_out = myFormat.format(take_out); money = myFormat.format(myCheckingAccount.getBalance()); if (completed) { System.out.println ("After withdrawal of $" + money_out + ", balance = $" + money); } else { System.out.println ("Insuffient funds to withdraw $" + money_out + ", balance = $" + money); } System.out.println(); //to test the savings account class SavingsAccount yourAccount = new SavingsAccount ("William Shakespeare", 400); System.out.println ("Account Number " + yourAccount.getAccountNumber() + " belonging to " + yourAccount.getOwner()); money = myFormat.format(yourAccount.getBalance()); System.out.println ("Initial balance = $" + money); yourAccount.deposit (put_in); money_in = myFormat.format(put_in); money = myFormat.format(yourAccount.getBalance()); System.out.println ("After deposit of $" + money_in + ", balance = $" + money); completed = yourAccount.withdraw(take_out); money_out = myFormat.format(take_out); money = myFormat.format(yourAccount.getBalance()); if (completed) { System.out.println ("After withdrawal of $" + money_out + ", balance = $" + money); } else { System.out.println ("Insuffient funds to withdraw $" + money_out + ", balance = $" + money); } yourAccount.postInterest(); money = myFormat.format(yourAccount.getBalance()); System.out.println ("After monthly interest has been posted," + "balance = $" + money); System.out.println(); // to test the copy constructor of the savings account class SavingsAccount secondAccount = new SavingsAccount (yourAccount,5); System.out.println ("Account Number " + secondAccount.getAccountNumber()+ " belonging to " + secondAccount.getOwner()); money = myFormat.format(secondAccount.getBalance()); System.out.println ("Initial balance = $" + money); secondAccount.deposit (put_in); money_in = myFormat.format(put_in); money = myFormat.format(secondAccount.getBalance()); System.out.println ("After deposit of $" + money_in + ", balance = $" + money); secondAccount.withdraw(take_out); money_out = myFormat.format(take_out); money = myFormat.format(secondAccount.getBalance()); if (completed) { System.out.println ("After withdrawal of $" + money_out + ", balance = $" + money); } else { System.out.println ("Insuffient funds to withdraw $" + money_out + ", balance = $" + money); } System.out.println(); //to test to make sure new accounts are numbered correctly CheckingAccount yourCheckingAccount = new CheckingAccount ("Issac Newton", 5000); System.out.println ("Account Number " + yourCheckingAccount.getAccountNumber() + " belonging to " + yourCheckingAccount.getOwner()); } }
/**Defines any type of bank account*/ public abstract class BankAccount { /**class variable so that each account has a unique number*/ protected static int numberOfAccounts = 100001; /**current balance in the account*/ private double balance; /** name on the account*/ private String owner; /** number bank uses to identify account*/ private String accountNumber; /**default constructor*/ public BankAccount() { balance = 0; accountNumber = numberOfAccounts + ""; numberOfAccounts++; } /**standard constructor @param name the owner of the account @param amount the beginning balance*/ public BankAccount(String name, double amount) { owner = name; balance = amount; accountNumber = numberOfAccounts + ""; numberOfAccounts++; } /**copy constructor creates another account for the same owner @param oldAccount the account with information to copy @param the beginning balance of the new account*/ public BankAccount(BankAccount oldAccount, double amount) { owner = oldAccount.owner; balance = amount; accountNumber = oldAccount.accountNumber; } /**allows you to add money to the account @param amount the amount to deposit in the account*/ public void deposit(double amount) { balance = balance + amount; } /**allows you to remove money from the account if enough money is available,returns true if the transaction was completed, returns false if the there was not enough money. @param amount the amount to withdraw from the account @return true if there was sufficient funds to complete the transaction, false otherwise*/ public boolean withdraw(double amount) { boolean completed = true; if (amount Introduction In this lab, you will be creating new classes that are derived from a class called BankAccount. A checking account is a bank account and a savings account is a bank account as well. This sets up a relationship called inheritance, where BankAccount is the superclass and CheckingAccount and SavingsAccount are subclasses. This relationship allows CheckingAccount to inherit attributes from BankAccount (like owner, balance, and accountNumber, but it can have new attributes that are specific to a checking account, like a fee for clearing a check. It also allows CheckingAccount to inherit methods from BankAccount, like deposit, that are universal for all bank accounts. You will write a withdraw method in CheckingAccount that overrides the withdraw method in BankAccount, in order to do something slightly different than the original withdraw method. You will use an instance variable called accountNumber in SavingsAccount to hide the accountNumber variable inherited from BankAccount. The UML diagram for the inheritance relationship is as follows BankAccount balance double owner: String -account Number :String enumberOfAccounts int Bank Account() BankAccount(name :String, amount :double): +BankAccount (oldAccount:BankAccount, amount double): deposit (amount double): void withdraw(amount: double) :boolean +getBalance :double getowner():String +getAccountNumber String setBalance (amount: double): void setAccountNumber(newAccountNumber :String) :void CheckingAccount SavingsAccount FEE double rate double savingsNumber:int Checking Account(name: String, accountNumber :String amount double) withdraw (amount: double) :boolean +SavingsAccount(name:String, amount :double) Savings Account(oldAccount: SavingsAccount, amount double: postInterest ():void getAccountNumber ():String Task #1 Extending Bank Account Introduction In this lab, you will be creating new classes that are derived from a class called BankAccount. A checking account is a bank account and a savings account is a bank account as well. This sets up a relationship called inheritance, where BankAccount is the superclass and CheckingAccount and SavingsAccount are subclasses. This relationship allows CheckingAccount to inherit attributes from BankAccount (like owner, balance, and accountNumber, but it can have new attributes that are specific to a checking account, like a fee for clearing a check. It also allows CheckingAccount to inherit methods from BankAccount, like deposit, that are universal for all bank accounts. You will write a withdraw method in CheckingAccount that overrides the withdraw method in BankAccount, in order to do something slightly different than the original withdraw method. You will use an instance variable called accountNumber in SavingsAccount to hide the accountNumber variable inherited from BankAccount. The UML diagram for the inheritance relationship is as follows BankAccount balance double owner: String -account Number :String enumberOfAccounts int Bank Account() BankAccount(name :String, amount :double): +BankAccount (oldAccount:BankAccount, amount double): deposit (amount double): void withdraw(amount: double) :boolean +getBalance :double getowner():String +getAccountNumber String setBalance (amount: double): void setAccountNumber(newAccountNumber :String) :void CheckingAccount SavingsAccount FEE double rate double savingsNumber:int Checking Account(name: String, accountNumber :String amount double) withdraw (amount: double) :boolean +SavingsAccount(name:String, amount :double) Savings Account(oldAccount: SavingsAccount, amount double: postInterest ():void getAccountNumber ():String Task #1 Extending Bank Account
Step by Step Solution
There are 3 Steps involved in it
Step: 1
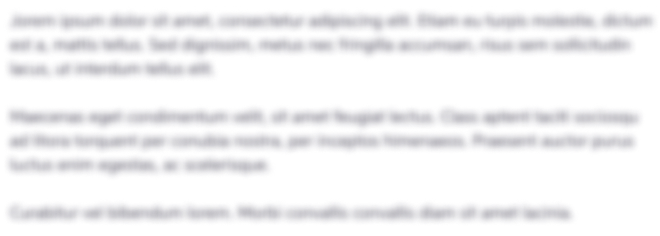
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started