Answered step by step
Verified Expert Solution
Question
1 Approved Answer
--- Demonstrating Julian Dates --- Today's date is : February 4, 2020 Tomorrow will be : February 5, 2020 Yesterday was : February 3, 2020
--- Demonstrating Julian Dates --- Today's date is : February 4, 2020 Tomorrow will be : February 5, 2020 Yesterday was : February 3, 2020 This year is a leap year! Demonstrating: 2/27/2100 Adding 1 day to the date : 2/28/2100 Adding another day : 2/29/2100 Just one more day : 3/1/2100 Going backwards by 2 days : 2/28/2100 Demonstrating: 2/27/2000 Adding 1 day to the date : 2/28/2000 Adding another day : 2/29/2000 Just one more day : 3/1/2000 Going backwards by 2 days : 2/28/2000 Demonstrating: 2/27/2001 Adding 1 day to the date : 2/28/2001 Adding another day : 3/1/2001 Just one more day : 3/2/2001 Going backwards by 2 days : 2/28/2001 Demonstrating: 12/30/1999 Adding 1 day to the date : 12/31/1999 Adding another day : 1/1/2000 Just one more day : 1/2/2000 Going backwards by 2 days : 12/31/1999 --- Demonstrating Gregorian Dates --- Today's date is : February 17, 2020 Tomorrow will be : February 18, 2020 Yesterday was : February 16, 2020 This year is a leap year! Demonstrating: 2/27/2100 Adding 1 day to the date : 2/28/2100 Adding another day : 3/1/2100 Just one more day : 3/2/2100 Going backwards by 2 days : 2/28/2100 Demonstrating: 2/27/2000 Adding 1 day to the date : 2/28/2000 Adding another day : 2/29/2000 Just one more day : 3/1/2000 Going backwards by 2 days : 2/28/2000 Demonstrating: 2/27/2001 Adding 1 day to the date : 2/28/2001 Adding another day : 3/1/2001 Just one more day : 3/2/2001 Going backwards by 2 days : 2/28/2001 Demonstrating: 12/30/1999 Adding 1 day to the date : 12/31/1999 Adding another day : 1/1/2000 Just one more day : 1/2/2000 Going backwards by 2 days : 12/31/1999
You are provided with a skeleton project to work from that has JUnit setup and includes the unit tests for this assignment. The code is:
/** * Assignment 5 for CS 1410 * This program demonstrates the use of the GregorianDate and JulianDate classes * * @author James Dean Mathias */ public class Assign5 { public static void main(String[] args) { System.out.printf("--- Demonstrating Julian Dates --- "); demoJulianFromToday(); JulianDate futureYear = new JulianDate(2100, 2, 27); demoDateJulian2(futureYear); JulianDate leapYear = new JulianDate(2000, 2, 27); demoDateJulian2(leapYear); JulianDate notLeapYear = new JulianDate(2001, 2, 27); demoDateJulian2(notLeapYear); JulianDate endOfYear = new JulianDate(1999, 12, 30); demoDateJulian2(endOfYear); System.out.printf(" --- Demonstrating Gregorian Dates --- "); demoGregorianFromToday(); GregorianDate futureYearG = new GregorianDate(2100, 2, 27); demoDateGregorian2(futureYearG); GregorianDate leapYearG = new GregorianDate(2000, 2, 27); demoDateGregorian2(leapYearG); GregorianDate notLeapYearG = new GregorianDate(2001, 2, 27); demoDateGregorian2(notLeapYearG); GregorianDate endOfYearG = new GregorianDate(1999, 12, 30); demoDateGregorian2(endOfYearG); } /** * @brief Helper method use to exercise the capabilities of a concrete JulianDate class constructed from the * default constructor (today). * * @author James Dean Mathias */ public static void demoJulianFromToday() { JulianDate date = new JulianDate(); System.out.print("Today's date is : "); date.printLongDate(); System.out.println(); date.addDays(1); System.out.print("Tomorrow will be : "); date.printLongDate(); System.out.println(); date.subtractDays(2); System.out.print("Yesterday was : "); date.printLongDate(); System.out.println(); if (date.isLeapYear()) { System.out.println("This year is a leap year!"); } else { System.out.println("This year is not a leap year."); } } /** * @brief Helper method use to exercise the capabilities of a concrete GregorianDate class constructed from the * default constructor (today). * * @author James Dean Mathias */ public static void demoGregorianFromToday() { GregorianDate date = new GregorianDate(); System.out.print("Today's date is : "); date.printLongDate(); System.out.println(); date.addDays(1); System.out.print("Tomorrow will be : "); date.printLongDate(); System.out.println(); date.subtractDays(2); System.out.print("Yesterday was : "); date.printLongDate(); System.out.println(); if (date.isLeapYear()) { System.out.println("This year is a leap year!"); } else { System.out.println("This year is not a leap year."); } } /** * Helper method use to exercise the capabilities of the JulianDate class. * * @author James Dean Mathias */ public static void demoDateJulian2(JulianDate date) { System.out.println(); System.out.print("Demonstrating: "); date.printShortDate(); System.out.println(); System.out.print("Adding 1 day to the date : "); date.addDays(1); date.printShortDate(); System.out.println(); System.out.print("Adding another day : "); date.addDays(1); date.printShortDate(); System.out.println(); System.out.print("Just one more day : "); date.addDays(1); date.printShortDate(); System.out.println(); System.out.print("Going backwards by 2 days : "); date.subtractDays(2); date.printShortDate(); System.out.println(); } /** * Helper method use to exercise the capabilities of the GregorianDate class. * * @author James Dean Mathias */ public static void demoDateGregorian2(GregorianDate date) { System.out.println(); System.out.print("Demonstrating: "); date.printShortDate(); System.out.println(); System.out.print("Adding 1 day to the date : "); date.addDays(1); date.printShortDate(); System.out.println(); System.out.print("Adding another day : "); date.addDays(1); date.printShortDate(); System.out.println(); System.out.print("Just one more day : "); date.addDays(1); date.printShortDate(); System.out.println(); System.out.print("Going backwards by 2 days : "); date.subtractDays(2); date.printShortDate(); System.out.println(); } }
This is the first assignment in a two-part series. The overall goal is to create a set of classes that are capable of managing and displaying dates by the Gregorian and Julian calendars. The purpose of these two assignments is to have you learn about creating classes, encapsulation, abstraction, and polymorphism. The first in the series takes a naive view of creating classes, resulting in a program that has a lot of duplicated code. Then, in the second part, you'll clean up all of the duplication through the use of the Java programming language features, making a big improvement over the first assignment in the series. Many calendars have been in use throughout human history, depending upon the culture and time. Two that have most impacted western civilization are the Julian and Gregorian calendars. The Julian calendar was in use for many centuries, but has a problem in that it "gains" about three days every four centuries. The Gregorian calendar, more or less, solves this problem by changing how it computes leap years to better account for the time it takes the Earth to complete a full orbit. Both calendars have the same months and number of days in each month, including 29 days in February on leap years. As noted above, the Gregorian calendar has a different rule for computing leap years. Also of importance, the first valid date for the Gregorian calendar is October 15,1582. For the purposes of this assignment, we are mostly interested in the difference in how leap years are determined, and using that, want to represent dates using both calendars. For example, today (when I'm writing this) on the Gregorian calendar is February 17th,2020, but on the Julian calendar is February 4th,2020. The following details the requirements for the assignment: - Create two classes, and - The two date classes must expose only the following instance methods and constructors, and provides an implementation that is appropriate for the calendar: Default constructor that sets the date to the current date. See notes below about dates to help you figure out how to do this appropriately for each calendar. Overloaded constructor that takes year, month, and date values and sets the date to that date: and JulianDate(int year, int month, int day) A method that adds a specified number of days to the calendar date: - This number might be small, like one, or it might be large, like many thousands of days; don't assume it is a small number. A method that subtracts a specified number of days to the calendar date: - Same as above, this number of days might be small or very large. A method that returns true/false if the calendar date is part of a leap year: A method that prints the calendar date (without a carriage return) in mm/dd/yyyy format: void A method that prints the calendar date (without a carriage return) in Monthname dd, yyyy format: - The following 'get' accessor methods. These aren't used by the sample driver code, but would normally be part of a class like this. These accessor methods are used by the unit tests. These methods return values about the calendar date the object represents, which might be today or it might be something else...whatever the current state of the date object is. - Store the year, month, and day values as instance (non-static) data members of the class. - The two date classes may provide only the following methods, which you'll use in support of the other public instance methods: A method that returns true/false if the specified year is a leap year: A method that returns the number of days in a month (for a year): A method that returns the number of days in a year: - It is possible you don't use this method in your assignment implementation, and that is okay. I've added it because it can be useful for implementing a "fast" method. Even if you don't use it, still provide an implementation so you pass all the unit tests. A method that returns the name of a month; first letter capitalized, remainder lowercase: You will notice there are two methods. We want to use the method when doing things like determining how many days are in a month, for a particular year. The method returns true if the date represented by the object is a leap year; hmmm, seems like there is a method that helps do that :) Leap Years A wiki reference about leap years is found at: https://en.wikipedia.org/wiki/Leap_year - Julian calendar: If the remainder of the year when divided by 4 is 0 - Gregorian calendar (from wiki link above): "Every year that is exactly divisible by four is a leap year, except for years that are exactly divisible by 100 , but these centurial years are leap years if they are exactly divisible by 400". The date classes expose an method that take a number of days and add that to the date of the object. There are many different ways to do this, but one approach to solving the problem is this... - Loop over the number of days to add Add one to the object day If the object day is now larger than the number of days in the object month... - Set the object day to 1 - Add one to the object month - If the object month is now 13 - Set the object month to 1 - Add one to the object year When testing for the number of days in the object month, use the method, that is a problem you've already solved. It is possible to create more computationally efficient approaches to solving this problem, but the above is easy to understand. Once you understand how the above works, you can challenge yourself to make it faster. To subject days, you'll do something similar, but subtracting 1 day at a time. Computing "Today" The default constructors for each of the date classes have to figure out the date for "today". As noted in the previous section, the method is used to obtain the number of milliseconds that have occurred since January 1, 1970. That number is the same regardless of the calendar, any calendar. Note, the number returned from this method does not account for the time zone of the computer it is running on, you need to account for that. To get the time zone offset to correct for local time you can call This gives the number of milliseconds to "add" to the current time. I say "add" because it is a negative number, it is the offset you need. For the Gregorian calendar you can use this knowledge to do the following... 1. Initializing the date to 1/1/1970. 2. Get the number of milliseconds since 1/1/1970 and convert to days; remembering to account for the time zone as described above. 3. Add that many days to the date object by calling the method. 4. Viola, that is today. For the Julian calendar you can use this knowledge to do the following... 1. Set the starting date to 1/1/1 (January 1 , year 1 ). 2. Add in the number of days until (Gregorian) January 1, 1970. That number is 719164 ; Here is a handy reference to compute the number of days between two dates: http://www.csgnetwork.com/juliancountdaysfromtocalc.html 3. Get the number of milliseconds since 1/1/1970 and convert to days; remembering to account for the time zone as described above. 4. Add that many days to the date object by calling the method. 5. Viola, that is today. Unit Test Note: When running the unit tests after 12:00 am (midnight) and before 1:00 am, they might show a failure. This is because we aren't accounting for DST and the unit test code is. Be sure to check the unit tests outside of this time range to ensure you don't see this quirk. In thinking about how to write the code for this assignment, follow the concepts you learned from Stepwise Refinement and bottom up implementation. The Stepwise Refinement has been done for you, that is the various methods you are required to write are detailed above. Your responsibility is the bottom up implementation. Stub in the required methods, returning default values until you are able to write the correct implementation. Start with the base methods, such as the method and build up from there. Write small tests in the method to test each method as you go. Don't move on to another method until you have the one you are currently working on correctly implemented. In other words, build up your code slowly, one method at a time and validating as you go, rather than trying to write all of the methods and then debugging afterwards. - The very last item you should work on is the default constructor that computes "today". - The second to last item to write is the method, because it is similar to just in reverse. - The should be the third from last item you work on. Get all of the simple items written, that don't have much complexity to them. Once they are written, write the method. The provided skeleton project contains code in the Assign5.java file that exercises all of the features of both classes; link to project . You'll want to first comment this code and slowly uncomment as you build up your classes; similarly with the unit tests. Example Output The provided driver code will produce output like the following, depending, of course, on which day you run your code
Step by Step Solution
There are 3 Steps involved in it
Step: 1
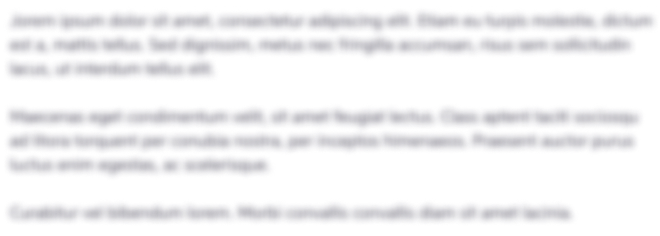
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started