Question
Describable.java This file defines an interface for objects that can be described. Methods describe() o Abstract method that does not take in anything and returns
Describable.java This file defines an interface for objects that can be described.
Methods describe() o Abstract method that does not take in anything and returns a description of the object as a String o (Note: any concrete class that implements Describable must provide a method definition for this method) GroceryItem.java This class represents a grocery item. do not make instance of this class (Hint: there is a keyword that prevents us from creating instances of a class). GroceryItem should implement the Comparable interface with the proper type parameter. All GroceryItems should also be Describable, but the implementation of the abstract method will be done only in the child classes.
Variables String name - The name of the item int itemCode - This represents a code given to the item double price - This represents a price of the item and will be used to compare and sort items
Constructors A constructor that takes in the name, itemCode, and price in that order and sets the instance variables accordingly
Methods
no methods other than those specified. Any extra methods will result in point deductions. All methods must have the proper visibility to be used where it is specified they are used. compareTo(GroceryItem other) o Override the compareTo method (You should be able to put @Override on the line before the method header) o Takes in a GroceryItem object and returns an int, adhering to the API contract (Comparable Interface) (This will only work if you have the proper generic type in the class header) o A GroceryItem is greater than another GroceryItem if its price is less than the other ones. For example, if GroceryItem itemOne has price = 8 and GroceryItem itemTwo has price = 5, itemOne is less than itemTwo. (Logically, this will allow us to sort from highest to lowest price). toString() o Returns a String describing the GroceryItem as follows: [itemCode]: [description] Note, this is a concatenation of the itemCode and output from describe() e.g. 80: This is a description. o Descriptions are handled in child classes o Must override toString() method defined in Object class
Grocery.java This file defines a class called Grocery.
Variables GroceryItem[] shelf - An array that simulates a shelf where we will hold objects of type GroceryItem. This array should not contain any null values for input. You don't have to check since we'll only input non-null values and full arrays but keep that in mind for your own testing. This array should always be sorted from highest price to lowest price.
Constructors A zero-argument constructor that initializes an empty shelf (size zero). A one argument constructor that takes in an array of GroceryItems that will be the items on the shelf. Remember that we want the shelf to be always sorted, and that you should not assume that the passed in array is sorted (there's an easy method for this operation)
Methods browseItems() o This method should print out the information of all GroceryItems in the shelf for the Grocery. o Format for the print statements: "[itemCode]: [description]" on a new line o Remember to disregard the brackets o The method should return nothing. o Hint: Think of what method could be useful to get the printed values addGroceryItem(GroceryItem newItem) o This method makes a new array of length + 1 that copies over all the previous elements as well as adds the new item. (Note: For Arrays.sort() to work, the array must not have any null values, which is why we keep it full) o It should then sort the array to maintain the shelf's order. o The method should return nothing. getGroceryItem(int code) o Returns the grocery item with given code. If the code doesn't exist, return null.
o If there are GroceryItems with the same code, return the first one found with the code. o The method should return a GroceryItem getNumberOfItems() o Returns the number of items on the shelf o The method should return an int Food.java
This file defines Food. Have Food extend the GroceryItem class.
Variables All variables must be not allowed to be directly modified outside the class in which they are declared, unless otherwise stated in the description of the variable. The Food class must have these variables. Do NOT re-declare any of the instance variables declared in GroceryItem class: age - an int that represents how old the Food is in months expiration - an int that represents how many months it takes for this Food to expire
Constructors A constructor that takes in the name, itemCode, price, age, and expiration and sets all fields accordingly. It must accept the variables in the specified order.
Methods no other methods than those specified. Any extra methods will result in point deductions. All methods must have the proper visibility to be used where it is specified they are used. calculateMonthsRemaining() o Return the difference between expiration and age Note, this is positive if the age is less than expiration, negative if it's greater describe() o Return "The food is [age] months old and has [remaining months value] months remaining before it expires." Note, the brackets should not be included in the returned String Supply.java
This file defines a supply item. A supply item is defined to be a non-food item that is sold at grocery stores, such as cleaning supplies, cards, etc. Have Supply extend the GroceryItem class.
Variables All variables must not be allowed to be directly modified outside the class in which they are declared, unless otherwise stated in the description of the variable. blurb - A string that represents a short description of the supply item.
Constructors A constructor that takes in the name, itemCode, price, description and sets all fields accordingly. It must accept the variables in the specified order.
Methods no other methods than those specified. Any extra methods will result in point deductions. All methods must have the proper visibility to be used where it is specified they are used. describe() o Return "The item looks like [short description]." without the brackets Note, the brackets should not be included in the returned String To prevent trivialization, you are only allowed to import java.util.Arrays. You are not allowed to import any other classes or packages.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
Heres the code for the requested classes adhering to the specifications and incorporating insights from the ratings Describablejava Java public interf...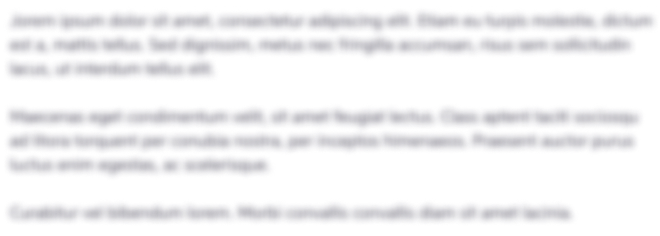
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started