Question
Describe and justify an algorithm for finding the shortest distance between each pair of vertices in an undirected graph in which each edge has a
Describe and justify an algorithm for finding the shortest distance between each pair of vertices in an undirected graph in which each edge has a given positive length. If there is no path between a pair of vertices a very large result should arise. (b) Is it sensible to use your algorithm to discover whether such a graph is connected? Suggest an alternative that would be appropriate for a graph of 1000 vertices and 10,000 edges.
answer all questions (a) What is a pipeline bubble and why might a branch instruction introduce one or more bubbles? (b) Explain, with the aid of an example, how conditional instructions may be used to reduce the number of bubbles in a pipeline. [4 marks] (c) What is the difference between branches, interrupts, software interrupts (initiated by a SWI instruction on the ARM) and exceptions? [8 marks] (d) What is an imprecise exception and why might a processor designer prefer it to a precise exception mechanism?
Information is to be conveyed from A to B using automatic repeat request (ARQ), forward error correction (FEC), and lossless compression. (a) Explain the terms ARQ, FEC and lossless compression. [5 marks] (b) If we consider each of these functions to be operating at different protocol layers, what would be the most sensible ordering of the layers, and why? [5 marks] (c) Suppose: The underlying bit channel has a capacity of B, a delay and error rate 0. The compression ratio is C < 1. The FEC has rate R < 1 and given an error rate 0 provides an error rate 1 (which is detected). The ARQ protocol has a window size of W. At what rate can the information be conveyed? [Hint: Consider when retransmissions are made.] State any assumptions you make about the operation of the ARQ protocol.
(a) Describe the limitations of human vision in terms of: (i) spatial resolution (ii) luminance (iii) colour and explain the implications that each of these limitations has on the design of display devices. [10 marks] (b) In image compression we utilise three different mechanisms to compress pixel data: (i) mapping the pixel values to some other set of values (ii) quantising those values (iii) symbol encoding the resulting values Explain each mechanism, describe the way in which it helps us to compress the image, and describe in what way it affects the visual quality of the resulting (decompressed) image when compared with the original. [10 marks] SECTION B 5 Comparative Programming Languages (a) Outline the main innovations that are in Simula 67 but were not in Algol 60, paying particular attention to Simula Classes. [6 marks] (b) Illustrate how Simula can be used to simulate a small restaurant with six tables, two waiters and small groups of customers arriving at random intervals. You need specify only the classes you would define. Most of the algorithmic details may be omitted. [6 marks] (c) Discuss to what extent Simula has been made redundant by the development of modern object-oriented languages such as Java.
Consider a language J which has Java-like syntax nested definitions of procedures within other procedures local variables (with static binding) raising and handling of named, parameterless exceptions Explain a possible run-time data structure which a compiler for J might use. [10 marks] A nave user of such a language suggests that the resultant compiled code will spend a significant fraction of execution time searchingboth finding the store location corresponding to the use of a variable name and finding the exception handler corresponding to the raising of a given exception name. Determine with justification whether this is so for your run-time data-structure proposed above. [4 marks] Now instead suppose a simple interpreter for J is written, so that searches for variable (or exception) names search the appropriate environment for the variable value or exception handler code. To what extent are these searches bounded by (a) the number of variables or exceptions in the program or (b) the dynamic or static nesting of procedures? [6 marks] 7 Prolog for Artificial Intelligence Consider the following Prolog program, which is intended to define the third argument to be the maximum value of the first two numeric arguments: max(X, Y, X) :- X >= Y, !. max(X, Y, Y). (a) Provide an appropriate query to show that the above program can give an incorrect result. [4 marks] (b) Explain the cause of the error. [6 marks] (c) Suggest a correction. [5 marks] (d) Write Prolog program to find the maximum of a list of numbers.
(a) Explain how to describe the structure of a collection of data using entities, attributes and relationships. [6 marks] (b) How would you identify particular instances of data in order to record the information in a database? Illustrate your answer by considering both a relational database maintained using SQL-92 and an ODMG database. [6 marks] (c) A high street bank has just announced a merger with a nationwide building society. You are employed as a consultant to advise on the integration of their client databases. Both institutions use relational databases. Write brief notes to alert the database administrators to the difficulties that they may encounter.
In a concurrent system it is usual to distinguish between safety properties and liveness properties. (a) What is meant by mutual exclusion, and how does a programmer ensure it is enforced in Java? Indicate whether it relates to safety or to liveness. [4 marks] (b) What is deadlock and how can it occur? Again, indicate whether it relates to safety or to liveness. [4 marks] A group of ten feeding philosophers has gathered to eat at a long trough, as shown below. They eat by leaning over the trough and so must be careful not to let their heads collide in the middle. To ensure safety they decide that around each position in the trough at most one philosopher is allowed to be eating at any one time. For instance, only one of 1, 2 or 9 could be eating at once, but there is no problem with 9, 3, 6 and 10 all eating together. 9 10 1 3 5 7 2 4 6 8 In a Java system, each philosopher is modelled by a thread which loops between eating and non-eating phases. There are four TroughPosition objects, corresponding to the four positions along the trough. Each philosopher has a reference to the position at which he or she is eating. (c) Define a Java class TroughPosition which supports methods startEating() and finishEating() to ensure safe execution for the philosophers at that position. [6 marks] (d) Can your solution suffer from deadlock? Either explain why it cannot occur, or explain how it could be avoided. [3 marks] (e) Can your solution suffer from starvation - that is, can one thread continuously remain in the startEating() method? Either explain why this cannot occur, or explain how it could be avoided. [3 marks] 4 CST.2003.5.5 SECTION B 5 Computer Graphics and Image Processing (a) Describe the A-buffer polygon scan conversion algorithm using 44 sub-pixels in each pixel. [10 marks] (b) It is possible to represent continuous tone greyscale images using just black ink on white paper because of limitations in the human visual system. Explain how and why. (c) Describe an algorithm which, given a greyscale image, will produce a black and white (bi-level) image of four times the resolution in each dimension which provides a good approximation to the greyscale image.
(a) Distinguish between the terms instance method and class method. [4 marks] (b) A newcomer to Java programming has written the following code: class Parent { public void test() { System.out.println("Parent"); } } public class Child extends Parent { public static void main(String[] args) { Parent p = new Parent(); Child c = new Child(); p.test(); c.test(); p = c; p.test(); c.test(); c = p; p.test(); c.test(); } public void test() { System.out.println("Child"); } } The javac compiler complains about one statement. Which one and why? Correct the code by inserting an appropriate cast. [4 marks] (c) With this correction the program will compile and run. Explain in outline what happens at run-time and show what output is printed. [5 marks] (d) Small print in the Java documentation says that you "cannot override a static method but you can hide it". If both test() methods are made static the program will again compile and run. Explain what happens this time and show what output is printed. [7 marks] 3 [TURN OVER CST.2005.10.4 3 Data Structures and Algorithms (a) Briefly outline how a sequence of symbols can be encoded as a sequence of Huffman codes, and explain under what assumptions Huffman encoding generates optimally compact code. [6 marks] (b) Estimate the number of bits needed to Huffman encode a random permutation of As, Bs and Cs, with each letter occurring one million times. [3 marks] (c) Estimate the number of bits needed to Huffman encode a random permutation of As, Bs and Cs, where A occurs two million times and B and C each occur one million times. [3 marks] (d) Estimate how many bits would be needed to encode the sequence in part (b) above using arithmetic coding. You may assume that log2 3 is about 1.6. [4 marks] (e) Estimate, with justification, how many bits would be needed to encode the sequence in part (c) above using arithmetic coding. [4 marks] 4 Artificial Intelligence I (a) What are the advantages and disadvantages of constraint satisfaction problem (CSP) solvers compared with search algorithms such as A? search, etc? [3 marks] (b) Give a general definition of a CSP. Define the way in which a solution is represented and what it means for a solution to be consistent and complete. [5 marks] (c) Assuming discrete binary constraints and finite domains, explain how breadthfirst-search might be used to find a solution and why this is an undesirable approach. [3 marks] (d) Give a brief description of the basic backtracking algorithm for finding a solution. [4 marks] (e) Describe the minimum remaining values heuristic, the degree heuristic and the least constraining value heuristic. [5 marks] 4 CST.2005.10.5 5 Comparative Programming Languages Consider the Prolog procedures named s and p defined as follows: s(H, [H|T], T). s(H, [N|T], [N|L]) :- s(H, T, L). p(X, [H|T]) :- s(H, X, Z), p(Z, T). p([], []). (a) Show how Prolog would evaluate the goal s(H, [a,b,c], T) giving all the successive instantiations of H and T that cause the goal to be satisfied, and hence describe in words what s does. [6 marks] (b) What value of Q causes the goal p([a], Q) to be satisfied? [3 marks] (c) What values of Q cause the goal p([a,b], Q) to be satisfied? [4 marks] (d) What values of Q cause the goal p([a,b,c], Q) to be satisfied? [5 marks] (e) Describe in words what p does.
(a) Distinguish between the terms instance method and class method. [4 marks] (b) A newcomer to Java programming has written the following code: class Parent { public void test() { System.out.println("Parent"); } } public class Child extends Parent { public static void main(String[] args) { Parent p = new Parent(); Child c = new Child(); p.test(); c.test(); p = c; p.test(); c.test(); c = p; p.test(); c.test(); } public void test() { System.out.println("Child"); } } The javac compiler complains about one statement. Which one and why? Correct the code by inserting an appropriate cast. [4 marks] (c) With this correction the program will compile and run. Explain in outline what happens at run-time and show what output is printed. [5 marks] (d) Small print in the Java documentation says that you "cannot override a static method but you can hide it". If both test() methods are made static the program will again compile and run. Explain what happens this time and show what output is printed. [7 marks] 3 [TURN OVER CST.2005.10.4 3 Data Structures and Algorithms (a) Briefly outline how a sequence of symbols can be encoded as a sequence of Huffman codes, and explain under what assumptions Huffman encoding generates optimally compact code. [6 marks] (b) Estimate the number of bits needed to Huffman encode a random permutation of As, Bs and Cs, with each letter occurring one million times. [3 marks] (c) Estimate the number of bits needed to Huffman encode a random permutation of As, Bs and Cs, where A occurs two million times and B and C each occur one million times. [3 marks] (d) Estimate how many bits would be needed to encode the sequence in part (b) above using arithmetic coding. You may assume that log2 3 is about 1.6. [4 marks] (e) Estimate, with justification, how many bits would be needed to encode the sequence in part (c) above using arithmetic coding. [4 marks] 4 Artificial Intelligence I (a) What are the advantages and disadvantages of constraint satisfaction problem (CSP) solvers compared with search algorithms such as A? search, etc? [3 marks] (b) Give a general definition of a CSP. Define the way in which a solution is represented and what it means for a solution to be consistent and complete. [5 marks] (c) Assuming discrete binary constraints and finite domains, explain how breadthfirst-search might be used to find a solution and why this is an undesirable approach. [3 marks] (d) Give a brief description of the basic backtracking algorithm for finding a solution. [4 marks] (e) Describe the minimum remaining values heuristic, the degree heuristic and the least constraining value heuristic. [5 marks] 4 CST.2005.10.5 5 Comparative Programming Languages Consider the Prolog procedures named s and p defined as follows: s(H, [H|T], T). s(H, [N|T], [N|L]) :- s(H, T, L). p(X, [H|T]) :- s(H, X, Z), p(Z, T). p([], []). (a) Show how Prolog would evaluate the goal s(H, [a,b,c], T) giving all the successive instantiations of H and T that cause the goal to be satisfied, and hence describe in words what s does. [6 marks] (b) What value of Q causes the goal p([a], Q) to be satisfied? [3 marks] (c) What values of Q cause the goal p([a,b], Q) to be satisfied? [4 marks] (d) What values of Q cause the goal p([a,b,c], Q) to be satisfied? [5 marks] (e) Describe in words what p does. [2 marks] 5 [TURN OVER CST.2005.10.6 6 Operating System Foundations (a) A device driver process carries out character I/O via a Universal Asynchronous Receiver/Transmitter (UART). (i) Why is hardware-software synchronisation needed? [1 mark] (ii) Describe polled operation. [2 marks] (iii) Describe interrupt-driven operation. [2 marks] (iv) Draw a state transition diagram for the device-driver process. Indicate the events that cause each transition and in each case explain the effect on the device driver's process descriptor and the operating system's scheduling queues. Assume interrupt-driven software. [7 marks] (b) The device driver process fills/empties a buffer of fixed size in an I/O buffer area. A process carrying out application requests reads and writes data in variable-sized amounts from the buffer. (i) Why must mutually exclusive access to the buffer be enforced? [2 marks] (ii) Why is condition synchronisation needed?
(a) A device driver process carries out character I/O via a Universal Asynchronous Receiver/Transmitter (UART). (i) Why is hardware-software synchronisation needed? [1 mark] (ii) Describe polled operation. [2 marks] (iii) Describe interrupt-driven operation. [2 marks] (iv) Draw a state transition diagram for the device-driver process. Indicate the events that cause each transition and in each case explain the effect on the device driver's process descriptor and the operating system's scheduling queues. Assume interrupt-driven software. [7 marks] (b) The device driver process fills/empties a buffer of fixed size in an I/O buffer area. A process carrying out application requests reads and writes data in variable-sized amounts from the buffer. (i) Why must mutually exclusive access to the buffer be enforced? [2 marks] (ii) Why is condition synchronisation needed?
(a) A Java static method is defined in class C by class C { public static int f(int x, int y) { int z = x; ...; return x+y*z; } } where '...' represents commands the details of which are not important to this question. It is called in an expression e of the form f(f(1,2), f(3,4)) Give JVM (or other stack machine) code corresponding to the expression e and explain how this is derived from the syntax tree for e. [6 marks] (b) Explain how the body of f above is mapped into JVM (or other stack machine) code, explaining the role of the registers FP and SP (precise details are not important, but their role should be well explained). You may write '...' for the translation of the '...' in f. [6 marks] (c) Consider the Java class definitions: class A { public int a1, a2; public void m() { println("I am an A with " + a1 + " and " + a2); } } class B extends A { public int b1, b2; public void m() { println("I am a B with " + a1 + " and " + a2 + " also with " + b1 + " and " + b2); } } Describe the run-time storage layout for objects of class A and for those of class B, particularly noting the size and offsets of members and how a cast of an object of type class B to one of class A can be achieved. Explain how calls to m() work, particularly in code like: public static void g(B x) { h(x); } public static void h(A x) { x.m(); }
Write complete C++ program to ask the user to enter 4 integers. The program must use a function to find the LARGEST integer among them and print it on the screen. a function that accepts three arguments: an array, the size of the array, and a number n. Assume that the array contains integers. The function should display all of the numbers in the array that are greater than the numbern.
negative. java program that takes both n (as input) and n integer inputs and add the given values to a defined array
The following Prolog relation appends a list A to a list B to give a list C. append([],Y,Y). append([H|T],Y,[H|Z]) :- append(T,Y,Z). (a) Using the append relation, write a Prolog predicate insert(X,Y,Z) that is true if X can be inserted into a list Y to give a list Z. Your relation should be capable of using backtracking to generate all lists obtained from Y by inserting X at some point, using a query such as: insert(c,[a,b],Z). to obtain Z=[c,a,b], Z=[a,c,b], and Z=[a,b,c] and it should generate each possibility exactly once. [5 marks] (b) Using the insert relation, write a Prolog predicate perm(X,Y) that is true if a list Y is a permutation of a list X. Your predicate should respond to a query such as perm([a,b,c],Y) by using backtracking to generate all permutations of the given list. [6 marks] (c) We have a list of events [e1,e2,...,en]. A partial order can be expressed in Prolog by stating before(e3,e4). before(e1,e5). and so on, where before(a,b) says that event a must happen before event b (although not necessarily immediately before). No ordering constraints are imposed other than those stated using before. Given a list of events, a linearisation of the list is any ordering of its events for which none of the before constraints are broken. Given the example above and the list [e1,e2,e3,e4,e5], one valid linearisation would be [e3,e1,e2,e5,e4]. However, [e4,e2,e1,e5,e3] is not a valid linearisation because the first before constraint does not hold. Using the perm predicate or otherwise, and assuming that your Prolog program contains before constraints in the format suggested above, write a Prolog predicate po(X,Y) that is true if Y is a valid linearisation of the events in the list X. Your relation should be capable of using backtracking to generate all valid linearisations as a result of a query of the form po([e1,e2,e3,e4,e5],Y).
(a) (i) Define the operators in the core relational algebra. [5 marks] (ii) Define the domain relational calculus. [4 marks] (iii) Show how the relational algebra can be encoded in the domain relational calculus.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
Lets break down the questions and answer them one by one Finding Shortest Distance in an Undirected Graph One commonly used algorithm for finding the ...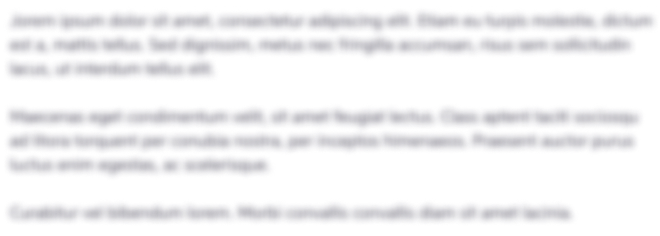
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started