Question
Description and Specifications Build a program that will shuffle a deck of cards. The deck of cards can be represented by integers 1 through 52.
Description and Specifications
Build a program that will shuffle a deck of cards. The deck of cards can be represented by integers 1 through 52. One way you could shuffle the cards is to generate a permutation of the integers. A permutation is a way in which a set of number of things can be ordered or arranged. Randomize the integers 1-52 such as in the example below. There are numerous ways to accomplish this but for the lab follow the steps below. Put your functions in Permutations.cpp. Create a header file (Permutations.h) and only put the first two function prototypes in the header. Follow the steps below in a driver program named Driver.cpp.
Using the initPermutation function, create an array of integers from 1 to n
Whenever the user calls nextPermutation generate a random index from 1 to the current size of the array
Obtain the integer at the randomly generated array index
Call remove Permutation from nextPermutation (the user never directly calls this function) to remove the integer from the array, shifting the remaining elements down in the array to fill the gap.
Return the integer pulled from the array and then print it out.
Release the dynamically allocated memory.
There are several necessary functions to enable this solution. The functions below should be in Permutations.cpp:
int* initPermutation(int n); //create and return an array of integers 1 to n
int nextPermutation (int* permutation, int& permuteSize);
void removePermutation (int* permutation, int permuteSize, int removeIndex);
Sample Output
Below is a possible output if n = 10. Remember yours should be different:
Deck Permutations: 4 2 7 5 9 1 8 3 10 6
Step by Step Solution
There are 3 Steps involved in it
Step: 1
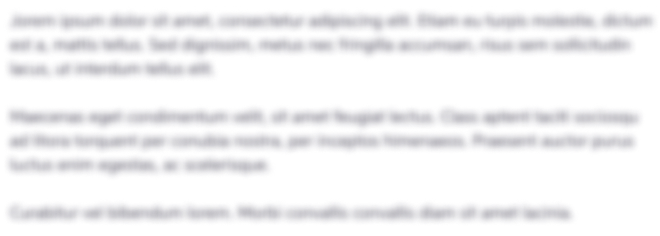
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started