Question
Description For this assignment, you will read in a signed int, which will be a base 10 number, from the terminal and you will convert
Description For this assignment, you will read in a signed int, which will be a base 10 number, from the terminal and you will convert the number into a signed binary number that represents that base 10 number read from input. The binary number will be represented by an array of type bool and will contain 32 elements (since all integers are 4 bytes = 32 bits). The way we will store the number is the element at index 0 of the boolean array will represent the sign, so if element at index 0 is true then the number will be negative and if it is false then the number is positive. The elements from indices 1 to 31 will represent the number in binary format where true represents 1 and false represents 0. Suppose if we have the number 65 in binary using our format the contents of the boolean array will be 00000000000000000000000001000001 since 1000001 is 65 in binary and the leftmost bit is 0 so it must be positive, and for -12 would be 10000000000000000000000000001100 since 1100 is 12 in binary and the leftmost bit is 1 so it must be negative and the leading zeros represent no value. When outputting the binary number you will print a - if the leftmost bit contains 1 or true and you will not output the leading zeros.
Specifications For the assignment, to get full credit your program must contain/perform the following You must have an array of bool to store the binary number using the format explain earlier, you are only allowed to have an array of booleans, no strings or array of any other type You must perform error checking if the user enters some non integer type, in that case you need to clear and ignore the input buffer and re-prompt the user until the input entered is valid (you dont need to check for the range of the integer)
I have most of it working except for the negative numbers. For example:
Enter a number : -2147483647
-2147483647 in binary is -11111111111111111111111111111111
I will out 111111111111111111111111111 without the negative sign in front and i cant figure out why. Here's my code:
#include
using namespace std;
const int SIZE = 32;// constant for array size
void decToBinary(int n) //function to convert decimal to binary
{
bool binNum[SIZE];// array to store binary number
//counter for binary array
int i = 0;
while (n > 0)
{
binNum[i] = n % 2;// storing remainder in binary array
n = n/2;
i++;
}
for(int j = i - 1; j >= 0; j--)// printing binary array in reverse order
cout << binNum[j];
}
void neg_decToBinary(int n)// functin to convert negative decimal to binary
{
bool binNum[SIZE];
int i = 0;
int pos = 31;//LMB
while (n != 0)
{
binNum[pos] = n % 2;
n = n/2;
pos--;
}
if(binNum[pos] == 1)// if LMB is negative
{
cout << " - ";
}
for(int i= pos + 1; pos + 1 < SIZE; pos++)
cout << binNum[pos+1];
}
int main()
{
int n;
cout << "Enter a number:" << endl;
cin >> n;
while(cin.fail())// checking for input failure
{
cout << "Enter a number:" << endl;// reprompts user
cin.clear();// clear input stream
cin.ignore(100, ' ');// ignore till the next line or character
cin >> n;
}
if(n == 0 || n == 1 || n == -1)// checking if the input equals one of these numbers
{
cout << "The binary form of " << n << " is " << n;
cout << endl;
}
else if(n > 1)// if input is greater than 1
{
cout << "The binary form of " << n << " is ";
decToBinary(n);
cout << endl;
}
else// must be negative number
{
cout << "The binary form of " << n << " is ";
neg_decToBinary(n);
cout << endl;
}
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
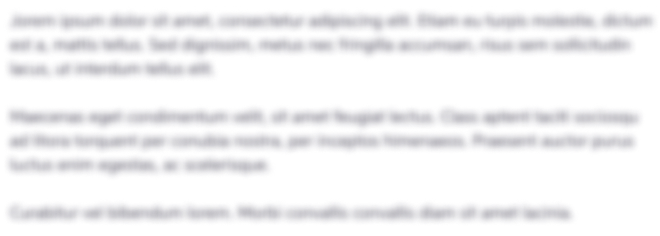
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started