Question
Description In this homework, you will practice sorting algorithms and recursive functions. From a text file containing several integers which youll save into an array,
Description
In this homework, you will practice sorting algorithms and recursive functions. From a text file containing several integers which youll save into an array, youll need to print out the original array, sort the array according to provided arguments, and then print the final sorted array.
Problem
You will be given a text file. This text file contains several integers that need to be sorted (in either ascending or descending order). Sort these integers, and then print the sorted array to the screen (cout) in accordance with the arguments provided.
Instructions on how to implement the sorting algorithm are listed later in this document. We will be giving you an outline of how it works, but you will be implementing that functionality yourself.
Weve given you a list of functions (see purple text later in document) that will need to be implemented, but the parameters that you use and the contents are entirely up to you. You will be reading your arguments from the command line. The name of the input file will be passed as argument 1, argv[1] . The number of lines in the file will be passed as argument 2, argv[2] . The sorting order (ascending or descending) will be passed as argument 3, argv[3] . The string ascending or descending will be given in lowercase . The sorting type (normal or recursive) will be passed as argument 4, argv[4] .
Text File Contents
The text file will contain several integers, each on a different line. The number of lines passed in as an argument will always be consistent with number of lines in the text file when we grade this.
Requirements/Instructions
Read all numbers from the text file and save them into an array. You may name your array as you like.
For every function, you can use as many parameters as you like, and may name them whatever you like as well.
You are required to create two functions getMin() and getMax() . These functions should accept at the least, an array and its size. From there, the function should calculate the min/max value contained within that array, and return a pointer to the address containing it.
getMin() : will return a pointer to the minimum value of the array.
getMax() : will return a pointer to the maximum value of the array.
Sorting the array: You are NOT allowed to use any standard sorting algorithms, you must implement the one provided (see end of document). Please create the following functions:
normalSort() : This function will sort an array in a given order (ascending or descending depending on an argument passed in) by applying the getMin() or getMax() functions above.
recursiveSort() : This function will apply the same sorting method as normalSort , but in a recursive manner.
Use a function swap(int &first, int &second) to swap two array elements.
Printing out arrays: You should print out the array both before sorting, and after sorting using a function printArray() . Once youve written the function normally, implement a recursive version that does the same thing.
printArray() : This function will print an array.
printArrayRec() : This function recursively prints the array.
Note: If you find yourself repeating any code more than once or twice, make a function out of it. This will make your code both easier to read as well as test.
Sorting Algorithm - Ascending
Key :
RED boxes indicate the current minimum
GREEN boxes indicate array elements for which sorting is already completed
YELLOW indicates the current array element/index that we are working with.
Example:
Given an array such as the one below, complete the following:
1. Find the minimum value contained in the array.
2. Swap that minimum value with the first element in the array
3. Then, move onto the second element.
4. Find the minimum value that occurs either in or AFTER the current elements index (i.e. not including those that are already sorted).
5. Swap the new minimum value with the current element.
6. You have now sorted the first 2 elements. Repeat the steps until the array is fully sorted.
Please be patient and scroll down to read the whole instructions. If you do not follow the instruction carefully, and your output does NOT match requirements, DO NOT email any TAs to ask for petition/regrade. This is automatically graded by computer program, and we ARE able to check whether a function displays recursive behavior. Everyones output must MATCH the below sample exactly .
Sample Input : (Dont copy paste the input file, please type it yourself)
sortingNumbers.txt :
Sample Outputs : Output on screen (cout)
./hw5.out sortingNumbers.txt 10 ascending normal
./hw5.out sortingNumbers.txt 10 ascending recursive
./hw5.out sortingNumbers.txt 10 descending normal
./hw5.out sortingNumbers.txt 10 descending recursive
Submission :
- Place your hw5.cpp file in the Linux server in your hw5 folder. If your file is NOT named exactly that, you will receive 0 Points.
- PLEASE test your program on the linux server before the due date.
- Do NOT hardcode the input filename. (0 Points if you do so)
- Do NOT hardcode the number of lines in the text file. (0 Points if you do so)
- Compile your program using the g++ command, and name your executable hw5.out.
g++ -std=c++11 hw5.cpp -o hw5.out
- You must use command line arguments for this assignment.
- Use Google and Stackoverflow, they are valuable resources!
- Dont use code that you dont understand. Not only will it be much harder to debug, but it may have different behavior than expected in certain situations, and cause test cases to fail that you didnt expect.
- Please DO NOT copy from friends, or any public sources completely. If we find that you have extremely similar code to another student, you will get 0 Points immediately,
- Hint : If you are struggling to learn recursion, focus on implementing the recursive printing function before you work on the sorting.
80 23 5 91 19Step by Step Solution
There are 3 Steps involved in it
Step: 1
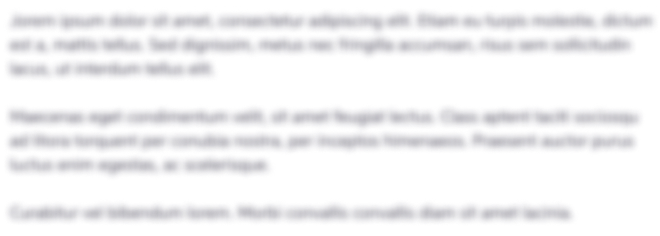
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started