Question
Description In this part of the assignment you are to generate random shapes, in random a position and filled with a random color. Please follow
Description
In this part of the assignment you are to generate random shapes, in random a position and filled with a random color.
Please follow the instructions as specified in the PDF file.
Input
There is no input.
Output
Your program MUST not print out anything to the standard output. Instead, it must create an HTML file containing the HTML and SVG code to visualize the random shapes.
Sample Input 1
No input
Sample Output 1
No output, just an HTML file
Hint
Use the constants defined in the following template to solve this part. Please make sure to submit only the functions createFile and genRandomArt.
#include#include #include #define ID0 ("") #define ID1 (" ") #define ID2 (" ") #define FILENAME ("A5P4.html") #define FOPENERR ("fopen error %s") #define TABTITLE ("My CSC 111 Art") #define WELCOME ("Welcome to my CSC 111 Webpage") #define RESUME ("SVG is a great skill for my co-op job resume") #define ANIMATIONTEXT ("My CSC 111 Art ...") #define WINWIDTH (800) #define WINHEIGHT (320) // helper macro to convert a value into a string #define STR_HELPER(x) #x #define STR(x) STR_HELPER(x) #define MAXNUMSHAPES 1000 #define STRSH (STR(MAXNUMSHAPES) " shapes generated") #define SHAPEMIN (0) #define SHAPEMAX (2) #define XMIN (0) #define XMAX (WINWIDTH) #define YMIN 0 #define YMAX (WINHEIGHT) #define RADMIN (10) #define RADMAX (50) #define FALLSHADE (1) #define REDSHADE (2) #define GREENSHADE (3) #define BLUESHADE (4) #define PURPLESHADE (5) #define FAVORITESHADE (6) #define SHADE (FAVORITESHADE) #if SHADE == FALLSHADE #define REDMIN (100) #define REDMAX (255) #define GREENMIN (100) #define GREENMAX (255) #define BLUEMIN (0) #define BLUEMAX (100) #elif SHADE == REDSHADE #define REDMIN (200) #define REDMAX (255) #define GREENMIN (50) #define GREENMAX (120) #define BLUEMIN (0) #define BLUEMAX (50) #elif SHADE == GREENSHADE #define REDMIN (0) #define REDMAX (50) #define GREENMIN (150) #define GREENMAX (255) #define BLUEMIN (50) #define BLUEMAX (150) #elif SHADE == BLUESHADE #define REDMIN (0) #define REDMAX (50) #define GREENMIN (50) #define GREENMAX (150) #define BLUEMIN (150) #define BLUEMAX (250) #elif SHADE == PURPLESHADE #define REDMIN (75) #define REDMAX (150) #define GREENMIN (50) #define GREENMAX (130) #define BLUEMIN (170) #define BLUEMAX (255) #elif SHADE == FAVORITESHADE #define REDMIN (163) #define REDMAX (255) #define GREENMIN (68) #define GREENMAX (178) #define BLUEMIN (4) #define BLUEMAX (42) #endif //SHADE #define OPMIN (0.6) #define OPMAX (1.0) #define PRECISION (100.0) //prototypes int main(void); int prng(int iLow, int iHigh); float prngFloat(float fLow, float fHigh, float precision); FILE* createFile(char* fnam); void prologue(FILE* ofp, char* winTabTitle, char* opening, char* col); void epilogue(FILE* ofp, char* closing, char* col); void drawHeader1HTML5(FILE* ofp, char* id, char* text, char* col); void drawParaHTML5(FILE* ofp, char* id, char* text, char* col); void openSVG(FILE* ofp, char* id, int w, int h); void closeSVG(FILE* ofp, char* id); void commentSVG(FILE* ofp, char* id, char* cmt); void fillRectSVG(FILE* ofp, char* id, int x, int y, int w, int h, int r, int g, int b, float op); void fillCircleSVG(FILE* ofp, char* id, int cx, int cy, int rad, int r, int g, int b, float op); void textSwipeAnimateSVG(FILE* ofp, char* id, char* txt, int fsz, int x, int y, int r, int g, int b, float op); void genRandomArtNumSet(int *sh, int *x, int *y, int *rad, int *rx, int *ry, int *w, int *h, int *r, int *g, int *b, float *op) ; void genRandomArt(FILE *ofp, char *id, int nShapes); int main(void){ FILE *ofp = createFile(FILENAME); if (ofp == NULL){ printf("createFile function not written yet "); } else { prologue(ofp, TABTITLE, WELCOME, "red"); genRandomArt(ofp, ID2, MAXNUMSHAPES); epilogue(ofp, RESUME, "blue"); }//if return EXIT_SUCCESS; }//main int prngInt(int iLow, int iHigh) { // Replace with your code return 0; }//prngInt float prngFloat(float fLow, float fHigh, float precision) { // Replace with your code return 0.0; }//prngFloat void genRandomArtNumbers(int n) { // Replace with your code }//genRandomArtNumbers void genRandomArtNumSet(int *sh, int *x, int *y, int *rad, int *rx, int *ry, int *w, int *h, int *r, int *g, int *b, float *op) { *sh = *x = *y = *rad = *rx = *ry = *w = *h = *r = *g = *b = 0; *op = 1.0; // replace with your code from Part III of Assignment 5 }//genRandomArtNumbers void genRandomArt(FILE *ofp, char *id, int nShapes) { int x, y, rad, rx, ry, w, h, r, g, b; float op; commentSVG(ofp, ID1, "Outline SVG viewbox"); openSVG(ofp, ID1, WINWIDTH, WINHEIGHT); commentSVG(ofp, ID2, "Define and paint SVG view box"); fillRectSVG(ofp, ID2, 0, 0, WINWIDTH, WINHEIGHT, 245, 245, 220, 1.0); commentSVG(ofp, ID2, "Draw SVG shapes"); for (int k = 1; k "); fprintf(ofp, "%s%s ", ID0, ""); fprintf(ofp, "%s%s ", ID0, ""); fprintf(ofp, "%s%s ", ID1, " "); fprintf(ofp, "%s%s ", ID2, winTabTitle); fprintf(ofp, "%s%s ", ID1, " "); fprintf(ofp, "%s%s ", ID0, ""); fprintf(ofp, "%s%s ", ID0, ""); drawHeader1HTML5(ofp, ID1, opening, col); }//prologue void epilogue(FILE *ofp, char *closing, char *col) { drawParaHTML5(ofp, ID1, STRSH, col); drawParaHTML5(ofp, ID1, closing, col); fprintf(ofp, "%s%s ", ID0, ""); fprintf(ofp, "%s%s ", ID0, ""); }//epilogue void openSVG(FILE *ofp, char *id, int w, int h) { fprintf(ofp, "%s ", id); }//closeSVG void fillRectSVG(FILE *ofp, char *id, int x, int y, int w, int h, int r, int g, int b, float op) { fprintf(ofp, "%s", id, x, y, w, h, r, g, b, op); }//fillRectSVG void fillCircleSVG(FILE *ofp, char *id, int cx, int cy, int rad, int r, int g, int b, float op) { fprintf(ofp, "%s ", id, cx, cy, rad, r, g, b, op); }//fillCircleSVG void defAnimateSwipeSVG(FILE *ofp, char *id, char *link, int f, int t, int b, int d) { fprintf(ofp, "%s ", id); fprintf(ofp, "%s ", id); }//defAnimateSwipeSVG void textSwipeAnimateSVG(FILE *ofp, char *id, char *txt, int fsz, int x, int y, int r, int g, int b, float op) { defAnimateSwipeSVG(ofp, ID2, "defAnimateSwipeSVG", 500, 50, 0, 5); fprintf(ofp, "%s", id, link, f, t, b, d); fprintf(ofp, "%s %s ", id, "defAnimateSwipeSVG", x, y, fsz, r, g, b, op, txt); }//textSwipeAnimateSVG void commentSVG(FILE *ofp, char *id, char *cmt) { fprintf(ofp, "%s ", id, cmt); }//commentSVG void drawHeader1HTML5(FILE *ofp, char *id, char *text, char *col) { fprintf(ofp, "%s%s
", id, col, text); }//drawHeader1HTML5 void drawParaHTML5(FILE *ofp, char *id, char *text, char *col) { fprintf(ofp, "%s%s
", id, col, text); }//drawParaHTML5
PDF instruction:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
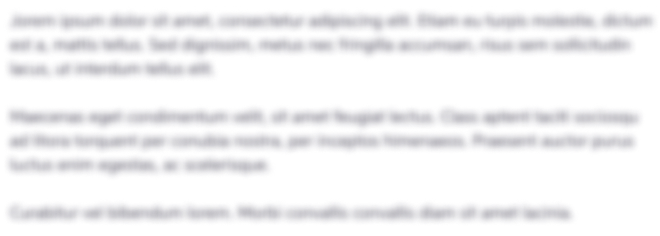
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started