Question
Description: Students are asked to design and code a multi-threading web server. The web client and server must follow the http protocol version 1.1. Requirements:
Description: Students are asked to design and code a multi-threading web server. The web client and server must follow the http protocol version 1.1. Requirements: The server must store html and jpeg files. When the requested file is not available in the server, the server must send the appropriate message to the client. The web server must be compatible with Chrome.
Note: I still missing the part of the requested file when is not available in the server,store html and jpeg files.
This is my code:
/* WebServer.java */ //package web_server; import java.net.ServerSocket; import java.net.Socket; public final class WebServer { public static void main(String argv[]) throws Exception { int port = 5555; ServerSocket WebSocket = new ServerSocket(port); while (true) { // Listen for a TCP connection request. Socket connectionSocket = WebSocket.accept(); //Construct object to process HTTP request message HttpRequest request = new HttpRequest(connectionSocket); Thread thread = new Thread(request); thread.start(); //start thread } } }
/*
HttpRequest.java
*/
//package web_server;
import java.io.*;
import java.net.*;
import java.util.*;
public final class HttpRequest implements Runnable {
final static String CRLF = " ";//For convenience
Socket socket;
// Constructor
public HttpRequest(Socket socket) throws Exception
{
this.socket = socket;
}
// Implement the run() method of the Runnable interface.
public void run()
{
try {
processRequest();
} catch (Exception e) {
System.out.println(e);
}
}
private void processRequest() throws Exception
{
InputStream is = socket.getInputStream();
DataOutputStream os = new DataOutputStream(
socket.getOutputStream());
// Set up input stream filters.
BufferedReader br = new BufferedReader(
new InputStreamReader(is));
String requestLine = br.readLine();
System.out.println(); //Echoes request line out to screen
System.out.println(requestLine);
//The following obtains the IP address of the incoming connection.
InetAddress incomingAddress = socket.getInetAddress();
String ipString= incomingAddress.getHostAddress();
System.out.println("The incoming address is: " + ipString);
//String Tokenizer is used to extract file name from this class.
StringTokenizer tokens = new StringTokenizer(requestLine);
tokens.nextToken(); // skip over the method, which should be GET
String fileName = tokens.nextToken();
// Prepend a . so that file request is within the current directory.
fileName = "." + fileName;
String headerLine = null;
while ((headerLine = br.readLine()).length() != 0) { //While the header still has text, print it
System.out.println(headerLine);
}
// Open the requested file.
FileInputStream fis = null;
boolean fileExists = true;
try {
fis = new FileInputStream(fileName);
} catch (FileNotFoundException e) {
fileExists = false;
}
//Construct the response message
String statusLine = null; //Set initial values to null
String contentTypeLine = null;
String entityBody = null;
if (fileExists) {
statusLine = "HTTP/1.1 200 OK: ";
contentTypeLine = "Content-Type: " +
contentType(fileName) + CRLF;
} else {
statusLine = "HTTP/1.1 404 Not Found: ";
contentTypeLine = "Content-Type: text/html" + CRLF;
entityBody = "
}
//End of response message construction
// Send the status line.
os.writeBytes(statusLine);
// Send the content type line.
os.writeBytes(contentTypeLine);
// Send a blank line to indicate the end of the header lines.
os.writeBytes(CRLF);
// Send the entity body.
if (fileExists) {
sendBytes(fis, os);
fis.close();
} else {
os.writeBytes(entityBody);
}
os.close(); //Close streams and socket.
br.close();
socket.close();
}
//Need this one for sendBytes function called in processRequest
private static void sendBytes(FileInputStream fis, OutputStream os)
throws Exception
{
// Construct a 1K buffer to hold bytes on their way to the socket.
byte[] buffer = new byte[1024];
int bytes = 0;
// Copy requested file into the sockets output stream.
while((bytes = fis.read(buffer)) != -1 ) {
os.write(buffer, 0, bytes);
}
}
private static String contentType(String fileName)
{
if(fileName.endsWith(".htm") || fileName.endsWith(".html"))
return "text/html";
if(fileName.endsWith(".jpg"))
return "text/jpg";
if(fileName.endsWith(".gif"))
return "text/gif";
return "application/octet-stream";
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
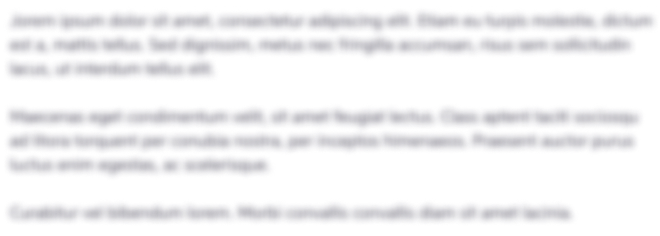
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started