Question
Description This assignment exercises your understanding of operator overloading in C++. You must overload all appropriate operators. As with other design details, type definition is
Description
This assignment exercises your understanding of operator overloading in C++. You must overload all appropriate operators. As with other design details, type definition is concerned with consistency. Focus on the expectations of the application programmer.
CaesarCipher class
The specification file for the CaesarCipher class has been provided for you. Your job will be to complete the implementation file and sufficiently test the CaesarCipher functionality in the test driver.
// CaesarCipher.h #ifndef CAESARCIPHER_H #define CAESARCIPHER_H #includeusing namespace std; class CaesarCipher { public: CaesarCipher(); CaesarCipher(const CaesarCipher &); string encrypt(string); string decrypt(string); CaesarCipher& operator = (const CaesarCipher &); CaesarCipher operator + (const CaesarCipher &); bool operator == (const CaesarCipher &); bool operator < (const CaesarCipher &); bool operator > (const CaesarCipher &); CaesarCipher& operator ++ (); CaesarCipher operator ++ (int); private: int shift; const int OFFSET_MIN = 32; const int OFFSET_MAX = 126; int getShift(); string encryptDecrypt(string, bool); bool isPositionInRange(int); }; #endif
Driver
A partial main.cpp test driver has been provided. Sufficiently test all the Big 4 (minus the destructor) and all the overloaded operators in the CaesarCipher class. Please make a comment on what exactly you're testing, so it's obvious to the person running your program. For example, how would you show the result of two CaesarCipher object added together? What will happen when the CaesarCipher object is incremented (pre vs postfix)?
Sample Output
- output1-2.txt
main.cpp starter code,
#include "CaesarCipher.h" #include#include using namespace std; int main() { CaesarCipher cc; string encryptedWord = cc.encrypt("Hello, world!"); cout << "Encrypted: " << encryptedWord << endl; string decryptedWord = cc.decrypt(encryptedWord); cout << "Decrypted: " << decryptedWord << endl; return 0; }
Class requirements
- Do not make any changes to the specification file beyond comments. You may also add private fields and functions, if necessary.
- Full documentation is not necessary, but include a description (in comments) for each public constructor and function.
- The comments should be included in the specification file ONLY.
- Consider what it means:
- to compare two CaesarCipher objects (i.e. ==, <, > operators)
- to add (i.e. + operator) two CaesarCipher objects
- to increment (pre and postfix) two CaesarCipher objects
- Communicate assumptions and use clearly in the documentation.
- You may reference and reuse portions of the CaesarCipher class from HW 2: Password Vault. Note that this assignment does not require the Encryptor interface.
- StringBuilder is not available in C++; you can use string concatenation.
- The exception thrown in encryptDecrypt() may be handled using the following code:
if (!isPositionInRange(indx)) throw invalid_argument("String to be encrypted has unrecognized character " + temp); // where temp is unrecognized character // note: you cannot concatenate a string with a character in C++
- When attempting to get random integers using the rand() function (and using srand() to get the seed value), you may be getting the same shifts every time. One way to resolve this is to create a static variable and check if the variable is set. If no, then call srand(); if yes, skip the call to srand().
// in .h, declare static variable, ie. isSeeded // in .cpp, set variable to false // in .cpp (i.e. getShift or constructor) if (!isSeeded) { // call srand() isSeeded = true; } // ... other code
- Name your files CaesarCipher.h, CaesarCipher.cpp, and main.cpp. No additional classes should be created.
Extra credit
Modify the IntegerList class to hold a list of CaesarCipher objects. The specification file (CaesarCipherList.h) is provided:
// CaesarCipherList.h // Specification file for the CaesarCipherList class. #ifndef CAESARCIPHERLIST_H #define CAESARCIPHERLIST_H #include "CaesarCipher.h" class CaesarCipherList { public: // Constructor CaesarCipherList(int); // Destructor ~CaesarCipherList(); // Copy constructor CaesarCipherList(const CaesarCipherList &); // Overloaded assignment operator CaesarCipherList& operator=(const CaesarCipherList &); // Add an element to the list void addElement(CaesarCipher); // Get element in the list at the provided index CaesarCipher getElement(int) const; // Get list size; int size() const; private: CaesarCipher *list; int capacity; // Capacity of array int numElements; // Number of elements in array void resize(); // Called to resize array when full }; #endif
- Do not change the CaesarCipher.h and CaesarCipher.cpp files.
- Feel free to rename the IntegerList class files to CaesarCipherList.h and CaesarCipherList.cpp and make the proper modifications to match the specification file shown above.
- Include Big 4 implementation: constructor, copy constructor, overloaded assignment operator, and destructor.
- Test all functionality in the test driver (i.e. main.cpp).
- Note that you made need to add CaesarCipherList functionality in order to test printing the contents of the list. To ensure good design, do not include any printing inside the class itself.
output.txt
***** RUN 1: *****
test constructor : cc
Encrypted: ,IPPSnb[SVPHc
Decrypted: Hello, world!
test constructor : cc2
Encrypted: Dahhk(zsknh`{
Decrypted: Hello, world!
*** testing copy constructor and overloaded assignment ***
test copy constructor : cc3(cc2)
Encrypted: Dahhk(zsknh`{
Decrypted: Hello, world!
test overloaded assignment operator : cc2 = cc
Encrypted: ,IPPSnb[SVPHc
Decrypted: Hello, world!
*** printing cc, cc2, and cc3 ***
test constructor : cc
Encrypted: ,IPPSnb[SVPHc
Decrypted: Hello, world!
test constructor : cc2
Encrypted: ,IPPSnb[SVPHc
Decrypted: Hello, world!test constructor : cc3
Encrypted: Dahhk(zsknh`{
Decrypted: Hello, world!
*** testing comparators ***
test comparators
cc == c3 is false
cc < c3 is true
cc > c3 is false
*** testing add ***
test add : cc2 = cc + cc3
Encrypted: (ELLOj^WORLD_
Decrypted: Hello, world!
*** testing increment ***
test increment (postfix) : cc4 = cc++
Encrypted: -JQQToc\TWQId
Decrypted: Hello, world!
result of cc4 after postfix :
Encrypted: ,IPPSnb[SVPHcDecrypted: Hello, world!test increment (prefix) : cc4 = ++ccEncrypted: .KRRUpd]UXRJeDecrypted: Hello, world!result of cc4 after prefix :Encrypted: .KRRUpd]UXRJeDecrypted: Hello, world!test increment (postfix) : cc4 = cc2++Encrypted: )FMMPk_XPSME`Decrypted: Hello, world!result of cc4 after postfix :Encrypted: (ELLOj^WORLD_Decrypted: Hello, world!test increment (prefix) : cc4 = ++cc2Encrypted: *GNNQl`YQTNFaDecrypted: Hello, world!result of cc4 after prefix :Encrypted: *GNNQl`YQTNFaDecrypted: Hello, world!test increment (postfix) : cc4 = cc3++Encrypted: Ebiil){tloia|Decrypted: Hello, world!result of cc4 after postfix :Encrypted: Dahhk(zsknh`{Decrypted: Hello, world!test increment (prefix) : cc4 = ++cc3Encrypted: Fcjjm*|umpjb}Decrypted: Hello, world!result of cc4 after prefix :Encrypted: Fcjjm*|umpjb}Decrypted: Hello, world!----------------------------*** testing list ***test list : add cc, cc2, cc3test print list[0] :Encrypted: .KRRUpd]UXRJeDecrypted: Hello, world!test print list[1] :
Encrypted: *GNNQl`YQTNFaDecrypted: Hello, world!test print list[2] :Encrypted: Fcjjm*|umpjb}Decrypted: Hello, world!***** RUN 2: *****test constructor : ccEncrypted: Lipps0${svph%Decrypted: Hello, world!test constructor : cc2Encrypted: i(//2MA:25/'BDecrypted: Hello, world!*** testing copy constructor and overloaded assignment ***test copy constructor : cc3(cc2)Encrypted: i(//2MA:25/'BDecrypted: Hello, world!test overloaded assignment operator : cc2 = ccEncrypted: Lipps0${svph%Decrypted: Hello, world!*** printing cc, cc2, and cc3 ***test constructor : ccEncrypted: Lipps0${svph%Decrypted: Hello, world!test constructor : cc2Encrypted: Lipps0${svph%Decrypted: Hello, world!test constructor : cc3Encrypted: i(//2MA:25/'BDecrypted: Hello, world!*** testing comparators ***test comparatorscc == c3 is falsecc < c3 is truecc > c3 is false*** testing add ***test add : cc2 = cc + cc3Encrypted: m,336QE>693+FDecrypted: Hello, world!
*** testing increment ***test increment (postfix) : cc4 = cc++Encrypted: Mjqqt1%|twqi&Decrypted: Hello, world!result of cc4 after postfix :Encrypted: Lipps0${svph%Decrypted: Hello, world!test increment (prefix) : cc4 = ++ccEncrypted: Nkrru2&}uxrj'Decrypted: Hello, world!result of cc4 after prefix :Encrypted: Nkrru2&}uxrj'Decrypted: Hello, world!test increment (postfix) : cc4 = cc2++Encrypted: n-447RF?7:4,GDecrypted: Hello, world!result of cc4 after postfix :Encrypted: m,336QE>693+FDecrypted: Hello, world!test increment (prefix) : cc4 = ++cc2Encrypted: o.558SG@8;5-HDecrypted: Hello, world!result of cc4 after prefix :Encrypted: o.558SG@8;5-HDecrypted: Hello, world!test increment (postfix) : cc4 = cc3++Encrypted: j)003NB;360(CDecrypted: Hello, world!result of cc4 after postfix :Encrypted: i(//2MA:25/'BDecrypted: Hello, world!test increment (prefix) : cc4 = ++cc3Encrypted: k*114OC<471)DDecrypted: Hello, world!result of cc4 after prefix :Encrypted: k*114OC<471)DDecrypted: Hello, world!----------------------------*** testing list ***
test list : add cc, cc2, cc3
test print list[0] :
Encrypted: Nkrru2&}uxrj'
Decrypted: Hello, world!
test print list[1] :
Encrypted: o.558SG@8;5-H
Decrypted: Hello, world!
test print list[2] :
Encrypted: k*114OC<471)D
Decrypted: Hello, world!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
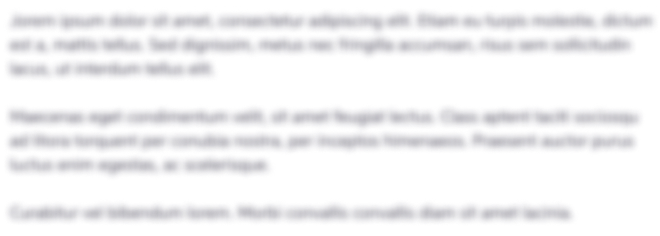
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started