Question
Description Write a program that reads from an input file a person's name followed by all their exam scores. Calculate and write the average score
Description
"Write a program that reads from an input file a person's name followed by all their exam scores. Calculate and write the average score for all students on each exam to an output file. Then, write each student's name to the output file followed by the student's score and grade on each exam."
Lab requirements:
Use command line argument #1 (argv[1]) to open the input file name.
Use command line argument #2 (argv[2]} to open the output file name.
Read and process student scores from the input file.
The first line of the input file has the number of students and exams.
Subsequent lines contain the student name followed by scores for each exam.
All students have the same number of exam scores.
Store the student names in a dynamic string array.
Store the exam scores in a two-dimensional dynamic double array (one row for each student, one column for each exam score, ie. # students x # of exams.)
Output the average score for each exam (rounded to one decimal place) followed by evenly spaced number of student grades for A's, B's, C's, D's, and E's. (List the letter grade in parentheses after the number of grades.)
If the student's score is within + or - 5 points of the average, give a grade of C.
If the grade is more than 5 points but less than 15 points above (or below) the average, give a grade of B (D).
If the grade is 15 points or more above (or below) the average, give a grade of A (E).
Output each student's exam grade in evenly spaced columns - the student score followed by their grade in parentheses.
Bonus requirements:
Calculate and output the average of all class exams (rounded to one decimal place).
Calculate and output average of each student's exam grades (rounded to one decimal place).
Helps and Hints
Note: Code examples come from readily available, public websites (ie., stackoverflow.com, cplusplus.com, etc.)
Parsing an Input Text File.(collapse)
if (argc < 3) { cerr << "Please provide name of input and output files"; return 1; } cout << "Input file: " << argv[1] << endl; ifstream in(argv[1]); if (!in) { cerr << "Unable to open " << argv[1] << " for input"; return 1; } cout << "Output file: " << argv[2] << endl; ofstream out(argv[2]); if (!out) { in.close(); cerr << "Unable to open " << argv[2] << " for output"; }
The function main() has two arguments, traditionally called argc and argv. Argc is of type int and is the number of parameters from a command line used in calling the function main. Argv is of type char** and is an array of C string pointers. Argv[0] points to the first parameter on a command line (which is the command), argv[1] points to the second, and so forth. (The size of argv is argc.)
Use argv[1] for the name of the input file and argv[2] for the name of the output file. The following is an example of how to open files for reading and writing:
To set command line arguments in Visual Studio, right click on project name and select Properties. Under the Configuration Properties, select Debugging and enter Command Arguments.
#include and place the following code temporarily at the beginning of main to find where to place argv[1] input files:
char full[_MAX_PATH]; if (_fullpath(full, ".\", _MAX_PATH)) cout << "Place input files here: " << full << endl; else cout << "Invalid path" << endl;
Reading from a file.(collapse)
Use extraction operator (">>") to read from the input stream:
int num_students; int num_exams; in >> num_students >> num_exams; // Skip the rest of the line in.ignore(std::numeric_limits::max(), ' ');
Use getline to read from the input stream:
string line; getline(in, line); size_t p = 0; while (!isdigit(line[p])) ++p; // line[p] is the first digit
Use istringstream to read from the input stream:
string line = line.substr(p); // Put this into an istringstream istringstream iss(line); iss >> scores;
Use IO manipulators fixed, setw, and setprecision (found in header ) to format output:
out << std::setw(20) << name << " "; out << std::fixed << std::setprecision(0) << std::setw(6) << scores;
C++ two-dimensional dynamic arrays.(collapse)
A dynamic 2D array is basically an array of pointers to arrays. You should initialize it using a loop, like this:
int rows = 100; int cols = 200; int **myArray = new int*[rows]; for(int i = 0; i < rows; ++i) { myArray[i] = new int[cols]; }
Since new is used in creating the dynamic array, all column arrays must be deleted followed by the row array to prevent memory leaks:
for(int i = 0; i < rows; ++i) { delete [] myArray[i]; } delete [] myArray;
Detecting Memory Leaks.(collapse)
Place the following code near the beginning of the file containing your main function:
#ifdef _MSC_VER #define _CRTDBG_MAP_ALLOC #include #define VS_MEM_CHECK _CrtSetDbgFlag(_CRTDBG_ALLOC_MEM_DF | _CRTDBG_LEAK_CHECK_DF); #else #define VS_MEM_CHECK #endif
Put the following C pre-processor macro at the beginning of your main code:
int main(int argc, char * argv[]) { VS_MEM_CHECK // enable memory leak check // Your program... return 0; }
If you get something like the following when your program exits, YOU HAVE A MEMORY LEAK! You are required to remove all memory leaks.
The thread 0x34bc has exited with code 0 (0x0). Detected memory leaks! Dumping objects -> {149} normal block at 0x00365008, 4000 bytes long. Data: < > 01 00 00 00 02 00 00 00 03 00 00 00 04 00 00 00 Object dump complete. The program '[4984] Lab 01 - grades.exe' has exited with code 0 (0x0).
lab01_in_01.txt
6 8 Cody Coder 84 100 100 70 100 80 100 65 Harry Houdini 77 68 65 100 96 100 86 100 Harry Potter 100 100 95 91 100 70 71 72 Mad Mulligun 88 96 100 90 93 100 100 100 George Washington 100 72 100 76 82 71 82 98 Abraham Lincoln 93 88 100 100 99 77 76 93
lab01_out_01.txt
Exam Averages: Exam 1 average =90.3 0(A) 2(B) 2(C) 2(D) 0(E) Exam 2 average =87.3 0(A) 3(B) 1(C) 0(D) 2(E) Exam 3 average =93.3 0(A) 4(B) 1(C) 0(D) 1(E) Exam 4 average =87.8 0(A) 2(B) 2(C) 1(D) 1(E) Exam 5 average =95.0 0(A) 0(B) 5(C) 1(D) 0(E) Exam 6 average =83.0 2(A) 0(B) 1(C) 3(D) 0(E) Exam 7 average =85.8 0(A) 2(B) 2(C) 2(D) 0(E) Exam 8 average =88.0 0(A) 3(B) 1(C) 0(D) 2(E) Student Exam Grades: Cody Coder 84(D) 100(B) 100(B) 70(E) 100(C) 80(C) 100(B) 65(E) Harry Houdini 77(D) 68(E) 65(E) 100(B) 96(C) 100(A) 86(C) 100(B) Harry Potter 100(B) 100(B) 95(C) 91(C) 100(C) 70(D) 71(D) 72(E) Mad Mulligun 88(C) 96(B) 100(B) 90(C) 93(C) 100(A) 100(B) 100(B) George Washington 100(B) 72(E) 100(B) 76(D) 82(D) 71(D) 82(C) 98(B) Abraham Lincoln 93(C) 88(C) 100(B) 100(B) 99(C) 77(D) 76(D) 93(C) **BONUS** Class Average = 88.8 Student Final Exam Grade: Cody Coder 87.4(C) Harry Houdini 86.5(C) Harry Potter 87.4(C) Mad Mulligun 95.9(B) George Washington 85.1(C) Abraham Lincoln 90.8(C)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
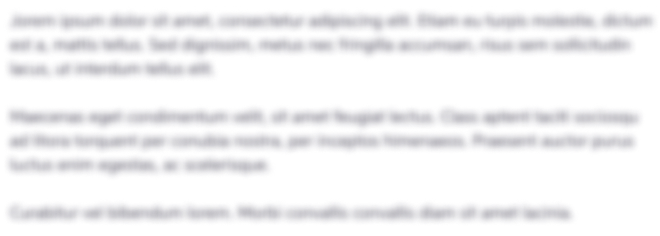
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started