Question
Design a network diagram in Java which can determine all paths in the network. I already have the code for it but I don't know
Design a network diagram in Java which can determine all paths in the network. I already have the code for it but I don't know how to implement the 3 java files into GUI frame. The program is supposed to take the input from the user in the GUI text box and then upon pressing the Calculate button, it should give process whatever is in the Node.java, NodeBuilder, and NetworkBuilder.java and show the output on the screen of the GUI. Right now since there is no GUI implemented in the program, so the user has to type "stop" to finish entering input - you can remove that functionality in NetworkBuilder since the user will then just press Calculate button. I just need to implement all these files ino GUI.
Node.java
import java.util.ArrayList; public class Node { private String activityName; private int duration; private ArrayListparents = new ArrayList (); private boolean end; public Node() { this.activityName = "ExampleActivity"; this.duration = 1; this.parents.add("null"); this.end = false; } public Node(String activityName, int duration, ArrayList parents) { this.activityName = activityName; this.duration = duration; this.parents = (ArrayList ) parents.clone(); this.end = false; } public String getActivityName() { return activityName; } public void setActivityName(String activityName) { this.activityName = activityName; } public int getDuration() { return duration; } public void setDuration(int duration) { this.duration = duration; } public ArrayList getParents() { return parents; } public void setParents(ArrayList parents) { for(int i=0;i nodes ){ for( Node node : nodes ) { if( node.parents.contains( this.activityName ) ) return; } this.end = true; } @Override public String toString() { return "Node [activityName=" + activityName + ", duration=" + duration + ", parents=" + parents + ", end=" + end + "]"; } }
NodeBuilder.java
import java.util.ArrayList; import java.util.Scanner; public class NodeBuilder { public static void main(String[] args) { //Initialize ArrayList to store created nodes ArrayListnodes = new ArrayList (); Scanner kb = new Scanner(System.in); // Initializing node variables String activityName; int duration; ArrayList parents = new ArrayList (); String input = ""; boolean stop = false; int i = 0; do { //Receive user node input System.out.println("Enter Node Information."); input = kb.nextLine(); //Setting up new Scanner and delimiter Scanner s = new Scanner(input); s.useDelimiter(","); //Set node variables with user input if(s.hasNext()) { activityName = s.next(); //System.out.println(activityName); } else activityName = "Activity " + i; if(s.hasNext()) { duration = s.nextInt(); //System.out.println(duration); } else duration = 0; while (s.hasNext()) { parents.add(s.next()); } if(!activityName.equals("stop")) { Node myNode = new Node(activityName, duration, parents); nodes.add(myNode); } else { stop = true; } //System.out.println(parents.size()); //System.out.println(nodes.size()); //reset parents ArrayList parents.clear(); //Closing Scanner s.close(); i += 1; } while (!stop); for(Node node : nodes) { node.setEnd( nodes ); } // for(int x=0;x NetworkBuilder.java
import java.util.ArrayList;
public class NetworkBuilder { // goal: static public void networkBuilder(ArrayListnodes) { ArrayList myStack = new ArrayList (); ArrayList starters = new ArrayList (); ArrayList enders = new ArrayList (); Node lastPopped = null; //Finding starting nodes for(Node node : nodes) { if(node.getParents().isEmpty()) { starters.add(node); } else if(node.getEnd()) { enders.add(node); } } //Building paths for(Node node : enders) { myStack.add(node); while(!myStack.isEmpty()) { //Getting the top myStack Node top = myStack.get( myStack.size()-1 ); //System.out.println("top:" + top.getActivityName()); //If the current top of myStack is a starter if( starters.contains( top ) ) { //Print stack int totalDuration = 0; for(int i = myStack.size()-1;i>=0;i--) { System.out.print(myStack.get(i).getActivityName() + ":" + myStack.get(i).getDuration() + " "); totalDuration += myStack.get(i).getDuration(); } System.out.print("Total Duration: " + totalDuration); System.out.println(); //Pop because we are the start lastPopped = top; myStack.remove(top); } else { String nextName = grabNext(top,lastPopped); //System.out.println("nextName:" + nextName); // Because at the end of the children if(nextName == null) { // Pop ourselves because no children lastPopped = top; myStack.remove( top ); } else { Node nextNode = getNodeFromName(nextName,nodes); myStack.add(nextNode); } } } } } static String grabNext(Node top, Node lastPopped) { if(lastPopped == null) { return top.getParents().get(0); } int index = top.getParents().indexOf(lastPopped.getActivityName()); if(index == top.getParents().size()-1) { return null; } return top.getParents().get(index+1); } static Node getNodeFromName( String name, ArrayList nodes ) { for( Node node : nodes ) { if( node.getActivityName().equals( name ) ) { return node; } } return null; } } DisplayGUI.java
//importing required classes
import java.awt.BorderLayout; import java.awt.Color; import java.awt.FlowLayout; import java.awt.GridLayout; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.util.Set; import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JOptionPane; import javax.swing.JPanel; import javax.swing.JTextArea; import javax.swing.JTextField; import javax.swing.JLabel;; //defining class, implementing listener to specify action for button public class DisplayGUI extends JFrame implements ActionListener{ //Declaring required component JTextField tf; JButton bt1,bt2,bt3,bt4,bt5,bt6; JTextArea ta1; JPanel pl1,pl2,pl3,pl4,pl5; JLabel lb1; //defining the constructor public DisplayGUI(){ //initializing the component tf=new JTextField(20); ta1=new JTextArea(25,20); bt1=new JButton("About"); bt2=new JButton("Help"); lb1 = new JLabel("Network Path Analyzer"); bt4=new JButton("X"); bt5=new JButton("Calculate"); bt6=new JButton("Reset"); bt4.setBackground(Color.red); pl1=new JPanel(); pl4 = new JPanel(); pl5 = new JPanel(); pl1.setLayout(new FlowLayout(FlowLayout.LEFT, 10, 10)); pl5.setLayout(new FlowLayout(FlowLayout.LEFT, 110, 10)); pl2=new JPanel(); pl3=new JPanel(); //adding the component to panel pl4.add(bt1); pl4.add(bt2); pl1.add(pl4); pl5.add(lb1); pl5.add(bt4); pl1.add(pl5); pl2.setLayout(new GridLayout(2,1)); pl2.add(bt5); pl2.add(bt6); pl2.add(tf); pl3.add(pl2); pl3.add(tf); //setting the GUI layout and adding the component setLayout(new BorderLayout()); add(pl1,BorderLayout.NORTH); add(pl3,BorderLayout.SOUTH); add(ta1,BorderLayout.CENTER); //adding the listener to the button bt1.addActionListener(this); bt2.addActionListener(this); bt4.addActionListener(this); bt5.addActionListener(this); bt6.addActionListener(this); } //defining the main function public static void main(String[] args) { //Creating an object of the class DisplayGUI ob=new DisplayGUI(); //setting the size of the GUI ob.setTitle("Network Path Analyzer"); ob.setSize(700,500); //making it visible ob.setVisible(true); //adding the exit function for closing the GUI ob.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } //function to perform action according to the buttons @Override public void actionPerformed(ActionEvent ae) { //getting the component who created the action String str=ae.getActionCommand(); //if about button is clicked if(str.equals("About")){ //showing the message to the user JOptionPane.showMessageDialog(bt1, "This program allows the user to create nodes of a desired network path by inputting the activity name, duration and a list of predecessors"); } //if the help button is clicked else if(str.equals("Help")){ //showing message to the user JOptionPane.showMessageDialog(bt1, "To compute the Network, type Activity name(multiple characters),Duration(integer),Dependencies(predecessors). Then press Calculate. If an error is displayed, click on " + " and enter again. To close the screen, click on X on top right corner."); } else if(str.equals("Project Title")){ } //if calculate button is clicked add the text to the text area else if(str.equals("Calculate")){ ta1.setText(tf.getText()); } //if user want to exit else if(str.equals("X")){ System.exit(1); } //resetting the text area else{ ta1.setText(""); tf.setText(""); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
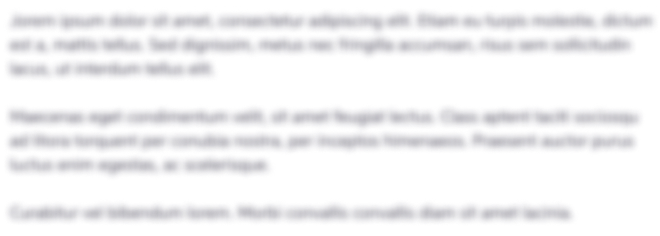
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started