Question
Design a program named NameListSorted.java, which implements a list structure with linked list node (LLNode.java). Your NameListSorted ADT should have the following features: add(Name name),
Design a program named NameListSorted.java, which implements a list structure with linked list node (LLNode.java). Your NameListSorted ADT should have the following features: add(Name name), size(), isEmpty, isFull, insert(Name name) (does it make sense to you?) , search(Name name) - returns index of the object(the first occurrence) in the list, returns -1 if not found, get(index) - returns the Name object(not LLNode) in the specified index location, remove(index), remove(Name name) -remove the all occurrences of the object(content), removeAll(), print(String prefix)-print all objects start with "prefix" print() - print all names, one name per line. print(startingIndex) - print all names starting from the startingIndex(inclusive) print(startingIndex, endingIndex) - print all names starting from the startingIndex(inclusive) to the endingIndex (exclusive);
sublist(startingIndex, endingIndex) - returns a list(NameListSorted) containing all elements (names) from the startingIndex(inclusive) to the endingIndex(exclusive). if startingIndex<0, then start from the first element; if endingIndex>= the total number of elements, then the list should include the last element.
build(File file) - build the sorted list from the file. The first line in the data file indicates the number (N) of names in the file, followed by N lines, each line represents a name: firstName lastName. the method header: public void build(File file).
- Your list is sorted based on alphabetic order of their last name, then first name
- print()-print all names in the list
- print(String predix)-print all names whose last name starts with prefix
Write a driver program named NameListDriver.java that reads names from the given data file, construct a list, then test your methods. Finally, run Grader.class to grade your code.
Click to down files for this assignment
Submission requirements:
screenshot both java code and running result (Grader.class) then upload them here. Name.txt:
public class Name implements Comparable
private String firstName; private String lastName; public Name(){ //todo for non-parameter constructor } public Name(String firstName, String lastName){ setFirstName(firstName); setLastName(lastName); }
public String getFirstName(){ return firstName; } public String getLastName(){ return lastName; } public void setFirstName(String firstName){ this.firstName = firstName; } public void setLastName(String lastName){ this.lastName = lastName; } public int compareTo(Name n){ if(lastName.compareTo(n.getLastName())==0){ return firstName.compareTo(n.getFirstName()); } return lastName.compareTo(n.getLastName()); } public String toString(){ return firstName+" "+lastName; } } LLNode.txt:
public class LLNode{
private Name name; private LLNode next; public LLNode(){ name=null; next=null; } public LLNode(Name name, LLNode next){ setName(name); setNext(next); }
public Name getName(){ return name; } public LLNode getNext(){ return next; } public void setName(Name name){ this.name = name; } public void setNext(LLNode next){ this.next = next; }
} Grader:
import java.io.File; public class Grader { public Grader() { } public static void main(String[] var0) { int var1 = 0; int var2 = 0; byte var3 = 22; try { NameListSorted var4 = new NameListSorted(); System.out.println("A new list added names[B,B],[A, A], [D,D]"); var4.add(new Name("B", "B")); var4.add(new Name("A", "A")); var4.add(new Name("D", "D")); var4.print(); int var5 = var4.size(); if (var5 == 3) { System.out.println("The size is 3-correct!"); ++var2; } else { System.out.println("The size should be 3, but yours returns " + var5); ++var1; } System.out.println(""); Name var6 = new Name("B", "B"); int var7 = var4.search(var6); if (var7 == 1) { System.out.println("search name[B,B] returns 1-corrrect."); ++var2; } else { System.out.println("search name[B,B] should return 1, but yours returns " + var7 + " - Wrong!"); ++var1; } System.out.println("after removing [B,B]"); var4.remove(var6); var4.print(); var5 = var4.size(); if (var5 == 2) { System.out.println("The size now is 2-correct."); ++var2; } else { System.out.println("The size now should be 2, but yours return " + var5 + "-Wrong!"); ++var1; } System.out.println("After adding [D,D], [A,A],[D,D]"); Name var8 = new Name("A", "A"); Name var9 = new Name("D", "D"); Name var10 = new Name("D", "D"); var4.add(var10); var4.add(var8); var4.add(var9); var4.print(); var5 = var4.size(); if (var5 == 5) { System.out.println("The size now is 5-correct."); ++var2; } else { System.out.println("The size now should be 2, but yours return " + var5 + "-Wrong!"); ++var1; } var7 = var4.search(var6); if (var7 == -1) { System.out.println("search name[B,B] returns -1-corrrect."); ++var2; } else { System.out.println("search name[B,B] should return -1, but yours returns " + var7 + " - Wrong!"); ++var1; } var7 = var4.search(var10); if (var7 == 2) { System.out.println("search name[D,D] returns 2-corrrect."); ++var2; } else { System.out.println("search name[D,D] should return 2, but yours returns " + var7 + " - Wrong!"); ++var1; } System.out.println("After remove[D,D]"); var4.remove(var9); var4.print(); var7 = var4.search(var10); if (var7 == -1) { System.out.println("search name[D,D] returns -1 -corrrect."); ++var2; } else { System.out.println("search name[D,D] should return -1, but yours returns " + var7 + " - Wrong!"); ++var1; } System.out.println("After removing [A,A]"); var4.remove(var8); var4.print(); if (var4.isEmpty() && var4.size() == 0) { System.out.println("Now the list is empty and size=0 - Correct!"); ++var2; } else { System.out.println("Your list is either not empty or the size method did not return 0. Wrong!"); ++var1; } System.out.println("After adding [D,D], [A,A],[D,D],[B,B]"); var4.add(var9); var4.add(var8); var4.add(var10); var4.add(var6); var4.print(); var7 = var4.search(var6); if (var7 == 1) { System.out.println("search name[B,B] returns 1-corrrect."); ++var2; } else { System.out.println("search name[B,B] should return 1, but yours returns " + var7 + " - Wrong!"); ++var1; } System.out.println("afer calling removeAll():"); var4.removeAll(); var4.print(); if (var4.isEmpty() && var4.size() == 0) { System.out.println("Now the list is empty and size=0 - Correct!"); ++var2; } else { System.out.println("Your list is either not empty or the size method did not return 0. Wrong!"); ++var1; } System.out.println("adding [D,D], [A,A],[D,D],[B,B]"); var4.add(var9); var4.add(var8); var4.add(var10); var4.add(var6); var4.print(); System.out.println("Create a sublist by calling sublist(1, 50)"); NameListSorted var11 = var4.sublist(1, 50); System.out.println("***************THe sublist:************"); var11.print(); var7 = var11.search(var6); if (var7 == 0) { System.out.println("search name[B,B] in the sublist returns 0-corrrect."); ++var2; } else { System.out.println("search name[B,B] in the sublist should return 0, but yours returns " + var7 + " - Wrong!"); ++var1; } Name var12 = new Name("C", "C"); var4.add(var12); System.out.println("Now add[C,C] to original list, NOT the sublist! THs sublist should remain the same."); var5 = var11.size(); if (var5 == 3) { System.out.println("The size of the sublist is 3-correct."); ++var2; } else { System.out.println("The size of the sublist should be 3, but yours return " + var5 + "-Wrong!"); ++var1; } System.out.println("For reference, here is your original list:"); var4.print(); var4 = new NameListSorted(); System.out.println(" ========================= Create a new list."); System.out.println("build the list from file by calling build(file) method"); var4.build(new File(var0[0])); var5 = var4.size(); if (var5 == 434) { System.out.println("The size of the new list is 434-correct."); ++var2; } else { System.out.println("The size of the new list should be 434, but yours return " + var5 + "-Wrong!"); ++var1; } System.out.println("print by calling print(10, 20)"); var4.print(10, 20); int[] var13 = new int[]{0, 20, 50, 100, 200, 500}; String[] var14 = new String[]{"Kaitlyn Abbott", "Averi Barajas", "Yaretzi Booth", "Carla Cooke", "Conrad Howe", null}; for(int var15 = 0; var15 < var13.length; ++var15) { Name var16; if (var14[var15] == null) { var16 = var4.get(var13[var15]); System.out.print("calling get(" + var13[var15] + ") should return null. Yours returned " + var16); if (var16 == null) { ++var2; System.out.println(". Correct"); } else { ++var1; System.out.println(". Wrong!"); } } else { var16 = var4.get(var13[var15]); System.out.print("calling get(" + var13[var15] + ") should return " + var14[var15] + ". Yours returned " + var16); if (var14[var15].equals(var16.toString())) { ++var2; System.out.println(". Correct"); } else { ++var1; System.out.println(". Wrong!"); } } } Name var18 = new Name("Savanna", "Anthony"); var7 = var4.search(var18); if (var7 == 8) { System.out.println("search name [Savanna, Anthony] in the list returns 8-corrrect."); ++var2; } else { System.out.println("search name [Savanna, Anthony] in the list should return 8, but yours returns " + var7 + " - Wrong!"); ++var1; } System.out.println("Create a sublist by calling sublist(10, 20)"); var11 = var4.sublist(10, 20); System.out.println("***************THe sublist:************"); var11.print(); var4.removeAll(); System.out.println("remove all from list-not sublist!"); var5 = var11.size(); if (var5 == 10) { System.out.println("The size of the sublist is 10-correct."); ++var2; } else { System.out.println("The size of the sublist should be 10, but yours return " + var5 + "-Wrong!"); ++var1; } var18 = new Name("Carlie", "Baker"); var7 = var11.search(var18); if (var7 == 8) { System.out.println("search name [Carlie, Baker] in the sublist returns 8-corrrect."); ++var2; } else { System.out.println("search name [Carlie, Baker] in the sublist should return 8, but yours returns " + var7 + " - Wrong!"); ++var1; } } catch (Error | Exception var17) { System.out.println(var17); } System.out.println("Total testing cases: " + var3 + ". I got " + var2 + " correct"); System.out.println("My Grade: " + var2 + " out of " + var3); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
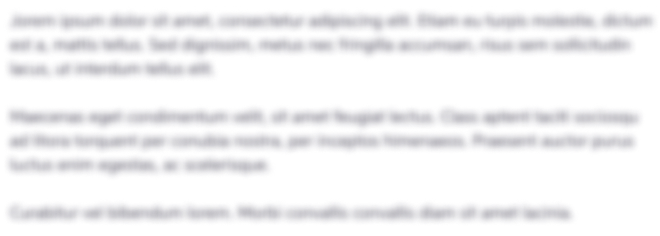
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started