Question
Design a program to manage and display data about passengers in the historic Titanic disaster, using the Passenger class should be used to represent each
Design a program to manage and display data about passengers in the historic Titanic disaster, using the Passenger class should be used to represent each passenger's data as represented in the given dataset found on Canvas called titanic. Edit the test to remove the documentation and explanation of what the file is that appears at the beginning. Exception handling must include recovering from potentially bad data in the input file. The collection of Passengers should be implemented as an ArrayList that once all the passengers are read in and added to the ArrayList you can loop through the ArrayList and generate statistics. The program consists of several classes working together as described below.
Preparation and approach
Plan to proceed incrementally, by first implementing the Passenger without any exception handling. Test them using the TitanicTester client (code given below). Next, add Exception handling and test using TitanicTester2 client (also given below). Finally, create your own client (Titanic) that uses data from the actual dataset; be sure to again use an incremental approach, implementing first the input from the file and getting that to work before proceeding to the remaining steps of displaying information about the data; leave writing the output file for last, after all the other parts are working correctly.
size=3 width="100%" align=center>
Classes
1) Passenger: Represents a passenger of the Titanic, with attributes (instance variables):
Instance data:
status (an integer: 1, 2, 3, or 4, representing 1st, 2nd, 3rd class or crew
child (a boolean: true = child, false = adult)
sex (a String: male or female)
survivor (a boolean: true/false indicating whether this passenger survived)
Constructors:
a constructor that has parameters for all the instance data
Later we will modify this to throw and exception - should throw an OutOfRangeException if any of the values cannot be used for the instance data (e.g., status = 5 or something else is not usable as instance data))
Methods:
accessors and mutators for all the instance data
toString() method
Hints:
* Note that you need to obtain the data in the correct format, so that the Passenger constructor gets the correct values and in the correct order for the instance variables.
3) OutOfRangeException: Code provided below. Use it in the constructor of the Passenger class to throw OutOfRangeException when the status is not in the range 1-4; or when sex is not "male" or "female" (yes, the world encoded in the Titanic dataset is rather binary in that way- not necessary anymore)
//********************************************************************
// OutOfRangeException.java
// Represents an exceptional condition in which a value is out of
// some particular range.
//********************************************************************
public class OutOfRangeException extends Exception
{
//-----------------------------------------------------------------
// Sets up the exception object with a particular message.
//-----------------------------------------------------------------
OutOfRangeException(String message)
{
super(message);
}
}
Clients
1) TitanicTester: Code provided below. This is a test client for Passenger class. Use it to ensure that the class is implemented correctly before proceeding to do exception handling with erroneous input or file input.
public class TitanicTester
{
public static void main(String[] args)
{
// create some Passenger objects
Passenger p1 = new Passenger (4, false, "male", false);
Passenger p1 = new Passenger (2, true, "female", false);
// Print out their info
System.out.println(p1);
System.out.println(p2);
ArrayList
titanic.addPassenger (3, true male false);
titanic.addPassenger (1, true, female true);
titanic.addPassenger (3, false, female ,false);
// Print info about collection (should be 3 passengers, 1 survivor)
System.out.println(titanic);
}
}
2) TitanicTester2: Cod.e provided below. Another test client for Passenger to test your exception handling. JUST CONSIDER THE EXCEPTIONS BELOW. Use it
to ensure that these classes are implemented correctly before proceeding to do file input.
public class TitanicTester2
{
public static void main(String[] args)
{ ArrayList
titanic.addPassenger (2 ,male, true, false); // exception - "male" in the wrong place - THIS EXCEPTION IS EXTRA CREDIT _ NOT NEEDED
titanic.addPassenger (5, true, male, false);
// exception - status cannot be 5
//Loop through and print out titanic }
}
3) Titanic: The client that processes the data file (see first paragraph in the assignment) (the actual survival data from the Titanic) and produces a file in a different format.
- Set
- up to scan from the input file.
- Scan
- lines from the input file, make a Passenger object and add that Passenger to the ArrayList. Repeat until all the data has been read
- Loop
- through and compute meaningful statistics
- Survivor
- Info
% children %adult
%male %female
Step by Step Solution
There are 3 Steps involved in it
Step: 1
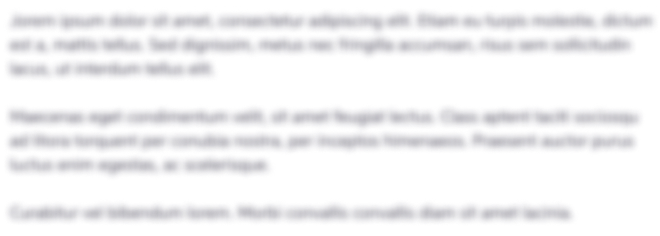
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started