Question
Design an abstract class named BankAccount to hold the following data for a bank account: -Account Name & Number -Balance -Number of deposits this month
Design an abstract class named BankAccount to hold the following data for a bank account:
-Account Name & Number -Balance -Number of deposits this month -Number of withdrawals -Annual interest rate -Monthly service charges
The class should have the following methods:
Constructor: The constructor should accept arguments for the balance and annual interest rate.
Deposit: A method that accepts an argument for the amount of the deposit. The method should add the argument to the account balance. It should also increment the variable holding the number of deposits.
Withdraw: A method that accepts an argument for the amount of the withdrawal. The method should subtract the argument from the balance. It should also increment the variable holding the number of withdrawals.
calcInterest: A method that updates the balance by calculating the monthly interest earned by the account, and adding this interest to the balance. This is performed by the following formulas:
Monthly Interest Rate = (Annual Interest Rate / 12) Monthly Interest = Balance * Monthly Interest Rate Balance = Balance + Monthly Interest
monthlyProcess: A method that subtracts the monthly service charges from the bal- ance, calls the calcInterest method, and then sets the variables that hold the number of withdrawals, number of deposits, and monthly service charges to zero.
Next, design a SavingsAccount class that extends the BankAccount class. The SavingsAccount class should have a status field to represent an active or inactive account. If the balance of a savings account falls below $25, it becomes inactive. (The status field could be a boolean variable.) No more withdrawals can be made until the balance is raised above $25, at which time the account becomes active again. The savings account class should have the following methods:
withdraw: A method that determines whether the account is inactive before a withdrawal is made. (No withdrawal will be allowed if the account is not active.) A withdrawal is then made by calling the superclass version of the method.
deposit: A method that determines whether the account is inactive before a deposit is made. If the account is inactive and the deposit brings the balance above $25, the account becomes active again. The deposit is then made by calling the superclass version of the method.
monthlyProcess: Before the superclass method is called, this method checks the number of withdrawals. If the number of withdrawals for the month is more than 4, a service charge of $1 for each withdrawal above 4 is added to the superclass field that holds the monthly service charges.
Mark the monthlyProcess method abstract in the BankAccount class
I have the following code done . I'm not sure how I can use the abstract class in bankaccount and call it in the savingsaccount.
public abstract class BankAccounts { protected double balance; //variable for balance protected double annualInterest; //variable for interest rates protected double serviceCharges; //variable for extra charges protected int num_deposits; //variable for deposits protected int num_withdraws; //variable for withdrawals public BankAccounts(double balance, double annualInterest) { this.balance = balance; //assigns value of balance to the field balance this.annualInterest = annualInterest; //assigns value of interest to the field annualinterest this.serviceCharges = 0; //initial service charges this.num_deposits = 0; //initial deposits this.num_withdraws = 0; //inital withdrawals } public void deposit(double amount) { balance += amount; //deposits amount num_deposits++; //number of deposits goes up } public void withdraw(double amount) { balance -= amount; //withdraws amount num_withdraws++; //number of withdrawals goes up } public void calculateInterest() { double monthlyInterestRate = (annualInterest/12); //finds monthly interest double monthlyInterest = this.balance * monthlyInterestRate; //finds monthly interest rate balance += monthlyInterest; //monthly balance after interest } public abstract void monthlyProcess(); public double getBalance() { return balance; //gets balance } public void setBalance() { this.balance = balance; //sets balance } public double getServiceCharges() { return serviceCharges; //gets service charge } public void setServiceCharges(double serviceCharges) { this. serviceCharges = serviceCharges; //sets service charge } public int getNum_deposits() { return num_deposits; //return number of deposits } public void setNum_deposits(int num_deposits) { this.num_deposits = num_deposits; //sets number of deposits } public int getNum_withdraws() { return num_withdraws; //gets number of withdrawls } public void setNum_withdraws(int num_withdraws) { this.num_withdraws = num_withdraws; //sets number of withdrawals } public double getAnnualInterest() { return annualInterest; //gets interest rate } public void setAnnualInterest(double annualInterest) { this.annualInterest = annualInterest; //set interest rate } } public class SavingsAccounts extends BankAccounts { private boolean status; //account status public SavingsAccounts(double balance, double annualInterest) { super( balance, annualInterest); if( balance >= 25) //if balance is greater or equal to $25 { status = true; //status is true } else { status = false; //status is false } } public void withdraw( double amount) { if(status) { super.withdraw(amount); //allows withdrawals if true status if(super.getBalance()<25) //if less than $25 status = false; //status is false } else { System.out.println("Cannot complete withdrawal. Account is inactive"); //if under $25 cannot withdraw } } public void deposit(double amount) { if(!status) { double available = super.getBalance() + amount; //balance after deposit if(available >= 25) //if balance after deposit is greater than $25 { status = true; //status is true } } super.deposit(amount); //return deposit } public void monthlyProcess() { int countWithdraws = super.getNum_withdraws(); //gets number of withdrawals if(countWithdraws > 4) //if withdrawals are greater than 4 { super.setServiceCharges(countWithdraws - 4); //sets service charge super.monthlyProcess(); //calls monthly process method if(super.getBalance()<25) //if balance is less than 25 after service charge status = false; //status is false } } public boolean isStatus() { return status; //gets status } public void setStatus(boolean status) { this.status = status; //sets status } } public class mainmethod { public static void main(String args[]) { SavingsAccounts sa = new SavingsAccounts(24,1.5); //new objects for initial balances and interst rates System.out.println("Initial account balance: $" + sa.getBalance()); //display initial balance printStatus(sa.isStatus()); //display status of account System.out.println(" Withdraw $22"); //display withdrawal sa.withdraw(22.00); //withdraws $22 System.out.println("Balance after withdrawal: $" + sa.getBalance()); //display results of withdrawal printStatus(sa.isStatus());//display status of account System.out.println(" Deposit $1"); //display deposit sa.deposit(1.00); //deposits $1 System.out.println("Balance after deposit: $" + sa.getBalance()); //display results of deposit printStatus(sa.isStatus());//display status of account sa.monthlyProcess(); //calls monthlyprocess method System.out.println(" Balance at the end of the month: $" + sa.getBalance()); //display end of month balance printStatus(sa.isStatus()); //display status of account System.out.println("Number of deposits made:" + sa.getNum_deposits()); //display amount of deposits System.out.println("Number of withdrawals made:" + sa.getNum_withdraws()); //display amount of withdrawals System.out.println("Monthly charges: " + sa.getServiceCharges()); //display applicable service charges } public static void printStatus(boolean status) { if(status) System.out.println("Account is active."); //display active account else System.out.println("Account is inactive."); //display inactive account } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
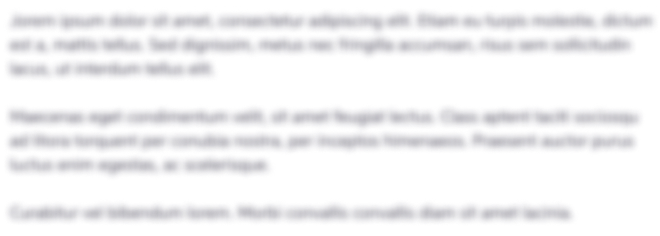
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started