Question
Design and code a class named Weather that holds information about weather conditions. Place your class definition in a header file named Weather.h and your
Design and code a class named Weather that holds information about weather conditions. Place your class definition in a header file named Weather.h and your function definitions in an implementation file named Weather.cpp. Include in your coding all of the statements necessary for your code to compile under a standard C++ compiler. The class Weather has the following three member data: A 7-character long C-style string (8 characters including the NULL byte) holding the calendar date associated with the weather information. The date is formatted as follows: (Jan/21, Apr/1, ...) A double value, representing the high temperature on the specified date A double value, representing the low temperature on the specified date Your design includes the following two member functions: void setData(const char*, double, double) - a modifier that stores data in a Weather object. The first parameter receives the address of a C-style string that holds the calendar date. The second and third parameters receive the low temperature and high temperatures respectively. void display() const - a query that displays the high and low temperature as well as the associated calendar date in the following format: o date left justified in a field of 10 using underscore '_' instead of white space o high and low temperatures right justified in fields of 6 with 1 decimal place each using underscore '_' instead of white space [Hint: use the reference example in the formatting notes to find the right manipulators.] [Hint: setw(int) only affects the next item sent to the stream.] Complete the following implementation file for the main module. The missing regions of code are in bold.
#include #include "Weather.h" using namespace std; int main() { int i; // loop counter int n; // number of objects in Weather array // declare a pointer named weatherArray of type Weather here cout << "Weather Data" << endl << "=====================" << endl << "Days of Weather: "; cin >> n; cin.ignore(); // allocate dynamic memory for weatherArray here for(i = 0; i < n; i++) { char dateDescription[7]; double high; double low; // add code to accept the user input for the weather array and set the data // for each object of weatherArray //[Hint] get() and ignore() } cout << endl << "Weather report:" << endl << "Date high low" << endl << "======================" << endl; for(i = 0; i < n; i++) { weatherArray[i].display(); } // deallocate dynamic memory here return 0; } Weather.h #ifndef WEATHER_H_ #define WEATHER_H_ #define MAX_DATE 7 class Weather { char date[MAX_DATE + 1]; double high; double low; public: void setData(const char*, double, double); void display() const; }; #endif Weather.cpp #define _CTR_SECURE_NO_WARNINGS #include #include #include #include "Weather.h" using namespace std; void Weather::setData(const char* d, double lo, double hi) { high = hi; low = lo; strncpy_s(date, d,6); } void Weather::display() const { cout << setw(10) << setfill('_') << left << date; cout.unsetf(ios::left); cout << fixed << setprecision(1) << setw(6) << high << setw(6) << low << endl; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
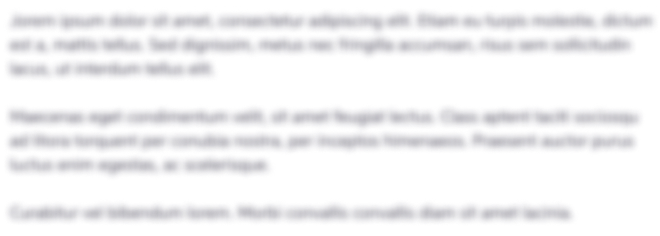
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started