Question
In this task you need to implement an unsorted list for StudentInfo class. Completing the following sub-tasks will help you to solve this task easily.
In this task you need to implement an unsorted list for "StudentInfo" class. Completing the following sub-tasks will help you to solve this task easily. a. Create "StudentInfo" class that has "Name", "ID" and "CGPA" as private properties. It should have constructor function(s) and any other necessary functions that are needed to modify and access the properties. You should also implement a "Print" function that prints the values of the properties of a "StudentInfo" object. b. Now modify the implementation of unsorted list that is available in google classroom (you may choose to implement from scratch if you want) so that it is no longer a class template. Instead it should work only with "StudentInfo" objects. c. It would be convenient to be able to search for a student with his/her ID. Therefore, you should implement the delete and retrieve function in such a way that they can operate with student ID as their function parameter. Now to test your data structure, create a few "StudentInfo" object in the driver file (main.cpp), insert them in a list and finally print the list.
#ifndef UNSORTEDLIST_H_INCLUDED
#define UNSORTEDLIST_H_INCLUDED
const int MAX_ITEMS = 10;
template
class UnsortedList
{
public:
UnsortedList();
void MakeEmpty();
bool IsFull();
int LengthIs();
bool InsertItem(ItemType);
bool DeleteItem(ItemType);
bool RetrieveItem(ItemType&);
bool GetNextItem(ItemType&);
void ResetList();
private:
int length;
ItemType info[MAX_ITEMS];
int currentPos;
};
#include "UnsortedList.tpp"
#endif //UNSORTEDLIST_H_INCLUDED
#include "UnsortedList.h"
template
UnsortedList
{
length = 0;
currentPos = -1;
}
template
void UnsortedList
{
length = 0;
currentPos = -1;
}
template
bool UnsortedList
{
return (length == MAX_ITEMS);
}
template
int UnsortedList
{
return length;
}
template
bool UnsortedList
{
if(!IsFull()) // if(IsFull()==false)
{
info[length] = item;
length++;
return true;
}
return false;
}
template
bool UnsortedList
{
int flag = 0;
int location = 0;
while (location < length )
{
if(item == info[location])
{
flag = 1;
break;
}
location++;
}
if(flag==1)
{
info[location] = info[length - 1];
length--;
return true;
}
return false;
}
template
bool UnsortedList
{
int location = 0;
while (location < length)
{
if(item == info[location])
{
item = info[location];
return true;
}
location++;
}
return false;
}
template
bool UnsortedList
{
if(currentPos { currentPos++; item = info [currentPos]; return true; } return false; } template void UnsortedList { currentPos = -1; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
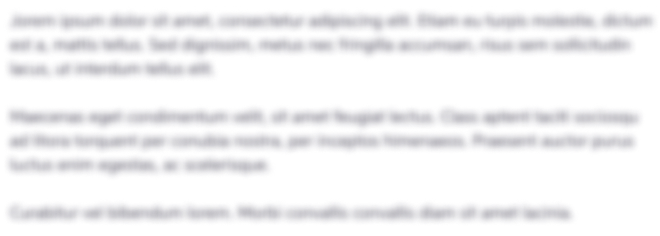
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started