Question
design and implement a C++ class which can be used to represent and manipulate simple integer vectors. You must use dynamic memory allocation to do
design and implement a C++ class which can be used to represent and manipulate simple integer vectors. You must use dynamic memory allocation to do this; you may not use standard vectors.
Define a class called intVector which handles all of the operations illustrated in the main function below:
#include
using namespace std;
//your definition of intVector here
int main()
{
int n;
cout << "Enter the size of intVector a: "; cin >> n;
// Create a new intVector with n elements, all zero
intVector a(n);
// Create a new intVector with 0 elements
intVector b;
// Print out some information about the size of this intVector:
cout << "Vector a has " << a.size() << " elements." << endl;
cout << "Vector b has " << b.size() << " elements." << endl;
// Print out the contents of intVector a (should be all zeroes!):
for(int r = 0; r < a.size(); r++)
{
cout << "a[" << r <<"] = " << a.get(r) << endl;
}
// Fill in a few values
// if a.size < index, the intVector is resized with intervening elements = 0
a.set(5, -5280); // 6th element is now -5280, elements 1-5 are 0
a.set(0, 123); // 1st element is now 123
// Create an identical copy of this intVector
intVector c(a); //the copy constructor is called here
// Assign intVector a to existing intVector b;
//don't implement an assignment operator. This should use the default assignment operator.
b = a;
// Change the original intVector some more
a.set(2, 555); // 3rd element is now 555
// Examine some elements of each intVector
cout << "a[0] = " << a.get(0) << " [should be 123]" << endl;
cout << "b[0] = " << b.get(0) << " [should be 123]" << endl;
cout << "c[0] = " << c.get(0) << " [should be 123]" << endl;
cout << "a[2] = " << a.get(2) << " [should be 555]" << endl;
cout << "b[2] = " << b.get(2) << " [should be 0, but will be 555 due to shallow copy]" << endl;
cout << "c[2] = " << c.get(2) << " [should be 0]" << endl;
return 0;
}
//your implementation of intVector member functions here
Step by Step Solution
There are 3 Steps involved in it
Step: 1
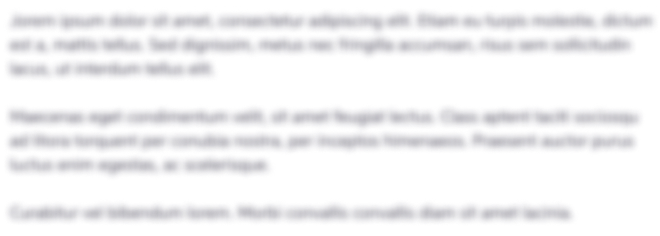
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started