Question
Design and implement a Player class that manages the songs on a music player device. The following class has been provided for you (do not
Design and implement a Player class that manages the songs on a music player device.
The following class has been provided for you (do not implement this class, the code is below. You should use this class to represent time values in your Player class.
TimeStamp:
The TimeStamp class stores an amount of time in seconds.
These operations are provided for a TimeStamp:
Two constructors:
one that takes an integer number of seconds as the argument.
The other takes one argument, a string containing the time value in
this format: mm:ss where mm is the minutes and ss is the seconds. The number of minutes must have at least one digit but may have more.
toSeconds() returns the time as a total number of seconds.
toString() returns the time as a string in the mm:ss format.
add(string) takes a string in the mm:ss format and adds the time represented by that string to the stored time.
subtract(string) takes a string in the mm:ss format and subtracts the time represented by that string from the stored time.
bool greaterThan(string): takes a string in the mm:ss format and returns true if the stored time is greater (later) than the time represented by the argument string.
Download the files TimeStamp.h and TimeStamp.cpp and incorporate them into your final program. Do not change these files and do not submit them.
Use the TimeStamp class as the data type for the length of the Songs described on the next page, as well as for the total length of all of the Songs stored on the Player.
Player:
You should implement the operations listed below for the Player class. You should use the function descriptions to determine what the member variables should be. However, you must declare a Song struct to store the data for a single song (make it a private member), and you must have an array of Song structures as a member variable.
Player(string name, float size) a constructor for the class.
name' is a name for it
'size' is a maximum capacity in Mb.
addSong(string band, string title, string length, float size) a function for adding a new song.
'band' is a name of the band
'title' is a name of the song
'length' is a time length of that song in a '1:23' format (mm:ss")
'size' is a size of the song in Mb
Return true if the song was added and false if there was not enough space (memory) for it or there was a song with the same band and title already in that device or if the device already has 1000 songs in it.
removeSong(string band, string title) a function for removing a song
'band' is a name of the band
'title' is a name of the song
Return true if the song was successfully removed and false if it wasn't present on the device.
displaySongInfo(string band, string title) a function to display a songs info in the following format:
Band:
Title:
Length:
Size:
'band' is a name of the band
'title' is a name of the song
The output must line up in two columns, but the labels may be right justified.
displaySongsByLength() a function to display songs sorted by their time length.
deviceInfo() a function to display info about the device in the following format:
Name:
Number of songs:
Total length:
Free space left:
The output must line up in two columns, but the labels may be right justified.
Input/Output:
Driver.cpp (provided below) contains code that you can use to test the Player class (the expected output will be in a comment at the end of the driver file). You do not need to write a main function for the program.
NOTES:
This program should be done in a Linux or Unix environment, using a command line compiler like g++. Do not use codeblocks, eclipse, or Xcode to compile.
Do removeSong last!!
Create a Makefile to compile and test the Player class. There will be four goals in this makefile, because you will have three .cpp files. Use the makefile from the timedemo example and modify it to work with these new files. Use the following names for your files:
TimeStamp.h
TimeStamp.cpp
Player.h
Player.cpp
Driver.cpp
Driver.cpp:
#include
using namespace std;
#include "Player.h"
using namespace std;
int main() {
Player ipod("iPod touch", 32.0);
ipod.addSong("Pink Floyd", "Hey You", "4:41", 10.82);
// expecting "Song is already present."
ipod.addSong("Pink Floyd", "Hey You", "4:41", 10.82);
ipod.addSong("The Doors", "Light My Fire", "7:06", 15.90);
// expecting "Not enough memory."
ipod.addSong("The Rolling Stones", "Gimme Shelter", "4:30", 10.33);
ipod.addSong("The Rolling Stones", "Gimme Shelter", "4:30", 5.27);
ipod.displaySongInfo("Pink Floyd", "Hey You");
// expecting 3 songs, total length of 16:17 and 0.01 Mb of free space left
ipod.deviceInfo();
ipod.removeSong("Pink Floyd", "Hey You");
// expecting 2 songs, total length of 11:36 and 10.83 Mb of free space left
ipod.deviceInfo();
Player sony("Sony Walkman", 64.0);
// expecting "Song was not found!"
sony.removeSong("The Doors", "Light My Fire");
sony.addSong("Nirvana", "Polly", "2:57", 6.76);
sony.addSong("Metallica", "Turn The Page", "6:06", 14.06);
sony.addSong("Cream", "White Room", "5:03", 11.64);
// expecting order: Nirvana, Cream, Metallica
sony.displaySongsByLength();
// expecting 3 songs, total length of 14:06 and 31.54 Mb of free space left
sony.deviceInfo();
}
/*
Song is already present.
Not enough memory.
Song info:
Band: Pink Floyd
Title: Hey You
Length: 4:41
Size: 10.82 Mb
Device info:
Name: iPod touch
Songs: 3/1000
Total length: 16:17
Free space left: 0.01 Mb
Device info:
Name: iPod touch
Songs: 2/1000
Total length: 11:36
Free space left: 10.83 Mb
Song was not found!
All songs sorted by length:
Band: Nirvana
Title: Polly
Length: 2:57
Size: 6.76 Mb
Band: Cream
Title: White Room
Length: 5:03
Size: 11.64 Mb
Band: Metallica
Title: Turn The Page
Length: 6:06
Size: 14.06 Mb
Device info:
Name: Sony Walkman
Songs: 3/1000
Total length: 14:06
Free space left: 31.54 Mb
*/
TimeStamp.cpp:
#include
using namespace std;
#include "TimeStamp.h"
TimeStamp::TimeStamp(string time) {
int index = time.find(':');
int minPart = stoi(time.substr(0, index));
int secPart = stoi(time.substr(index + 1));
sec = minPart * 60 + secPart;
}
TimeStamp::TimeStamp() {
sec = 0;
}
TimeStamp::TimeStamp(int _sec) {
sec = _sec;
}
int TimeStamp::toSeconds() {
return sec;
}
string TimeStamp::toString() {
string minPart = to_string(sec / 60);
string secPart = to_string(sec % 60);
return minPart + (sec % 60 < 10? ":0": ":") + secPart;
}
void TimeStamp::add(string other) {
TimeStamp temp(other);
sec += temp.sec;
return;
}
void TimeStamp::subtract(string other) {
TimeStamp temp(other);
sec -= temp.sec;
return;
}
bool TimeStamp::greaterThan ( string b) {
TimeStamp temp(b);
return sec > temp.sec;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
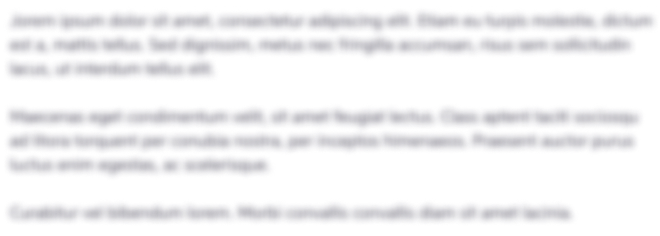
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started