Question
Design and implement a Point class which models points in the Cartesian coordinate system. Design... Design and implement a Point class which models points in
Design and implement a Point class which models points in the Cartesian coordinate system. Design...
Design and implement a Point class which models points in the Cartesian coordinate system.
Design and implement a Line class using the idea that 2 points determine a line.
Be sure to include the following methods: 1. A constructor with defaults. 2. Get and Set methods. 3. Appropriate useful methods.
Write a main driver program (no user input) that demonstrates the usage of your class designs.
//This is a start to it
#include
#include
#include
using namespace std;
class Point {//requires cmath
private:
double x, y;
public:
Point(double argx = 0, double argy = 0);
void setX(double argx);
void setY(double argy);
double getX(void);
double getY(void);
void displayPoint(void);
double distanceFromOrgin(void);
};
class Line {
private:
Point a, b;
public:
Line(Point argA = { 0,0 }, Point argB = { 0,0 });
void setA(Point arg);
void setB(Point arg);
Point getA(void);
Point getB(void);
void displayLine(void);
void displayEquation(void);
double calculateSlope(void);
double yInterceptVal(void);
double root(void);
double evaluate(double x);
};
int main(void) {
Point myPoint, yourPoint = (3, 4);
Line myLine;
return(0);
}
//Class Implementations
Point::Point(double argx, double argy) {
x = argx;
y = argy;
}
void Point::displayPoint(void) {
cout << "(" << getX << ")" << "(" << getY << ")" << endl;
}
Point::Point(double argx, double argy) {
setX(argx);
setY(argy);
}
void Point::setX(double argx) {
x = argx;
}
void Point::setY(double argy) {
y = argy;
}
double Point::getX(void) {
return (x);
}
double Point::getY(void) {
return (y);
}
double Point::distanceFromOrgin(void) {
return(sqrt(getX()*getX() + getY() + getY()));
}
//
Line::Line(Point argA, Point argB) {
setA(argA);
setB(argB);
}
void Line::displayLine(void) {
cout << "The points are";
getA().displayPoint();
getB().displayPoint();
}
void Line::setA(Point arg) {
a = arg;
}
void Line::setB(Point arg) {
b = arg;
}
Point Line::getA(void) {
return(a);
}
Point Line::getB(void) {
return(b);
}
we havent learn about files yet so if you could just continue or fix what i have i would apreciate it.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
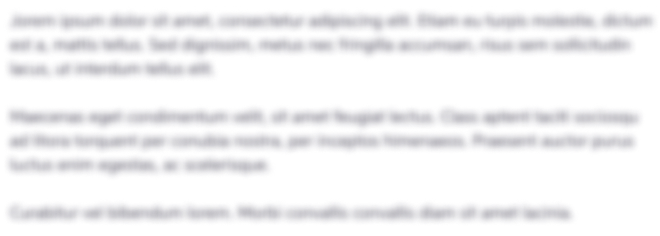
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started