Question
Design and implement a program to perform editing operations on a line of text. Your editor should be able to locate a specified target substring,
Design and implement a program to perform editing operations on a line of text. Your editor should be able to locate a specified target substring, delete a substring, and insert a substring at a specified location. The editor should expect source strings of less than 80 characters.
Here is what I have so far but I can't get it to run.
#include <stdio.h>
#include<string.h>
#include <ctype.h>
//Defines
#define MAX_LEN 80
#define NOT_FOUND -1
int index = 0;
int n = 0;
const char to_insert;
const char to_find;
char *delete(char *source, int index, int n);
char *do_edit(char *source, char command);
char get_command(void);
char *insert(char *source, const char *to_insert, int index);
int pos(const char *source, const char *to_find);
int main(void)
{
char source[MAX_LEN], command;
printf("Enter the source string: >");
gets(source);
for (command = get_command();
command != 'Q';
command = get_command())
{
do_edit(source, command);
printf("New Source %s", source);
}
printf("String after editing is: %s", source);
return(0);
{
char rest_str[MAX_LEN];
if (strlen(source) <= index + n)
{
source[index] = '0';
}
else
{
strcpy(rest_str, &source[index + n]);
strcpy(&source[index], rest_str);
}
return(source);
}
{
char str[MAX_LEN];
int index;
switch (command)
{
case 'D':
printf("String to delete");
gets(str);
index = pos(source, str);
if (index == NOT_FOUND)
printf("'%s' not found,str");
else
delete(source, index, strlen(str));
break;
case 'I':
printf("String to insert: ");
gets(str);
printf("Position of insertion is ");
scanf("%d", &index);
insert(source, str, index);
break;
case 'F':
printf("String to find: ");
gets(str);
index = pos(source, str);
if (index == NOT_FOUND)
printf("'%s' is not found at position %d", str, index);
break;
default:
printf("Invalid Edit Command '%c'", command);
}
return(source);
}
{
char command, ignore;
printf("Enter (D)elete,(I)nsert,(F)ind,or (Q)uit ");
scanf("'%c'", &command);
do
ignore = getchar();
while (ignore != '');
return (toupper(command));
}
{
char rest_str[MAX_LEN];
if (strlen(source) <= index)
{
strcat(source, to_insert);
}
else
{
strcpy(rest_str, &source[index]);
strcpy(&source[index], to_insert);
strcat(source, rest_str);
}
return(source);
}
{
int i = 0, find_len, found = 0, position;
char substring[MAX_LEN];
find_len = strlen(to_find);
while (NOT_FOUND && i <= strlen(source) - find_len)
{
strcpy(substring, &source[i], find_len);
substring[find_len] = '0';
if (strcmp(substring, to_find) == 0)
found = 1;
else
++i;
}
if (found)
position = i;
else
position = NOT_FOUND;
return(position);
}
}
this is in C.
Step by Step Solution
3.36 Rating (165 Votes )
There are 3 Steps involved in it
Step: 1
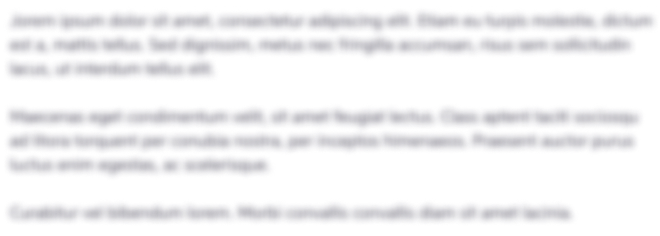
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started