Question
Design and implementation of a user interface. Master concepts on function: prototype, documentation, design, implementation, call. Practice on struct, string, pointers, pointer array, and dynamic
Design and implementation of a user interface.
Master concepts on function: prototype, documentation, design, implementation, call.
Practice on struct, string, pointers, pointer array, and dynamic memory allocation.
Learn separate compilation and linking for large project.
Get familiar with dynamic memory allocation.
Project Description
In this project, you are required to design a user interface that allows college applicants to access the basic admission information of the top 100 universities ranked by the U.S. news. Applicants can directly access school information, or make a data implementation, such as sorting and averaging on certain data. You will be given four files: school_database.txtand states.txt are the input data files, p2.c is a C code that has the main()function, andfunction.h is the header file that contains all the function prototypes. Your assignment is to implement all the functions defined in function.h in a file named function.c so themain() function in p2.c can run correctly. You can and are encouraged to define your own functions in function.c. However, you cannot make any changes in the four given files and you only need to submit function.c. Please read this description carefully.
General Requirements
For this project, your program should run with the following command:
a.out school_database.txt states.txt
wherea.outisthecompiledexecutablefile,school_database.txt isthenameofthefile that has all the university information, states.txt is the name of the file that has the full name and abbreviation of all the states including District of Columbia. You should do a safety check on this to ensure that you have the correct number of command line arguments and both input files are valid. The two input files from the command line arguments are elaborated below.
school_database.txt
The information of each school is listed with one school per row ended with a . Every row has exactly the same format as shown below (ignore the <>):
for private universities, and
for public universities.
where, Uname = nameoftheuniversity
Electrical & Computer Engineering Department, University of Maryland, College ParkFall 2018 ENEE150 Dr. Gang Qu
SAbb = abbreviationofthestatetheuniversityislocated GPA = averageGPAofthenewlyadmittedstudents SAT_M = averageSATmathscoreofthenewlyadmittedstudents SAT_R = averageSATreadingscoreofthenewlyadmittedstudents Pub_Pri = codeindicatingwhethertheschoolisaprivateinstitute(1=Yes,0=No)Tui_out = out-statetuition Tui_in = in-statetuition,onlyapplicableforpublicuniversities
For example,
states.txt
Yale University, CT, 4.35, 728, 712, 1, 53430
The file lists the 50 states in alphabetical order with DC as the 51st state. Each row shows one states full name and its abbreviation in the following format:
Description of the program
Maryland - MD
Once the program is running, you should first print out the following main menu (this portion is provided to you in a sample function.c):
Welcome to the admission checker! Here is a database of the nations top 100 universities.
1: Access Data 2: Data Implementation 0: EXIT Your choice:
0: terminate the program instantly. You must have this choice at any stages of the program. 1: Directly access the school information 2: Data implementation such as sorting and averaging
Then your program should keep on running based on the users choice until 0(EXIT) is chosen.First level choice 1: Access Data
On this option, your program should print out the following menu and wait for the user choice
1: ALL INFORMATION 2: GPA 3: SAT_MATH 4: SAT_READING
5: TUITION 6: LAST_STEP 0: EXIT
Electrical & Computer Engineering Department, University of Maryland, College ParkFall 2018 ENEE150 Dr. Gang Qu
where the LAST_STEP in choice 6 will return the program to the menu of the last level. In this case, the main menu that shows the first level choices: 1, 2, and 0. You must have this choice at any level except the first level.
Second level choices: 1-5
If the user chooses option between 1 and 5 in the last level, your program should print out the following menu and wait for the user choice
1: All Schools 2: Specific group 3: Specific school 4: LAST_STEP 0: EXIT
1: Print out the overall ranking, school name, state, and chosen information for all the schools.
2: Prompt the user to choose a specific group, then print the information for all the schools in
that specific group. Run the sample executable to get detailed information about this option.
3: Prompt the user to enter a valid school name, then print the information for that specific
school. Run the sample executable to get detailed information about this option.
Whenyouprintouttheinformation,usethefollowingformat(_ representsonespaceinUNIX, ignore the space in word. The number in parenthesis is the field width (that is, the number of UNIX spaces) for each piece of information):
_ _ _:_
Rank(3) name(60) state_abb(2) chosen information(depends on data)
If you are printing more than one piece of information, you must have 4 spaces between each two columns. For example, when you print out both GPA and SAT_Math score, use
_ _ _ _ 4.36 737
First level choice 2: Data Implementation
On this option, your program should print out the following menu and wait for the user choice
1: SORTING 2: AVERAGE 3: LAST_STEP 0: EXIT
1: Sort the schools based on the chosen criterion in the ascending order 2: Calculate the average of the chosen information for all the schools
Run the sample executable to get detailed information about each option. Pay attention to that the schools in the input file are sorted by the schools overall ranking. When you sort by another criterion, print out first the rank based on that criterion and then the overall school ranking.
Electrical & Computer Engineering Department, University of Maryland, College Park
Fall 2018 ENEE150 Dr. Gang Qu
NOTE:
You MUST use the data structure defined in p2.c and dont make any changes to the
given input files.
Make sure that you do the safety check on command line argument, input files, and users
choice at each level.
The number of characters in the university name and state name varies and may contain
space, you must use dynamic memory allocation to store them. Note that they are both
defined as pointer of char type in the structures for school and state (check p2.c).
For specific groups, the options are state, private, and public. If the user chooses state, you
should prompt the user to enter a valid case sensitive state name, either the full name or the
2-letter abbreviation.
If the user enters an invalid school or state name, keep asking the user to enter until a valid
input is provided or the user enters 0 to exit.
6.Readthesamplecodes(link.c, linkHead.c, linkHead.h)postedintheGLUE
public directory to see how to do the separate compilation and linking.
To pass the test cases. Please compile your code, run on GLUE and compare with the same
run with the same executable.
Passing a test case means that the output your code generates matches the output that the
sample executable generates. The match will be checked by the UNIX diff function.
We may change the school and state input files for test purpose. However, the number of
rows in these files will not change, that is, there will be 100 schools and 51 states.
Project Requirements:
The project must be implemented using C under the GLUE UNIX system in a file namedfunction.c whichshouldbesubmittedwiththefollowcommand
submit 2018 fall enee 150 010x 3 function.c
Your submission must conform to the expectations outlined above for program execution and input files.
Your submission must complete and implement all the given function prototypes
Code must be reasonably commented to allow to a user to follow the follow of their
program as well as neat as orderly.
Grading Criteria:
Correct compilation 5% Correct implementation of given functions 15% Use a pointer array to store all the school information 5% Pass all public test cases 30% Pass all secret test cases 40% Coding style and documentation 5%
Files that cannot be compiled will be given a 0. Late penalty is 20% per day. Submissions more than two days late will not be accepted.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
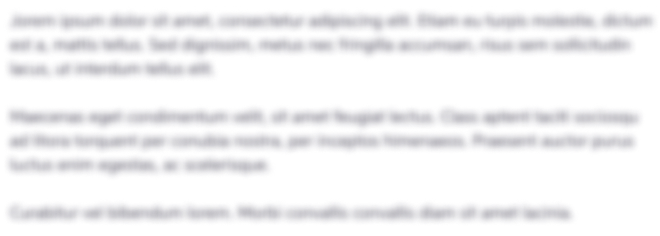
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started