Question
Design, by creating a UML diagram, a class named MyInteger. The class contains: An int data field named value that stores the int value represented
Design, by creating a UML diagram, a class named MyInteger. The class contains:
An int data field named value that stores the int value represented by this object.
A constructor that creates a MyInteger object with the passed int value.
A get method that returns the int value.
The instance methods isEven(), isOdd(), and isPrime() that return true if the value in this object is even, odd, or prime, respectively.
The static methods isEven(int), isOdd(int), and isPrime(int) that return true if the specified value is even, odd, or prime, respectively.
The static methods isEven(MyInteger), isOdd(MyInteger), and isPrime(MyInteger) that return true if the specified value is even, odd, or prime, respectively.
The instance methods equals(int) and equals(MyInteger) that return true if the value in this object is equal to the specified value.
A static method parseInt(char[]) that converts an array of numeric characters to an int value.
A static method parseInt(String) that converts a string into an int value.
Here is MyInteger Class:
class MyInteger { private int mValue;
public MyInteger(int value) { mValue = value; }
public int getValue() { return mValue; }
public boolean isEven() { return (mValue % 2) == 0; }
public boolean isOdd() { return (mValue % 2) == 1; }
public boolean isPrime() { if (mValue == 1 || mValue == 2) { return true; } else { for (int i = 2; i < mValue; i++) { if (i % mValue == 0) return false; } } return true; }
public static boolean isEven(int myInt) { return (myInt % 2) == 0; }
public static boolean isOdd(int myInt) { return (myInt % 2) == 1; }
public static boolean isPrime(int myInt) { if (myInt == 1 || myInt == 2) { return true; } else { for (int i = 2; i < myInt; i++) { if (i % myInt == 0) return false; } } return true; }
public static boolean isEven(MyInteger myInt) { return myInt.isEven(); }
public static boolean isOdd(MyInteger myInt) { return myInt.isOdd(); }
public static boolean isPrime(MyInteger myInt) { return myInt.isPrime(); }
public boolean equals(int testInt) { if (testInt == mValue) return true; return false; }
public boolean equals(MyInteger myInt) { if (myInt.mValue == this.mValue) return true; return false; }
public static int parseInt(char[] values) { int sum = 0; for (char i : values) { sum += Character.getNumericValue(i); } return sum; }
public static int parseInt(String value) { return Integer.parseInt(value); } }
If there is a better code based on what I just posted and the question, then i'd appreciate it.
At this point, I have trouble to create following intructions in the TestMyInteger.java
The instructions are as follows:
Once your UML diagram is complete implement the class naming the Java file MyInteger.java. Create another Java file, a program named MyIntegerTester.java that tests all methods in the MyInteger class by creating a MyInteger object with a value, then calling all the methods and checking that they do what they are supposed to do. You can "wire in" the test data, no need to prompt the user for anything. One technique is to write both classes at the same time. As much as possible you implement and test one method at a time. This minimized the amount of new code you have to look at when debugging a method. Only the tester file will have main() method in it.
In the end, I would likke a code that prints out like this based on the assignment.
Tests use MyInteger n1 object constructed with MyIneger(5)
Testing equals(int), n1 and 6: false
Testing equals(MyInteger), n1 and n2 = 7: false
Testing isEven(), n1 which is 5, is even? false
Testing isOdd(), n1 which is 5, is odd? true
Testing static isPrime(), n1 which is 5, is prime? true
Testing instance isPrime(), 5 is prime? true
Next two tests use char[] and Sring data of 3539
Testing parseInt(char[]): 3539
Testing parseInt(String)Passing: 3539
Overall the output of whenn the MyIntegerTester.java is completed should like something like that above. I would also like SCREENSHOTS if possible of the current programs being run
Step by Step Solution
There are 3 Steps involved in it
Step: 1
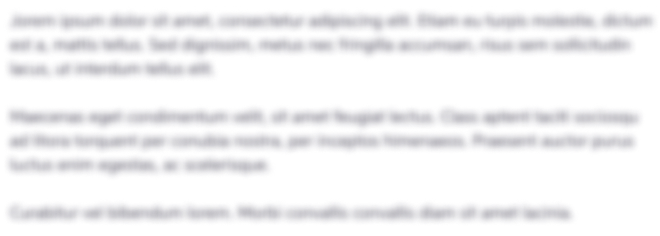
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started