Question
Design MoneyMarketAccount class that inherits the BankAccount classs, set the interest rate to 4% -> 0.04. Create two MoneyMarket Accounts in TestAccounts class and state
Design MoneyMarketAccount class that inherits the BankAccount classs, set the interest rate to 4% -> 0.04.
Create two MoneyMarket Accounts in TestAccounts class and state them to Bank Account array and display their info. Java
Savingsaccount code
public class SavingsAccount extends BankAccount {
public SavingsAccount()
{
super();
}
public SavingsAccount(int acctNumber, String name, double balance, double interestRate){
super(acctNumber, name, balance, interestRate);
}
public double calcInterest(){
return getBalance() * getInterestRate();
}
}
Bankaccount code
public abstract class BankAccount {
private int acctNumber;
private String name;
private double balance;
private double interestRate;
public BankAccount(){
acctNumber = 0;
name ="";
balance=0.0;
interestRate = 0.0;
}
public BankAccount(int acctNumber, String name, double balance, double interestRate){
this.acctNumber = acctNumber;
this.name =name;
this.balance=balance;
this.interestRate = interestRate;
}
public void setAcctNumber(int acctNumber)
{
this.acctNumber = acctNumber;
}
public int getAcctNumber()
{
return acctNumber;
}
public void setName(String name)
{
this.name = name;
}
public String getName()
{
return name;
}
public void setBalance(double balance)
{
this.balance = balance;
}
public double getBalance()
{
return balance;
}
public void setInterestRate(double interestRate)
{
this.interestRate = interestRate;
}
public double getInterestRate()
{
return interestRate;
}
public abstract double calcInterest();
@Override
public String toString() {
return "Account number : "+ acctNumber + " with account owner " + name + " has a balance of "+ balance + " has interestRate " + interestRate;
}
}
Test Account
import java.util.*;
public class TestAccounts {
public static void main(String[] args){
BankAccount C1 = new CheckingAccount();
C1.setAcctNumber(1002);
C1.setName("Bob Lee");
C1.setBalance(1500.00);
C1.setInterestRate(0);
BankAccount S1 = new SavingsAccount();
S1.setAcctNumber(2005);
S1.setName("Victor Taylor");
S1.setBalance(8000.00);
S1.setInterestRate(0.02);
BankAccount C2 = new CheckingAccount(1001, "John smith", 5000.00, 0.0);
BankAccount S2 = new SavingsAccount(2001, "Mary Smith", 3000.00, 0.01);
BankAccount [] Accounts = {C1, C2, S1, S2};
System.out.println("Checking Account interest :" + C2.calcInterest());
System.out.println("Savings Account interest :" + S2.calcInterest());
for(BankAccount ba: Accounts)
System.out.println(ba.toString());
}
}
Checking account code
public class CheckingAccount extends BankAccount {
public CheckingAccount()
{
super();
}
public CheckingAccount(int acctNumber, String name, double balance, double interestRate){
super(acctNumber, name, balance, interestRate);
}
public double calcInterest(){
return 0;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
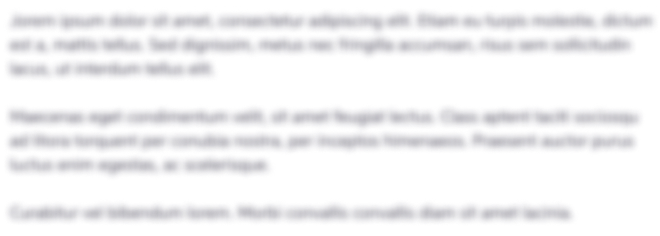
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started