Question
Develop a complete Java program for each of the following problems. Please name the programs as indicated and add proper program headers and output labels
Develop a complete Java program for each of the following problems. Please name the programs as indicated and add proper program headers and output labels as specified. Do not use loops, arrays, methods, or other concepts we did not discuss to date, please build on what we have covered to date.
Program #1 (10 points): Write a Java program (name it RandomNumbersYourname) that generates random numbers as follows.
a) A random integer number between 25 and 45 (inclusive).
b) A random integer number between -20 and 20 (inclusive).
c) A random integer number between -50 and -20 (inclusive).
d) A random floating-point number between 0.0 and 21.9999 (inclusive).
Properly label output for each part above, print the outputs on separate lines, and use the tab escape character (\t) to line-up the outputs after the labels (Formatting is also one of the grading item) as shown in this sample run:
a) A random integer between 20 and 80 (inclusive): 51 b) A random integer between -20 and 20 (inclusive): -9 c) A random integer between -50 and -20 (inclusive): -31 d) A random float between 0.0 and 21.9999 (inclusive): 19.9048
Separate your code into sections with proper in-line comments such as
// Part a): Generate integer number between 20 and 80 (inclusive)
Program #2 (10 points): Write a Java program (name it StringMethodsYourname) that reads from the user two strings (say string_1, and string_2). The program uses String class methods to manipulate these input strings as follows and prints out the outcome as shown below.
a) Determine the length of string_1.
b) Determine the length of string_2.
c) Concatenate both strings, separated by space.
d) Check if the two strings have same set of characters with regard to case (i.e., equal).
e) Convert string_1 to upper case.
f) Convert string_2 to lower case.
g) Extract one valid sub-string of multiple characters from string_1.
Make sure to properly label your output prompts for each manipulation above and print the outputs on separate lines. Use the escape character (\t) to line-up the outputs after the labels as follows (for inputs: John and Amy):
a) Length of String 1: 4 characters
b) Length of String 2: 3 characters
c) Concatenation: John Amy
d) Equal Strings? Not equal
e) Uppercase String 1: JOHN
f) Lowercase Sting 2: amy
g) Valid substring: oh
Separate your code into sections with proper in-line comment before each section, such as
// Part A: Determine the length of string_1
Program #3 (10 points): Write a Java program (name it MathMethodsYourname) that uses the MATH class methods to perform the following tasks.
a) Prompt the user to enter a negative integer number and print out its absolute value.
b) Prompt the user to enter a floating-point number representing an angle and print out the angles cosine, sine, and tangent values.
c) Prompt the user to enter a floating point number and print out both of its floor and ceiling values.
d) Prompt the user to enter two floating-point numbers (say X and Y) and print out the value of XY (X is raised to the power of Y).
e) Prompt the user to enter an integer number and print out its square root. Try that with both negative and positive inputs.
Make sure to properly label your output for each task and print the outputs on separate lines. Use the tab escape character to line-up the outputs after the labels. See sample run below.
Enter a negative integer number: -5 The absolute value of -5 is: 5 Enter a floating-point number representing an angle: 0.45 The cosine of this angle is: 0.90044710753787 The sine of this angle is: 0.43496552337706423 The tangent of this angle is: 0.48305505091399376 Enter a floating point number: 5.39 The floor value of 5.39 is: 5.0 The ceiling value of 5.39 is: 6.0 Enter a floating-point number as X: 2.0 Enter a floating-point number as Y: 3.0 The value of X^Y is: 8.0 Enter an integer number: 16 The square root of 16 is: 4.0
The number in the label is an users input. Not a static value.
Enter a floating point number: 5.39 The floor value of 5.39 is: 5.0
Separate your code into sections with proper in-line comments such as
// Part A: Enter a negative number and print its absolute value
Step by Step Solution
There are 3 Steps involved in it
Step: 1
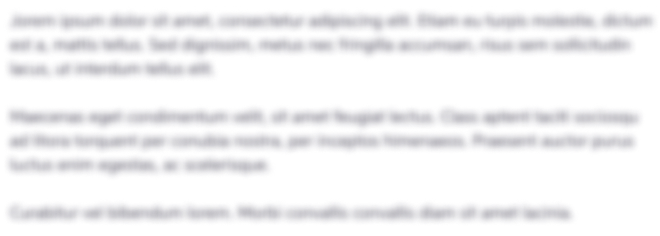
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started