Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Develop a console - based inventory management system using C + + that utilizes both arrays and vectors to store and manipulate inventory data. This
Develop a consolebased inventory management system using C that utilizes both arrays and vectors to store and manipulate inventory data. This assignment will help you understand the basics of arrays and vectors in C including their manipulation, dynamic resizing in the case of vectors and practical application in a simple inventory system.
Skills Tested:
Array and Vector manipulation
Basic InputOutput in C
Conditional statements and loops
Basic understanding of classes
Instructions:
Setup Your Development Environment:
Ensure you have a C compiler installed like GCC or Clang
Use any IDE or text editor of your choice eg Visual Studio Code, Code::Blocks
Define the InventoryItem Structure:
Create a structure named InventoryItem with the following fields:
string name for the item's name.
int quantity for the quantity in stock.
float price for the price per item.
Implement ArrayBased Functions:
Initialize a fixedsize array of InventoryItem with a predefined size.
Develop functions to add, display, and search items in the array.
Handle scenarios where the array is full.
Implement VectorBased Functions:
Create a vector of InventoryItem.
Develop similar functions as for the array, demonstrating vectors' dynamic nature.
Develop the Main Program:
Write a main function to implement a menudriven approach.
Allow the user to choose between the array or vectorbased system.
Enable operations like adding an item, viewing all items, and searching for an item.
Incorporate Error Handling:
Ensure the program handles errors, such as searching for a nonexistent item.
Document the Code:
Comment the code to explain the logic, particularly at crucial junctures.
Implement Using a Class:
Use a class for the inventory instead of structures and functions.
Add Item Deletion Feature:
Include functionality to delete an item from the inventory.
Implement Sorting Feature:
Add a feature to sort items by name or quantity.
Add Item Update Feature:
Implement functionality to update the quantity or price of an existing item.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
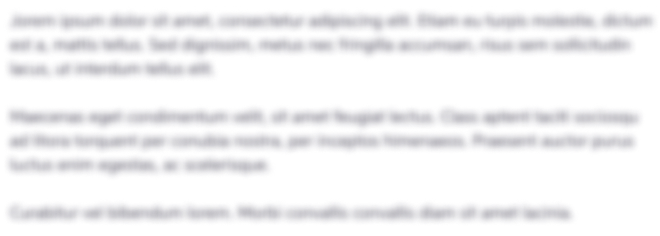
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started