Question
Develop a JUnit like test tool MyJUnit that extends class TestHarness. In TestHarness, there are two overloading methods boolean checkEqual(int actual, int expected) and boolean
Develop a JUnit like test tool MyJUnit that extends class TestHarness. In TestHarness, there are two overloading methods boolean checkEqual(int actual, int expected) and boolean checkEqual(double actual, double expected) for comparing primitive data type values.
The MyJUnit can accept multiple testlet class names, such as java MyJUnit RectangleTestlet BoxTestlet, to test testlet classes in order. Most importantly, the MyJUnit tool must be able to handle new testlet classes in the future without a need of code change. All the testlet classes implement an interface called TestletIF which defines a runTest method. Feel free to specify the argument for the method.
Please prepare two testlet classes to test the two classes Rectangle and Box, respectively. The Rectangle class has a method int getArea(int w, int h), while the Box class has a method double getVolume(double l, double w, double h). Assume all input variables should range between 1 and 10. Perform boundary value analysis for the two classes through the two testlets.
Hint for Part 2 Here shows a sample snippet for RectangleTestlet. The ??? is an object used to run checkEqual. This object should be passed in not created by using the keyword new, such as new TestHarness(). In other words, the TestHarness object should be the same one that creates the Testlets instead of being a new object.
package pkg;
public class RectangleTestlet implements Testlet {
Rectangle r = new Rectangle();
public void runTest() {
???.checkEqual(r.getArea(5,5), 25); // need object ??? to run checkEqual
}
}
To create an object of RectangleTestlet, you will need to take advantage of Reflection. Simple snippet code is shown below. This way of coding allows the tool to take in whatever Testlet classes that are developed in the future.
package pkg;
public class MyProg {
public static void main(String[] args){
Class c = Class.forName(args[0]); // The args[0] would be pkg.RectangleTestlet
Object o = c.newInstance();
// Now cast the object to a Testlet class.
// With the Testlet object, you can run its method.
}
}
The problem is how to access the TestHarness object from your Testlet object. A good solution is to pass the TestHarness object to the Testlet's method. In that case, you can utilize the TestHarness object to do all sorts of comparison that are available in the TestHarness object.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
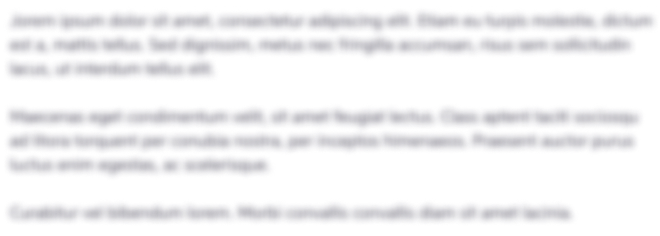
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started