Question
Develop the following Python program: a tool to convert binary numbers to decimal, and decimal numbers to binary, and a simple text-only menu to add
Develop the following Python program: a tool to convert binary numbers to decimal, and decimal
numbers to binary, and a simple text-only menu to add or subtract numbers. In this program,
binary numbers will be respresented as text strings of only 1s or 0s, and should always appear in
strings that are multiples of eight characters long (length of 8, or 16, or 24, ....) In case the number
to be represented does not require the full amount of the length, the leading positions are lled
with 0s. Decimal and binary numbers will all be integers.
The algorithm to convert binary numbers to decimal is to start at the high end, scanning through
the string from left to right. Each time a 1 character is encountered, the value of 2 raised to the
appropriate power is added in. Recall that the last position is 20, or the value of 1. (In an eight-bit
binary string, the rst position is 27, or 128.)
The algorithm to convert decimal numbers into binary is as follows.
1. First, divide the number by 2 using integer divison, retaining both the quotient and the
remainder.
2. The remainder becomes the last character of the binary string, whether 1 or 0.
3. The quotient is then divided by 2 again, using integer division and retaining both the quotient
and the remainder.
4. This new remainder is pre-xed to the front end of the binary string.
5. Steps 3 and 4 are repeated as often as necessary, until both the quotient and remainder come
out as zero.
6. Once the binary number string is completed, sucient zeros are pre-xed to the font end to
round out a multiple of eight bits in length.
Each of the conversion routines should be placed inside of functions to be easily called from the
main program. (As desired, they could be spread over several functions, if needed, but each of
them ought to have one main function that is to be called to start the conversion.)
Once both conversion routines are ready, a simple menu program should be written. It prints out
to the screen a menu that can be similar to the following:
What do you want to do?
1. Enter the rst number
2. Enter the second number
3. Add the two numbers together
4. Subtract the second number from the rst
5. Exit the program
The user makes a choice by entering 1 through 4 as desired. If the rst number is entered, it is
shown back to the user with appropriate text to explain it. If the second number is entered, both
numbers are shown back to the user, with text explanations. In either case, the menu is displayed
again afterwards. When option 3 is selected, the two numbers are added together and the sum
displayed. All of these numbers are to be entered by the user as binary text strings, converted to
decimal, and added, and converted back for display on the screen. (You may choose to display
both decimal and binary on the screen, or only the binary.)
When option 4 is selected, subtraction is performed rather than addion. In this situation, care
should be taken to avoid negative numbers. Your program will have to include some mechanism to
detect the possibility of a negative number and responding gracefully should this happen.1
Step by Step Solution
There are 3 Steps involved in it
Step: 1
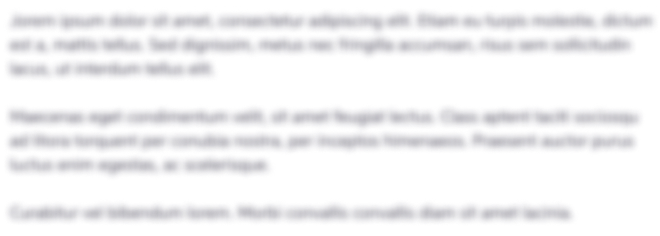
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started