Question
dice.css body { font-family: Arial, Helvetica, sans-serif; background-color: white; margin: 0 auto; width: 450px; border: 3px solid blue; padding: 0 2em 1em; } h1 {
dice.css
body { font-family: Arial, Helvetica, sans-serif; background-color: white; margin: 0 auto; width: 450px; border: 3px solid blue; padding: 0 2em 1em; } h1 { color: blue; margin-bottom: .25em; } label { width: 4em; text-align: right; padding-bottom: .5em; } input { width: 4em; margin-left: .5em; margin-bottom: .5em; } fieldset { margin-bottom: 1em; } #turn { display: none; } #turn.open { display: block; } #turn p { font-weight: bold; color: red; } #player1, #player2 { width: 10em; } #new_game { width: 6em; margin-left: 1em; } #hold { margin-right: 2em; }
dice.js
"use strict"; var $ = function(id) { return document.getElementById(id); };
var newGame = function() { }; var takeTurn = function() { }; var holdTurn = function() { }; window.onload = function() { $("new_game").onclick = newGame; $("roll").onclick = takeTurn; $("hold").onclick = holdTurn; $("player1").focus(); // call the load method and pass it the page elements the game object needs game.load($("player1"), $("player2"), $("score1"), $("score2"), $("current"), $("die"), $("total")); };
library_die.js
var Die = function() {}; Die.prototype.rollDie = function() { var random = Math.random(); random = Math.floor(random * 6); return random + 1; };
library_game.js
var game = { player1: null, // these properties will be set by the load method player2: null, currentPlayer: null, load: function(name1Node, name2Node, score1Node, score2Node, currentNode, dieNode, totalNode) { this.player1 = { name: name1Node, score: score1Node, pig: new Pig() }; this.player2 = { name: name2Node, score: score2Node, pig: new Pig() }; this.currentPlayer = { name: currentNode, roll: dieNode, total: totalNode, pig: this.player1.pig // initial value - will be changed by changePlayer method }; return this; }, isValid: function() { if (this.player1.name.value === "" || this.player2.name.value === "") { return false; } else { return true; } }, clearScores: function() { // reset player1 score value and reset its Pig object // reset player2 score value and reset its Pig object }, setInitialPlayer: function() { // if game is valid set initial player by calling the changePlayer method }, takeTurn: function() { // use the Pig object of the currentPlayer property to take a turn }, changePlayer: function() { // display result of last roll in the currentPlayer display properties // if current player's turn is zero, need to switch players // and start new turn }, hold: function() { // use the currentPlayer Pig object to hold // determine whether player1 or player1 are the current player, then // update that player's score with the current total }, checkWinner: function() { // check the players' Pig objects to see if either is at or above 100 // total points. If so, return that player's name. Otherwise, return // the string "none". } };
library_pig.js
var Pig = function() { // properties to keep track of the current roll, the current // turn, and the game total this.total = 0; this.turn = 0; this.roll = 0; }; // inherit from Die object Pig.prototype = new Die();
Pig.prototype.takeTurn = function() { // use inherited method to roll the die this.roll = this.rollDie(); // use inherited method to roll the die // update or reset the turn property based on result of die roll this.turn = (this.roll === 1) ? 0 : this.turn + this.roll; }; Pig.prototype.hold = function() { // update the game total this.total = this.total + this.turn; // reset other properties for next turn this.roll = 0; this.turn = 0; }; Pig.prototype.reset = function() { // reset all properties this.total = 0; this.roll = 0; this.turn = 0; };
index.html
Let's Play PIG!
's turn
Step by Step Solution
There are 3 Steps involved in it
Step: 1
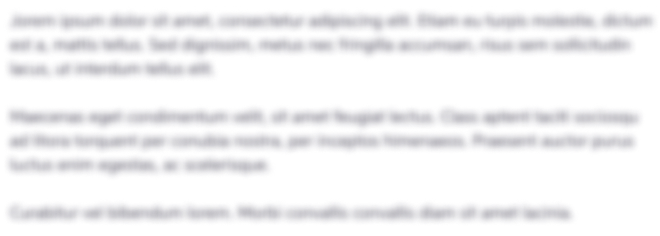
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started