DIRECTIONS: First, create a new file inside the tracker folder named Exercise.js We are going to make a module that contains two files: trackerService.js and
DIRECTIONS:
-
First, create a new file inside the tracker folder named Exercise.js We are going to make a module that contains two files: trackerService.js and Exercise.js. This will help you understand how npm works
-
Open the new file in your VS Code Editor and then: a) Copy the definitions for the exercise objects from trackerService.js (lines 60-70, inclusive) into Exercise.js
b) create an Exercise class definition with a constructor that accepts a single parameter (see simpleObjects.js for an example). This parameter will be a String. Note this is separate from the exercise objects placed in this file
c) The Exercise class will have 2 instance properties. One will be named activity and will reference one of the exercise function objects. For Example:
this.activity = new walking();
The other will be named type and will hold the name of the activity. Inside the constructor, check the string parameter's value. If it is "walking" create an instance of walking exercise object and assign it to the activity instance property, if it is "running" create and assign an instance of the running exercise object, else throw an error. Finally, create an instance property named type (if you haven't already done so ) and set its value to be the value of the parameter.
Up to this point, you are basically just moving the logic from tracker constructor to the Exercise constructor
d) Add a function named calculate to the Exercise class. The function accepts "weight" and "distance" parameters. It will pass these values to the object's activity's calculate function and returns the value.
This would be called by writing something like
this.activity.calculate(weight, distance);
e) export the Exercise class (VERY IMPORTANT)
module.exports = Exercise;
f) save the file
3) Inside the trackerService file:
a) At the top, import the Exercise definition:
var Exercise = require("./Exercise.js");
b) In the tracker constructor, get rid of the redundant if/else branches (We just moved these into Exercise). Replace them with a call to create a new instance of Exercise(type).
this.exercise = new Exercise(exercise);
This should automatically assign the exercise and calculate method.
You should leave the try catch statement alone.
c) you can remove the local definitions for walking and running exercise objects if you haven't already
d) note that we are exporting the tracker from here. if you read the package.json file you'll notice that trackerService is the main file for this module. Therefore whatever is exported from trackerService will be made available whereever this is imported (not the Exercise).
e) save the file!
- Look at the package.json file. this is the module metadata from its initial state. Currently it should some version 0.1.2 Go ahead and change the version number to 0.1.3.
Then, run
npm pack
This should create a new .tgz file (tracker-0.1.3.tgz).
-
Go into the ActivityTracker folder and run:
npm install ../tracker/tracker-0.1.3.tgz
check to make sure it all works by running (from within the ActivityTracker folder):
node activityTracker.js
As long as it works then you're ready for the final step. If it doesn't work, you may have to review these steps to make sure you've done everything here. Fix whatever is wrong, then re-pack the tracker module, and then re-install it into the ActivityTracker folder.
Notice that the activityTracker.js file has more object literals to process AND the last object is a swimming object. This version of the tracker cannot recognize swimming activities. We'll fix this in the last step of the lab.
By modularizing the Exercises, we've made it much easier to reuse them (DRY), made it easy to add more (extension) of them, separated the functionality of the tracker from the functionality of the calculation (SRP), and we abstracted/encapsulated the things that can change. We can add as many "Exercises" to the program as we'd like, without ever having to change the trackerService file. And Exercises can be "plugged in" elsewhere, if we need them.
- Now adding more types of activities is relatively easy. Currently the driver does not recognize "swimming". All we need is an else if branch in the Exercise class constructor, and a new exercise function object for the appropriate calculation to fix this.
Add a function Object named "swimming" to the Exercise.js file. Use the following formula:
calories burned = (6 * Weight in KG * 3.5) / 200;
However, there is a slight problem. This formula only provides CALORIES BURNED PER MINUTE not total calories, unlike the other two formulae. To get the data we want, we need the time.
Since we also need all exercise function signatures to match, that means you need all of the function objects to accept three parameters (weight, distance, time). In scenarios like this, it's ok to accept parameters that you will ignore.
So walking and running now need a time parameter added to the end of their parameter lists, but thats it. There is no need to change their calculations. And swimming should have matching signature. In the swimming calculation, you can ignore distance parameter and return total calories using time and the given formula.
We can add other types of exercise similarly, though we won't always need to add a new parameter; the formula for swimming just happened to need something more.
NOTE: the formula requires weight in kg but the array of Object literals in activityTracker.js provides weight in pounds. 1 kg = 2.2 pounds, for the purposes of this exercise.
Don't forget to update your package.json files
Today We Looked At How To Define And Export Object... | Chegg.com
can I get correct code with output for this exercise please?
Here is the code:
Trackersrive.js file:
/*trackerService.js
Provides Activity Tracker Functionality utilizing Strategy Design Pattern.
Note that this file uses ES6 syntax for defining a class.
Activities: Walk/Run
Input: Exercise type
Distance (miles)
Weight (lbs)
Time (minutes)
Output: Calories Burned
Formula Sources:
https://lovandy.com/wellness/physical/calories-burned-by-walking.html AND
https://lovandy.com/wellness/physical/calories-burned-running.html
*/
class tracker{
constructor(exercise, weight, distance, time) {
try{
if (exercise.toString().toLowerCase() == "walking"){
this.exercise = new walking(); //exercise is a new instance of
//of the function objects below
} else if (exercise.toString().toLowerCase() == "running") {
this.exercise = new running();
} else {
//throwing errors is as simply as throwing an object literal
//with a message property.
throw { message: "Unknown exercise!"}; //if the exercise is unknown
}
//Note that, like constructors in Java/C++, nothing is being returned
//from the constructor. We have to use keyword this to differentiate
//between a property of the object that is being created and a value passed in
this.weight = Number(weight);
this.distance = Number(distance);
this.time = Number(time);
//catch any error thrown in object creation and re-throw it to
//calling module.
} catch (err){
console.log("Error recieved during object creation");
throw err;
}
}
calculate(){
return this.exercise.calculate(this.weight, this.distance);
}
calcSpeed(){
return this.distance/(this.time/60); //returns in miles per hour
}
}
//This calorie calculations for walking and running are
//implemented as Function Objects. These can be assigned
//to this.exercise at runtime.
var walking = function(){
this.calculate = function (weight, distance){
return 0.3 * weight * distance;
}
};
//requires weight in lbs, and distance in miles
var running = function(){
this.calculate = function (weight, distance){
return 0.63 * weight * distance;
}
};
//Export statements expose data we want to be "importable". In this case
//I want to export the tracker class (including its prototypes). I don't
//want to export the function objects (walking and running) from here because
//they are for internal use only.
module.exports = tracker;
//Your Lab assignment will be to further modularize this code and move most
//of the exercise specific stuff into an Exercise module.
this is the first homework file of Tracker service :Index.js
var tracker = require("./tracker/trackerservice.js");
var activities = [{ activity: "walking", weight: 150, distance: 3, time: 45 }, { activity: "running", weight: 200, distance: 4, time: 40 },
{ activity: "running", weight: 175, distance: 5, time: 45 }, { activity: "running", weight: 140, distance: 10, time: 240 }]
var index = 0;
for (let activity of activities) {
console.log("Activity: " + (index + 1));
if (activity.activity == "walking") {
console.log(activity.activity);
console.log("Weight: " + activity.weight + " lbs");
console.log("Speed: " + tracker.calcSpeed(activity.distance, activity.time) * 60 + "miles per hour");
console.log("Calories Burned: " + tracker.calcWalkCal(activity.weight, activity.distance));
}
else if (activity.activity == "running") {
console.log(activity.activity);
console.log("Weight: " + activity.weight + " lbs");
console.log("Speed: " + tracker.calcSpeed(activity.distance, activity.time) * 60 + "miles per hour");
console.log("Calories Burned: " + tracker.calcRunCal(activity.weight, activity.distance));
}
console.log("");
index = index + 1;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
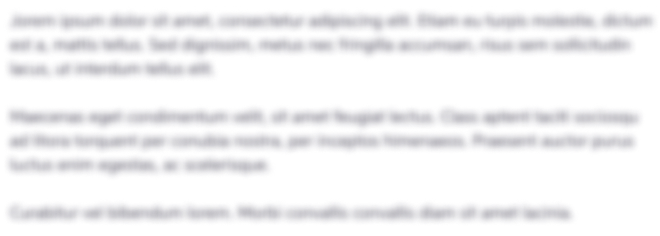
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started