Question
DIY (50%) Your task for part two of this workshop is to create a class module to represent a Bus. Develop your module int files
DIY (50%) Your task for part two of this workshop is to create a class module to represent a Bus. Develop your module int files Bus.h and Bus.cpp The Bus class encapsulates the number of seats and the number of passengers in a bus. global constant value The price of a bus ticket is $125.34 and will not change throughout the program. the constructor A Bus can be instantiated using the number of seats and the number of passengers on board. By default, a bus has 20 seats and no passengers. When created the following validation is done on the arguments of the constructor: number of seats must be a coefficient of 2 number of seats must be between 10 and 40 (inclusively) the number of passengers should be between 0 and the number of seats. If the values do not match the above criteria, the bus is considered out of service. Displaying a bus on the screen. A bus can be drawn on the screen by getting inserted into the cout object and under the drawing, on a new line the total fare for the bus, based on the number of passengers and price of one ticket must be displayed. For example, a Bus with 12 seats and 7 passengers should be displayed as follows: ______________________ | [2][2][2][1][ ][ ]|_\_ | Seneca College ) `---OO-------------O---' Total Fare Price: 877.38 If the Bus is not in service the following message should be displayed instead: Out of service! Use the following function in your display routine as a private member of Bus class to draw the bus on the screen. // draws a bus with // seat = number of seats // psng = number of passengers // on ostream void drawBus(ostream& ostr, int seats, int psng) { int i, p; ostr.fill('_'); ostr.width((seats / 2) * 3 + 4); ostr << "_"; ostr << endl << "| "; for (i = 0, p = -1; i < (seats / 2); i++, ostr << "[" << ((p += 2) < psng ? '2' : ((p == psng) ? '1' : ' ')) << "]"); ostr << "|_\\_" << endl; ostr << "| "; ostr.fill(' '); ostr.width(((seats / 2) * 3 - 14) / 2); ostr << " " << "Seneca College"; ostr.width(((seats / 2) * 3 - 14) / 2 + (seats % 4 != 0)); ostr << " " << " )" << endl; ostr << "`---OO"; ostr.fill('-'); ostr.width((seats / 2) * 3 - 5); ostr << "-" << "O---'" << endl; } you may modify this code as long as the output is not changed. Reading the specs. of a bus form console: The specifications of a bus can be read from the console by extracting a Bus object from istream (cin). Extraction should be done as follows read an integer into the number of seats ignore the next character. read an integer into the number of passengers. perform the same validation on the attributes as the constructor and if it fails, set the bus to out of service. Type Conversion Operator overloads Boolean conversion. This read only conversion returns true if the number of seats is greater than zero. (i.e. Returns true if the bus is in service) Integer conversion This read only conversion returns the number of passengers if the Bus is in service otherwise, it will return -1 Double Conversion This read only conversion returns the total fare of the bus for the trip using the number of passengers on the bus and the price of a ticket. If the Bus is out of service this conversion returns -1.0. Unary Operator overloads: prefix operator-- If the bus is out of service or empty, this operator returns false. Otherwise, it will reduce the number of passengers by one and returns true; prefix operator++ If the bus is out of service or full, this operator returns false. Otherwise, it will increase the number of passengers by one and returns true; postfix operator-- Works exactly like the prefix operator-- postfix operator++ Works exactly like the prefix opeator++ Binary Member Operators Assigning A bus object to an integer value. Sets the number of passengers of a bus by assigning it to the integer value and If the number of passengers exceeds the number of seats, the bus will be out of service. Returns a reference to the current object at the end. Bus B(12,0); cout << (B = 7); will print the following: ______________________ | [2][2][2][1][ ][ ]|_\_ | Seneca College ) `---OO-------------O---' Total Fare Price: 877.38 Adding an integer to a Bus object using += operator Adds the integer value to the number of passengers if the bus is in service. If the number of passengers exceeds the number of seats, the bus will go out of service. Returns a reference to the current object at the end. Bus B(10,2); cout << (B += 5); Will print the following: ______________________ | [2][2][2][1][ ][ ]|_\_ | Seneca College ) `---OO-------------O---' Total Fare Price: 877.38 Adding a bus object to another bus object using += operator. If both busses are in service it will move the passengers from one bus (right) to another (left). If there is not enough seat in the left bus, the rest of the passengers remain in the right bus. In the end, a reference to the left bus is returned. Bus A(10, 2); Bus B(20, 12); cout << A << B; cout << (A += B) << B; Will print the following: ___________________ | [2][ ][ ][ ][ ]|_\_ | Seneca College ) `---OO----------O---' Total Fare Price: 250.68 __________________________________ | [2][2][2][2][2][2][ ][ ][ ][ ]|_\_ | Seneca College ) `---OO-------------------------O---' Total Fare Price: 1504.08 ___________________ | [2][2][2][2][2]|_\_ | Seneca College ) `---OO----------O---' Total Fare Price: 1253.40 __________________________________ | [2][2][ ][ ][ ][ ][ ][ ][ ][ ]|_\_ | Seneca College ) `---OO-------------------------O---' Total Fare Price: 501.36 Comparing two buses using the == operator. If both buses are in service and the numbers of passengers are the same, this operator returns true, otherwise, it will return false. Bus A(10,5); Bus B(20,5); if (A == B) cout << "Same number of passnegers" << endl; Helper Binary Operator Overload sum of an integer number (at left) and a bus (at right) If the bus is in service, this operation should return the sum of the number and the number of passengers on the bus. Otherwise, the integer number is returned as if the number of passengers on the bus was 0. Bus A(10,5),B(5,50); int I = 3 + A; // I will be 8 I = 10 + B; // I will be 10 Tester Program // Workshop #5: // Version: 1.0 (corrected typeconversion bug) // Date: 2021/10/06 // Author: Fardad Soleimanloo // Description: // This file tests the DIY section of your workshop /////////////////////////////////////////////////// #include } void typeConversionTest() { Bus bus[] = { {}, {36,20}, {42} }; cout << endl << "Type Conversion operator tests" << endl; for (int i = 0; i < 3; i++) { cout.setf(ios::fixed); cout.precision(2); if (bus[i]) { cout << int(bus[i]) << " Passengers:" << endl; cout << double(bus[i]) << " Dollars total fare." << endl << "---------------" << endl; } else { cout << "This bus is out of service!" << endl; } } cout << "END Type Conversion operator tests" << endl; } void IOTest() { cout << endl << "Operator <<, Operator >>, display and read test" << endl; Bus bus[] = { {}, {25}, {20,25}, {20,-1}, {30,21} }; for (int i = 0; i < 5; i++) { cout << bus[i] << "----------" << endl; } cout << "Enter the following valid values:" << endl << " 22,11" << endl << ">"; cin >> bus[0]; cout << bus[0] << "----------" << endl; cout << "Enter the following valid values:" << endl << " 24,0" << endl << ">"; cin >> bus[0]; cout << bus[0] << "----------" << endl; cout << "Enter the following invalid values:" << endl << " 44,20" << endl << ">"; cin >> bus[0]; cout << bus[0] << "----------" << endl; cout << "Enter the following invalid values:" << endl << " 24,25" << endl << ">"; cin >> bus[0]; cout << bus[0] << "----------" << endl; cout << "END Operator <<, Operator >>, display and read test" << endl; } Execution Sample Operator <<, Operator >>, display and read test __________________________________ | [ ][ ][ ][ ][ ][ ][ ][ ][ ][ ]|_\_ | Seneca College ) `---OO-------------------------O---' Total Fare Price: 0.00 ---------- Out of service! ---------- Out of service! ---------- Out of service! ---------- _________________________________________________ | [2][2][2][2][2][2][2][2][2][2][1][ ][ ][ ][ ]|_\_ | Seneca College ) `---OO----------------------------------------O---' Total Fare Price: 2632.14 ---------- Enter the following valid values: 22,11 >22,11 _____________________________________ | [2][2][2][2][2][1][ ][ ][ ][ ][ ]|_\_ | Seneca College ) `---OO----------------------------O---' Total Fare Price: 1378.74 ---------- Enter the following valid values: 24,0 >24,0 ________________________________________ | [ ][ ][ ][ ][ ][ ][ ][ ][ ][ ][ ][ ]|_\_ | Seneca College ) `---OO-------------------------------O---' Total Fare Price: 0.00 ---------- Enter the following invalid values: 44,20 >44,20 Out of service! ---------- Enter the following invalid values: 24,25 >24,25 Out of service! ---------- END Operator <<, Operator >>, display and read test Type Conversion operator tests 0 Passengers: 0.00 Dollars total fare. --------------- 20 Passengers: 2506.80 Dollars total fare. --------------- This bus is out of service! END Type Conversion operator tests Unary operator tests b1: 10 b2: 0 --b1; --b2; Passenger removed! Bus empty! b1: 9 b2: 0 -------------------------------- ++b1; ++b1; --b2; Passenger added! Bus full! Passenger added! b1: 10 b2: 1 -------------------------------- b1--; b2--; b2--; Passenger removed! Passenger removed! Bus empty! b1: 9 b2: 0 -------------------------------- b1++; b1++; b2++ Passenger added! Bus full! Passenger added! b1: 10 b2: 1 -------------------------------- Binary Member operator tests b1: 0, b2: 7, b3: 8 b1 = b2 += b3; b1: 10, b2: 10, b3: 5 bad += b3; bad: -1, b3: 5 --------------------------------------------- b1 = 20; b2 = 2; b1: -1, b2: 2 b1 = 2; b1: -1 --------------------------------------------- b1 += 1; b2 += 1; b3 += 4; b1: -1, b2: 3, b3: 9 --------------------------------------------- b1: 5, b2: 10, b3: 10 b1: ___________________ | [2][2][1][ ][ ]|_\_ | Seneca College ) `---OO----------O---' Total Fare Price: 626.70 b2: ___________________ | [2][2][2][2][2]|_\_ | Seneca College ) `---OO----------O---' Total Fare Price: 1253.40 b3: _______________________________ | [2][2][2][2][2][ ][ ][ ][ ]|_\_ | Seneca College ) `---OO----------------------O---' Total Fare Price: 1253.40 b1 has 5 passengers and b2 is 10 passengers therefore thier head counts are different b2 has 10 passengers and b3 is 10 passengers therefore thier head counts are equal --------------------------------------------- Binary non-member operator test There are 5 passengers at the bus stop and there are 10 passengers on the bus. When the passengers get off the bus there will be 15 passengers at the bus stop! Reflection Study your final solutions for each deliverable of the workshop, reread the related parts of the course notes, and make sure that you have understood the concepts covered by this workshop. This should take no less than 30 minutes of your time and the result is suggested to be at least 150 words in length. Create a file named reflect.txt that contains your detailed description of the topics that you have learned in completing this workshop and mention any issues that caused you difficulty. You may be asked to talk about your reflection (as a presentation) in class. DIY Submission (part 2) To test and demonstrate the execution of your program use the same data as shown in the output example. Files to Submit Bus.h Bus.cpp w5p2_tester.cpp Submission Process: Upload your source code to your matrix account. Compile and run your code using the g++ compiler as shown above and make sure that everything works properly. Then, run the following command from your account replace profname.proflastname with your professor's Seneca userid replace ?? with your subject code (200 or 244) replace # with the workshop number replace X with the workshop part number (1 or 2) ~profname.proflastname/submit 2??/w#/pX
Step by Step Solution
There are 3 Steps involved in it
Step: 1
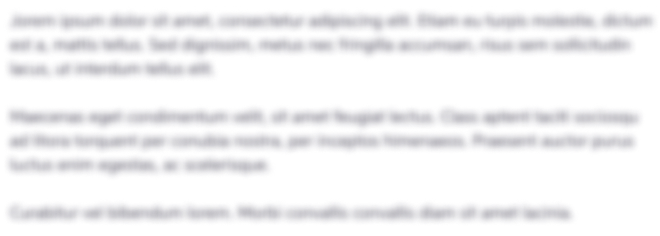
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started