Answered step by step
Verified Expert Solution
Question
1 Approved Answer
do main method for part A and B Part A: Inheritance and Polymorphism Objective: The objective of this assignment is to reinforce your understanding of
do main method for part A and B Part A: Inheritance and Polymorphism
Objective: The objective of this assignment is to reinforce your understanding of method overriding, Inheritance and Polymorphism in Java by implementing it in a scenario involving employee management.
Instructions:
Problem Description: You are tasked with developing an employee management system that allows users to input employee information, perform various operations on the employee data, and display the results. The program should utilize method overloading to handle different scenarios of calculating employee salaries, including the calculation of salaries with and without bonuses.
Class Definition: Define a class called Employee that represents an employee and contains methods for managing employee data:
o Attributes:
name: A String representing the name of the employee.
employeeId: An int representing the employee's ID
salary: A double representing the base salary of the employee.
o Methods:
Employee
String name, int employeeId, double salary
: Constructor method to initialize the attributes.
double calculateSalary
: Method to calculate and return the base salary of the employee.
Derived Classes: Define two derived classes: HourlyEmployee and SalariedEmployee. These classes should inherit from the Employee class.
o HourlyEmployee class:
Attributes:
hoursWorked: A double representing the number of hours worked by the employee.
hourlyRate: A double representing the hourly rate of the employee.
bonus: A double representing the bonus amount of the employee.
Methods:
double calculateSalary
: Override the calculateSalary method to calculate and return the salary of the hourly employee
hoursWorked
hourlyRate
double calculateSalary
double bonus
: Overloaded calculateSalary method to calculate and return the salary of the hourly employee
hoursWorked
hourlyRate
bonus
o SalariedEmployee class:
No need to override the calculateSalary method as it will use the base implementation from the Employee class.
Polymorphic Method: Define a method called printSalary
Employee employee
in a separate class or in the main class. This method should accept an object of the Employee class
or its derived classes
as input and print the employee's name, ID
and salary.
Main Program: In the main program:
o Create instances of the HourlyEmployee and SalariedEmployee classes.
o Call the printSalary method for each instance to display the employee's information and salary.
o Demonstrate the use of method overloading by calculating the salary of an employee with a bonus using the overloaded method.
Bonus Calculation: When the calculateSalary
double bonus
method is called for an employee, it should add the bonus amount to the base salary and return the total salary.
Evaluation Criteria: Your code will be evaluated based on the following criteria:
Correct implementation of the Employee, HourlyEmployee, and SalariedEmployee classes with appropriate methods and attributes.
Proper use of method overloading in the Employee class for calculating salaries.
Proper implementation of inheritance and method overriding in the derived classes.
Clarity and completeness of the printSalary method for displaying employee information.
Correctness of the salary calculations performed by the program, including the use of method overloading and bonus calculation.
Overall code organization, readability, and adherence to Java best practices.
Part B: Exception Handling in Java
Objective: This assignment aims to strengthen your understanding of exception handling in Java by implementing error detection and handling mechanisms in a scenario involving user input validation and data processing.
Instructions:
Description: In this task, you will develop a Java program that demonstrates your understanding of exception handling. You will create a program that simulates a simple banking system, where users can perform transactions such as deposits, withdrawals, and transfers. Your program should handle various types of exceptions gracefully to ensure smooth operation.
Bank Account Class: Define a class named BankAccount with the following attributes and methods:
o Attributes: account number, account holder name, balance.
o Methods: deposit, withdraw, and display account information.
Exception Handling: Implement exception handling in the BankAccount class to handle potential errors:
o Handle the scenario where the withdrawal amount exceeds the account balance by displaying an error message to the user.
o Handle the case of negative deposit or withdrawal amounts by displaying an error message to the user.
o Implement appropriate try
catch blocks in the d
Step by Step Solution
There are 3 Steps involved in it
Step: 1
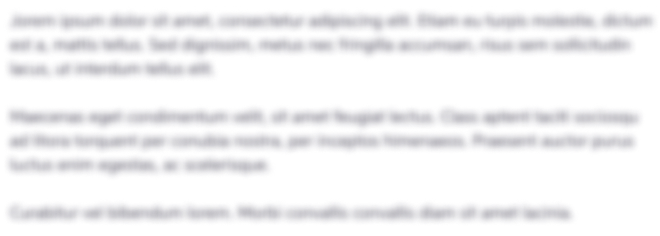
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started