Question
DO NOT CHANGE STARTER CODE Platform Independent Solution Please see Syllabus for more information. Your solution has to be platform-independent; it must run on any
DO NOT CHANGE STARTER CODE
Platform Independent Solution
Please see Syllabus for more information.
Your solution has to be platform-independent; it must run on any platforms including any online IDEs:
You solution should be free of any use of packages. Example, absolutely no
/* Java Program Example - Java Import Packages */ package YOUR_CUSTOM_PACKAGE HERE;
Your solution, regardless of how many classes it has, should be in one .java file.
Your solution has to run on any online IDEs.
If your solution fails the platform-independence criteria, you will have to resubmit work subject to the late penalty.
Your solution should be reusable, meaning it will be invoked and validated against a series of input sequentially to produce consistent outcomes like so:
Solution sol = new Solution(); sol.your_function(test_input_1...); sol.your_function(test_input_2 ...);
Given the uncertainty surrounding the outbreak of the Coronavirus disease (COVID-19) pandemic in 2020, the federal government has to work tirelessly to ensure the distribution of needed resources such as medical essentials, water, food supply among the states, townships, and counties in the time of crisis.
You are a software engineer from the Right Resource, a company that delivers logistic solutions to local and state entities (schools, institutions, government offices, etc). You are working on a software solution to check that given a set of resources, whether they can be proportioned and distributed equally to k medical facilities. Resources are represented as an array of positive integers. You want to see if these resources can be sub-divided into k facilities, each having the same total sum (of resources) as the other facilities. For example with resources = {1,2,3,4,5,6} we can sub-divide them into k = 3 medical facilities, each having the same total sum of 7: {1,6}, {2,5}, and {3,4}.
Starter Code
Write a solution method, canDistribute, that returns whether it is possible (true or false) that a given set of resources divides into k equal-sum facilities. You will solve this problem using a recursion:
public class HW4_1 { public static void main(String[] args) { // your solution will be tested as such, sequentially with random input Solution sol = new Solution(); sol.canDistribute(new int[]{1}, 2); sol.canDistribute(new int[]{1,2,2,3,3,5,5}, 12); // etc. } } /** * PURPOSE: * PARAMETERS: * RETURN VALUES: */ class Solution { public boolean canDistribute(int[] resources, int groups) { // YOUR CODE HERE } }
Examples
input: {3,4,5,6}, 2
output: true
Explanation: {3,6}, {4,5}
input: {1}, 1
output: true
Explanation: {1}
input: {1 , 3 , 2 , 3 , 4 , 1 , 3 , 5 , 2 , 1}, 5
output: true
Explanation: {3,2}, {4,1}, {5}, {2,3}, {1,3,1}
input: {1}, 4
output: false
Explanation: cannot split further with a value of 1 in resource.
Constraints and Assumptions
0 < resources.length() < 1000.
0 < medical facilities < 1000.
A sub-divided facility is never empty.
The solution method returns false if resources cannot be evenly distributed, true otherwise.
There may be more than one ways to sub-divide a given set of resources into k facilities. Doesn't matter. Your solution should return true in that case.
This problem can be solve using iterations. However that is against the spirit of this homework which calls for the use of recursion. You will use a Recursion for this problem. Failure to do so received -4 points.
For this problem, resources CANNOT be sub-divided into more sub-groups. For example if resources = {2,1}, you cannot transform it into resources = {1,1,1}, etc.
Hints
Note: you may choose to solve this problem in your own way or use the following pseudo code.
Check for all boundary conditions to return result immediately if known, e.g., resources.length() = 1 and k = 1, return true, resources.length() = 1 and k = 4, return false, etc.
Find the purported allocation for each group, i.e., allocation = SUM(resources)/k.
Sort the resources in either ascending or descending order.
Create another solution method of your choice to enable recursion.
Remaining Pseudo Code:
Create an empty array of integers with k elements. This is your "memory buffer". At the end of your recursion, you want this memory buffer be filled with equally allocated values, allocation.
Pick the highest resource (one with the highest integral value) in your resources.
Check if the selected resource is <= allocation:
If yes, add that value to the first available element in your memory buffer of k elements. Go to #4.
If not, move on (return false) to allow recursion to go back, servicing the recursive calls already in your memory buffer.
Note: if there's no available space to accommodate the selected resource, it is impossible for resources to be allocated equally.
Advance to the next highest resource. Repeat Step #3. If all resources have been considered and there is no more next highest, go to Step #5.
Check if every element in your memory buffer has the same value. If so you have an evenly allocated distribution - return true. False otherwise.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
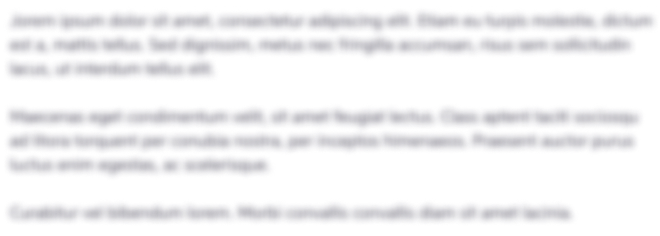
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started