Answered step by step
Verified Expert Solution
Question
1 Approved Answer
do part I in A4Tester java public class A4Tester { private static int testPassCount = 0; private static int testCount = 0; private static double
do part I in A4Tester
java
public class A4Tester { private static int testPassCount = 0; private static int testCount = 0; private static double THRESHOLD = 0.1; // allowable margin of error for floating point results private static A4List list0 = null; private static A4List list1 = null; private static A4List list2 = null; private static A4List list3 = null; public static void main(String[] args) { /* * PART I: * implement and test each of the following methods * found in A4Exercises.java, one at a time */ testAddToAll(); // testArrayContains(); // testSameInARow();
public class A4LinkedList implements A4List { // Completed for you, should not be changed private StudentNode head; private StudentNode tail; private int numElements; // Completed for you, should not be changed public A4LinkedList() { head = null; tail = null; numElements = 0; } // Completed for you, should not be changed public int size(){ return numElements; } // Completed for you, should not be changed public boolean isEmpty() { return head == null; } // Completed for you, should not be changed public void insert(Student s) { StudentNode n = new StudentNode(s); if (head == null) { head = n; } else { tail.next = n; } tail = n; numElements++; } /* * Purpose: create a string representation of list * Parameters: none * Returns: String - the string representation * * Completed for you, should not be changed */ public String toString() { return "{" + toStringRecursive(head) + "}"; } public String toStringRecursive(StudentNode cur) { if (cur == null) { return ""; } else if (cur.next == null) { return cur.getData().toString() + toStringRecursive(cur.next); } else { return cur.getData().toString() + ", " + toStringRecursive(cur.next); } } public boolean inProgram(String program) { return inProgramRecursive(head, program); } private boolean inProgramRecursive(StudentNode cur, String program) { return false; // so it compiles } public Student getStudent(String sID) { return getStudentRecursive(head, sID); } private Student getStudentRecursive(StudentNode cur, String sID) { return null; // so it compiles } public double averageGPA() { if (size() == 0) { return 0.0; } else { return sumGPARecursive(head)/size(); } } private double sumGPARecursive(StudentNode cur) { return 0.0; // so it compiles } public double programAverage(String program) { // call a recursive helper method! return 0.0; } public Student highestGPA() { // call a recursive helper method! return null; } }
public class A4Exercises { /* * Purpose: add valToAdd to all elements in the given array * Parameters: int[] array - the array to modify * int valToAdd - the value to modify items by * Returns: void - nothing */ public static void addToAll(int[] array, int valToAdd) { addToAllRecursive(array, valToAdd, array.length-1); } private static void addToAllRecursive(int[] array, int valToAdd, int i) { } /* * Purpose: determines whether the given array contains toFind * Parameters: int[] array - the array to search * int toFind - the value to search for * Returns: boolean - true if found, false otherwise */ public static boolean arrayContains(int[] array, int toFind) { return arrayContainsRecursive(array, toFind, array.length-1); } private static boolean arrayContainsRecursive(int[] array, int toFind, int i) { return false; // so it compiles } /* * Purpose: gets the number of times there are two of the same element in a row * Parameters: int[] array - the array to search * Returns: int - the number of occurrences where two adjacent elements are the same */ public static int sameInARow(int[] array) { return sameInARowRecursive(array, -1, array.length-1); } private static int sameInARowRecursive(int[] array, int prev, int i) { return 0; // so it compiles } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
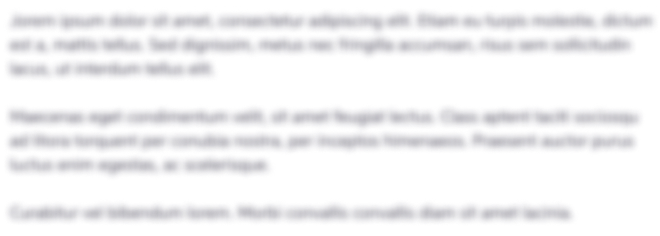
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started