Question
// document analysis project linked list structs // this contains both the word and the number of times the word occurs in the document. struct
// document analysis project linked list structs
// this contains both the word and the number of times the word occurs in the document.
struct word_entry
{
char *unique_word ; // be sure to malloc exactly enough space to hold the string for the word include the '\0'.
int word_count ; // The number of times this word occurs in the input document (by definition at least one).
} ;
//
struct node
{
struct word_entry one_word ;// a word we have seen at least once with the number of occurrences.
struct node *p_previous ; // set to NULL if this node is the first in the list.
struct node *p_next ; // set to NULL if this node is the last in the list
} ;
struct linked_list
{
struct node *p_head ; // points to the first node in the list. NULL if the list is empty.
struct node *p_tail ; // points to the last node in the list. NULL if the list is empty.
struct node *p_current ;// points to the most recently added or accessed record. NULL if the list is empty.
} ;
// linked list function prototypes
extern struct node *create_node( char *word ) ;
extern int add_node_at_head( struct linked_list *p_list, char *word ) ;
extern int clear_linked_list( struct linked_list *p_list ) ;
extern int add_node_after_current( struct linked_list *p_list, char *word ) ;
extern int find_word( struct linked_list *p_list, char *word ) ;
#endif // matches the #ifndef at the beginning that avoids multiple inclusions.
// For the passed linked_list pointer free all of the nodes in the list.
// Be careful to free the space for the string before freeing the node itself.
// Also be careful to save a copy of the pointer to the next item in the list before
// freeing the node.
// Lastly, return the number of nodes freed (which could be zero if p_list indicates an empty list).
int clear_linked_list( struct linked_list *p_list )
{
}
// Inserts a node after the current pointer in the linked list.
// Updates the passed set of pointers as needed based upon the addition that was done.
//
// It checks that p_list is not NULL. Checks that word is not NULL and is not an empty string.
// Returns 0 for failure if either word test fails.
// Also on failure no change is made to the pointers in p_list.
//
// On success it returns a 1 and updates at least the p_current member of p_list. p_current points to the newly added node.
// p_head is updated only if p_current is NULL (an empty list).
// p_tail is updated only if the new node is at the end of the list.
// When adding the first node to an empty list p_tail will also point to this same new node since it is the only node.
//
// Hint: use the create_node function to actually create the node.
// Hint: be sure to maintain both the p_previous and p_next pointers in each node.
// Hint: if this function is called with a p_current that is NULL (meaning the list is empty)
// use the add_node_at_head function to create the new node.
int add_node_after_current( struct linked_list *p_list, char *word )
{
return -1 ; // REMOVE THIS and replace with working code
}
// Searches the linked list for the passed word.
// NOTE -- this function REQUIRES that the linked list has been maintained in English language alphabetical order.
// Definition of match: every letter must match exactly including by case.
// e.g. "A" does NOT match "a". "a " does NOT match "a"
//
// If found it sets the current pointer to the matching node.
// If not found it returns a failure and sets the current pointer to the node just before the
// insertion point (by alphabetic order). Note the special case for a new word that goes at the
// beginning of the list. See the examples below.
//
// Hint: use strcmp to determine sorting order.
//
// If it is found the current pointer is set to the node containing the matching word.
// If it is found this function returns a 1 to indicate success.
//
// If it is not found the current pointer is set to the node just before the insertion point.
// If it is not found the function returns a 0 to indicate failure.
// e.g. the list contains the words "apple", "buy", "cat".
// Searching for "baby" would set current to the node containing "apple".
// Searching for "acid" would set current to NULL to indicate that "acid" belongs at the head.
// searching for "zebra" would set current to the node containing "cat".
//
// Tests on p_list and word: p_list, p_list->head, and word must all not be NULL.
// In addition, word must not be an empty string.
// We must have a valid list with at least one node and we must a valid word to match.
// If any of these conditions are violated this function returns a -1 to indicate invalid input.
int find_word( struct linked_list *p_list, char *word )
{
return -2 ; // REMOVE THIS and replace with working code
}
need clear_linked_list
need add_node_after_current
need find_word
the externs show the input of the functions
Step by Step Solution
There are 3 Steps involved in it
Step: 1
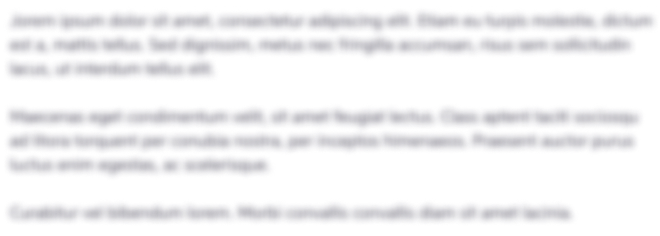
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started