Question
Does this code work? the steps were [ Instructor data is stored in a fixed-format file on disk. Records contain a fixed number of characters,
Does this code work? the steps were [
Instructor data is stored in a fixed-format file on disk. Records contain a fixed number of characters, and have no delimiters between fields or records. Your program is to read the input and populate a set of Instructor objects. Next, it displays the objects, and performs some analysis of the objects using loops and functions. Input Canvas PA2 contains a data file named pa2data. No suffix. Download this file onto your machine. Please keep the file name the same, although the file path on your machine will be different from everybody elses. Records in the file have the following format: Instructor ID payroll code 3 characters number 6 digits First name 10 characters Middle initial 1 character Last Name 15 characters Department code 5 characters Salary 6 digits You may assume that the data is valid, and that there is at least one input record. Instructor class (or struct) Instructor has members for all the input fields. The instructor number and salary members are ints, the middle initial is a char, and the rest are strings. Define a class (or a struct) that matches this description. There are no member functions for Instructor so a struct is probably a better choice. There are some differences between the input fields and the form of the Instructor members. The Instructor ID field in the input is an example of a coded key. It may look like a 9-character ID, but in reality it contains two distinct fields. The first three characters are a payroll code. The next six characters are a unique number. You may assume that the numbers are assigned such that every instructor has a unique 9-character ID. The salary is a whole number (no decimal places).
Processing the input Open the input file. Check for successful open. If the open failed, display an error message and return with value 1. Use a properly-structured loop (as we discussed in class) to read the input file until EOF. Use the read function to read each record into a character array large enough to hold the entire record. For each record, dynamically allocate and populate an Instructor object. Use a pointer array to manage all the created Instructor objects. Note that to populate some of the Instructor fields, youll need to perform a conversion of some type. Assume that there will not be more than 99 input records, so the size of the pointer array will be 100. Use a global variable to define the size of the pointer array. Using a pointer array The technique of using a pointer array to keep track of dynamically allocated objects is described in the dynamic allocation in-class examples we did. Dynamic allocation of structures is also covered in Gaddis chapter 11.9. Initialize each element in the array of pointers to nullptr. As you read input records and create new Instructor objects, point the next element in the pointer array at the new object. Later, when you loop through the pointer array to process the objects, youll know that there are no more when you come across a pointer with a null value. This way, the pointer array is self-contained and you dont need a counter. Display the Instructor objects When you hit EOF on the input file, close it, and display the entire list of instructors. Do not create the report while youre in the loop that reads input records, else you wont be dumping data from the structure youve created. Your output should look something like this: Employee ID Last name MI First Name Department Salary ====================================================================== ABC123456 Anconia D Francisco BUSN 123456 DEF234567 Bulsara B Farrokh MUSC 987654 ... salary function Code a function named salary, which has two parameters and a return value. The parameters are: 1) the Instructor pointer array 2) a pointer variable of type Instructor The return value is an int.
salary performs two bits of analysis. It loops through the Instructor objects, calculating the average salary. It also determines which Instructor has the highest salary. You can perform both analyses in the same loop. The average salary is passed back to the caller as the return value. The address of the Instructor object with the highest salary is passed back to the caller in the second parameter. Mains structure: open the input file if it fails to open, display an error message and terminate read the file and populate the Instructor objects display the Instructor data with appropriate column headers and formatting to make it readable invoke salary display the average salary and the name of the instructor with the highest salary clean up (deallocate memory, close files) return Source code file structure Use the standard approach to source files in this assignment. a .cpp for each function. Youll have at least two main and salary. You could have more if you design your project with more functions. a header file for the Instructor class / struct, properly guarded. a header file for the function prototype for salary, properly guarded. Debugging For debugging purposes, it would be a good idea to code your program in steps. First, write code to read the input and create the Instructor objects. Determine if your parsing works by displaying each Instructor as its created (but remove this display before you submit for a grade). Make sure that if you read n input records, you actually create n objects. Verify that the n+1th pointer in the Instructor pointer array contains nulls. Then, add the code to produce the report from the Instructor objects. Finally, code the salary function, call it, and display its results.
]
#include
#include
#include
#include
using namespace std;
const int MAX_RECORD = 100;
struct instructers{
int instructorID;
int salary;
char middleInital;
string payrollCode;
string firstName;
string lastName;
string departmentCode;
};
int salary(instructers* instructors[], instructers*& highestSalaryInstructor) {
int numInstructors = 0;
int totalSalary = 0;
int highestSalary = 0;
highestSalaryInstructor = nullptr;
while (instructors[numInstructors] != nullptr) {
totalSalary += instructors[numInstructors]->salary;
if (instructors[numInstructors]->salary > highestSalary) {
highestSalary = instructors[numInstructors]->salary;
highestSalaryInstructor = instructors[numInstructors];
}
numInstructors++;
}
if (numInstructors == 0) {
return 0;
} else {
return totalSalary / numInstructors;
}
}
int main() {
ifstream file;
file.open("pa2data");
if(!file.is_open()){
cout << "Error opening the file";
return 1;
}else{
}
instructers* instructors[MAX_RECORD];
char record[MAX_RECORD];
int numInstructors = 0;
for (int i = 0; i < MAX_RECORD; i++) {
instructors[i] = nullptr;
}
if (file.is_open()) {
file.read(record, MAX_RECORD);
while (!file.eof()) {
char CpayrollCode[4], CfirstName[11], CmiddleInitial[2], ClastName[16], CdeptCode[6], Csalary[7];
for (int i = 0; i < 3; i++) {
CpayrollCode[i] = record[i];
}
CpayrollCode[3] = '\0';
for (int i = 3, j = 0; i < 9; i++, j++) {
Csalary[j] = record[i];
}
Csalary[6] = '\0';
for (int i = 9, j = 0; i < 19; i++, j++) {
CfirstName[j] = record[i];
}
CfirstName[10] = '\0';
CmiddleInitial[0] = record[19];
CmiddleInitial[1] = '\0';
for (int i = 20, j = 0; i < 35; i++, j++) {
ClastName[j] = record[i];
}
ClastName[15] = '\0';
for (int i = 35, j = 0; i < 40; i++, j++) {
CdeptCode[j] = record[i];
}
CdeptCode[4] = '\0';
int salary = atoi(Csalary);
instructors[numInstructors] = new instructers;
instructors[numInstructors]->payrollCode = CpayrollCode;
instructors[numInstructors]->firstName = CfirstName;
instructors[numInstructors]->middleInital = CmiddleInitial[0];
instructors[numInstructors]->lastName = ClastName;
instructors[numInstructors]->departmentCode = CdeptCode;
instructors[numInstructors]->salary = salary;
numInstructors++;
file.read(record, MAX_RECORD);
}
file.close();
}
else {
cout << "Error. File failed to open.";
return 1;
}
cout << "Employee ID Last name MI First Name Department Salary " << endl;
cout << "====================================================================== " << endl;
numInstructors = 0;
while (instructors[numInstructors] != nullptr) {
cout << instructors[numInstructors]->payrollCode << " "
<< setw(15) << left << instructors[numInstructors]->lastName << " "
<< instructors[numInstructors]->middleInital << " "
<< setw(10) << left << instructors[numInstructors]->firstName << " "
<< setw(13) << left << instructors[numInstructors]->departmentCode << " "
<< setw(6) << right << instructors[numInstructors]->salary << endl;
numInstructors++;
}
for (int i = 0; instructors[i] != nullptr; i++) {
delete instructors[i];
}
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
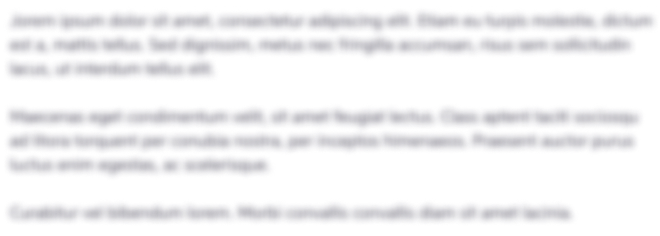
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started