Question
dog: it's better than cat (100pts) Purpose This assignment is meant to give students practice working on projects that contain multiple source code files. It
dog: it's better than cat (100pts) Purpose This assignment is meant to give students practice working on projects that contain multiple source code files. It will require the students to construct a Makefile and appropriate header files. It will also require the use of getopt to handle command line options, and manipulation of data read in from a file. The following functions will be of use to you (and read and write are mandatory). read (section 2) write (section 2) getopt (section 3) Description For this assignment, you will take the cat program you wrote for the last assignment and upgrade it to add more features. We will call it dog instead of cat, because canines are better than felines. These new features will include: The ability to specify a command line option (s x) to change the size of the buffer used for calls to read and write to be x bytes. The ability to specify a command line option (n x) to change the number of bytes read from each file to x. (Instead of reading the whole file as we would have without this option.) The ability to specify a command line option (c x) to have the program apply a Caesar cipher with a shift of x to alphabetical characters in the data read before writing its results. The ability to specify a command line option (r x) to have the program apply a rotation of x to each byte read from the file before sending the data to be output. The ability to specify a command line option (x) to output the data in the file as hexadecimal. The ability to specify a command line option (b) to output in binary notation, most significant bit first. Requirements The main loop should be in your main function in one source code file. The functions that process the data (binary/hex/Caesar cipher/rotation) should be found in another file. Their declarations will need to be in a header file, so that they can be called from main even though they will be implemented in that other file. You must make a Makefile that has rules sufficient to compile your program when a user types make in the same directory as your source code. Only files that have changed or that have had their dependencies change should be recompiled. You must use the system calls read for the input from the files and write for the output. You may use cerr and perror to display error messages, but cout is disallowed, as are printf and fprintf, for the output data.
Obviously, your source code must be well documented. If it is not, there will be a large point penalty. This requirement holds for all coding assignments in this course, whether this line is in the description of the assignment or not. The features enabled by the command line options listed in the description section above must be implemented. Note: If both r and c are specified, the behavior can change depending on which is executed first. Dont allow a user to specify both. Give them an error message and exit if they try. Also: Binary and hexadecimal notation flags are mutually exclusive, as it would not be possible to represent the data as both simultaneously. If both binary and hexadecimal modes are specified, it is an error. Print a warning and quit. The other command line parameters can all be on at the same time without any problem. Make sure that they work together. If no command line options are specified, this program should behave the same as cat would have, as was specified in the previous program. Caesar cipher A Caesar cipher is a very primitive method of encrypting text data. It involves taking the letters of the input and shifting them upwards alphabetically by some number of letters. This number is given as the option parameter in the command line option that turns on the Caesar shift. If that parameter was not an integer, do not perform any shift. Only letters (uppercase and lowercase) should have any shift applied at all. Leave any other characters untouched. You should assume the files are using the ASCII encoding for this purpose. (man 7 ascii).
Binary rotation While the Caesar cipher above was interpreting the bytes that you read as text, changing only alphabetical characters, this option should apply to any value, alphabetical or not. The principle is the same, but instead of applying it to AZ and az, it is applied to each one-byte value interpreted as 0255. Hexadecimal representation All numbers are stored as collections of binary bits on the computer. A char is one byte long, which is 8 bits. When dealing with files that are not meant to be read by a human (binary mode as opposed to text mode), it can be convenient to encode the data in a format called hexadecimal.
Hexadecimal,thebase-16numbersystem,isusefulbecauseitisbasedonapowerof2(24= 16),and so each hexadecimal digit represents 4 bits. Numbers represented as decimal (base-10) do not line up in the same way, so it can be hard to know which bits are on and which are off.Eachbyteis8bits,andcanberepresentedas2hexdigits. Your program should be able to output the data in this format(2hexdigitsperinputbyte)when ever the x command line parameter is enabled. Keepinmindthattherewillbetwocharactersofoutputforeverycharacterofinputinthismode.Since you are not allowed to use cout for the output, you wont be able to use the hexIO manipulator to accomplish his. Likewise,functionsfromtheprintffamilyusingaformatof%xarenotpermittedeither.Hint: You can use the bitwise operators in C++(&,|)toisolatethehighorder4bitsfromtheloworder4bitsinabyte,andthebitshiftoperators(>>,
Every byte is made up of 8 bits, 0 to 7 . When interpreting them as an unsigned number, each bit, answers the question of whether 2 is part of the number represented. When you output the data in binary format, you will output a 1 if the bit is true, or a 0 if the bit is false, for all 8 bits, starting from the most significant one (7 , which represents 128). Notice that the output data will be eight times longer than the input data. Some examples of output % cat file1 Hello World
% ./dog file1 Hello World % ./dog n 5 file1 Hello % ./dog n 5 c 1 file1 Ifmmp % ./dog n 5 x file1 65486c6c6f % ./dog n 5 b file1 0110010101001000011011000110110001101111 What to turn in? Submit, through Blackboard, the following files: The C++ source file containing your main function. The C++ source file containing the functions implementing the new features added. A C++ header file (.h) containing the declarations for each of the functions implementing the new features. A Makefile set up as shown in class. The rules should be set up so that only the files that have changed need to be recompiled.
Caesar Shift of 1 Caesar Shift of 2 Figure 1: A diagram of the basic Caesar shift cipher for shifts of one and two. 0-255 5 3 N N 24 23 | 24 22 24 21 24 20 Decimal Hex Binary 0 0 0000 1 1 0001 2 0010 3 3 0011 4 0100 0101 0110 7 0111 8 1000 9 91001 A 1010 11 B 1011 12 C 1100 13 D 1101 14 E 1110 15 F 1111 24 * Figure 2: A diagram illustrating how the bits of a byte can be represented in hexadecimal notation. BINARY REPRESENTATION value = 128b+ 64b6 + 32b3 +1664 + 8b3 + 4b2 + 2b+ 1b Caesar Shift of 1 Caesar Shift of 2 Figure 1: A diagram of the basic Caesar shift cipher for shifts of one and two. 0-255 5 3 N N 24 23 | 24 22 24 21 24 20 Decimal Hex Binary 0 0 0000 1 1 0001 2 0010 3 3 0011 4 0100 0101 0110 7 0111 8 1000 9 91001 A 1010 11 B 1011 12 C 1100 13 D 1101 14 E 1110 15 F 1111 24 * Figure 2: A diagram illustrating how the bits of a byte can be represented in hexadecimal notation. BINARY REPRESENTATION value = 128b+ 64b6 + 32b3 +1664 + 8b3 + 4b2 + 2b+ 1bStep by Step Solution
There are 3 Steps involved in it
Step: 1
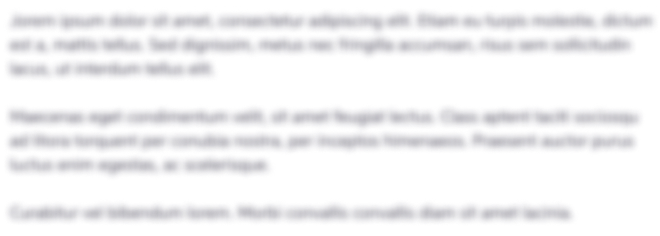
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started