Question
Download and run the attached program. For input you can use the temperature data files provided previously. When the program runs it puts up a
Download and run the attached program. For input you can use the temperature data files provided previously. When the program runs it puts up a window. Click the Browse button and open a temperature data file to plot. The purple curve is a smoothed version of the daily data points. The smoothing can be changed by entering a different number into the smoothing box and clicking the Plot button. The sensitivity of the peak finding can be changed by changing the Peak size and clicking Plot.
The program contains a function named find_local_min_max(y, delta) that finds the peaks/valleys. Write a new function named find_local_min_max2(y, delta) that counts a point as a peak if it is bigger than the points within delta distance before or after it. If delta = 2, the y[9] is a peak if it is bigger than y[8]m y[7], y[10] and y[11]. Use a similar strategy to find valleys. Think about how to handle points near the beginning and end of the data. Can you think of any flaws with this peak/valley algorithm. Write a version of the program that replaces the call to the original peak/valley finder with a call to your function. Run both programs and compare the results.
ATTACHED PROGRAM BELOW
Created on Thu Oct 13 08:31:01 2016
Reads temperarure data from input text file,
plots raw data, smoothed data and uses hysteresis based method
to find local temperature min/max.
@author: rutkowsk
"""
from tkinter import *
from tkinter.filedialog import *
#import numpy as np
import matplotlib
matplotlib.use('TkAgg')
from matplotlib.backends.backend_tkagg import FigureCanvasTkAgg, NavigationToolbar2TkAgg
import matplotlib.pyplot as plt
#================================================================
# smooth data by averaging with neighbor points
def smooth(x):
y = []
y.append((x[0] + x[1])/2)
for i in range(1,len(x) - 1):
y.append((x[i-1] + x[i] + x[i+1])/3)
y.append((x[-1] + x[-2])/2)
return y
#================================================================
# find peaks using hysteresis
def find_local_min_max(y, delta):
y_lo = y[0] - delta
y_hi = y[0] + delta
hi_list = []
lo_list = []
direction = None
for i in range(len(y)):
if y[i] > y_hi:
y_hi = y[i]
y_lo = y_hi - 2*delta
if direction == 'down':
lo_list.append(i_last)
direction = 'up'
i_last = i
elif y[i] < y_lo:
y_lo = y[i]
y_hi = y_lo + 2*delta
if direction == 'up':
hi_list.append(i_last)
direction = 'down'
i_last = i
return lo_list, hi_list
#================================================================
# get input data from file
def get_data(fname):
f_in = open(fname, "r")
temp = []
prev_temp = 0
for s in f_in.readlines():
curr_temp = float(s.split()[3])
if curr_temp > -99: # fix -99 missing data marker
prev_temp = curr_temp
else:
curr_temp = prev_temp
temp.append(curr_temp)
return temp
#================================================================
# set plot parameters
def set_plot_params(plt):
#plt.ion() # interactive mode on
plt.rcParams["figure.figsize"] = (14, 7)
plt.rcParams['axes.facecolor'] = 'ivory'
plt.rcParams['figure.facecolor'] = 'powderblue'
plt.rcParams['figure.titlesize'] = 'large'
#================================================================
# plot raw data, smoothed data, peaks/valleys
def plot_data(x, y, hyst, smooth_count):
plt.clf()
plt.xlim(0, len(y) - 1)
plt.title(inputFileName.get())
plt.xlabel("Day Number")
plt.ylabel("Temperature Degrees F")
#--------------------------------------------
# plot original data
plt.plot(x, y, color='g', label='Raw Data')
#--------------------------------------------
# find/plot peaks/valleys
lo_plist, hi_plist = find_local_min_max(y, hyst)
peaky = [y[i] for i in hi_plist] # local highs
plt.plot(hi_plist, peaky, 'rs', label='Local Highs')
peaky = [y[i] for i in lo_plist] # local lows
plt.plot(lo_plist, peaky, 'bs', label='Local Lows')
#--------------------------------------------
# smooth data
s2 = smooth(y) # smooth data
for i in range(smooth_count):
s2 = smooth(s2)
plt.plot(x, s2, color='m', label ='Smoothed Data', linewidth = 3.0)
#--------------------------------------------
# setup the legend
plt.legend();
legend = plt.legend()
fr = legend.get_frame()
fr.set_facecolor('wheat')
fr.set_edgecolor('black')
#plt.show()
canvas.show()
#================================================================
# GUI
#================================================================
root = Tk()
root.title('Simple Plot')
# a tk.DrawingArea
set_plot_params(plt)
canvas = FigureCanvasTkAgg(plt.figure(), master=root)
canvas.show()
canvas.get_tk_widget().pack(side=TOP, fill=BOTH, expand=1)
# data file
row = Frame(root)
row.pack(side=TOP, fill=X, padx=5, pady=5)
Label(row, text = 'Input File').pack(side=LEFT, expand=NO, fill=X, padx=15)
inputFileName = StringVar()
Entry(row, textvariable = inputFileName).pack(side=LEFT, expand=YES, fill=X, padx=15)
# event handler
def browseInput():
inputFileName.set(askopenfilename(title="Select input file", filetypes=[("*.TXT", "*.txt")]))
temp = get_data(inputFileName.get())
plot_data(range(len(temp)), temp, hyster.get(), smooth_iter.get())
#canvas.show()
# browse input button
Button(row, fg ="blue" , text = "Browse", width = 10, command = browseInput).pack(side=LEFT, expand=NO, fill=X, padx=15)
# smoothing/peak finding parameters
row = Frame(root)
row.pack(side=TOP, fill=X, padx=5, pady=5)
Label(row, text = 'Smoothing').pack(side=LEFT, expand=NO, fill=X, padx=15)
smooth_iter = IntVar()
smooth_iter.set(100)
Entry(row, textvariable = smooth_iter).pack(side=LEFT, expand=YES, fill=X, padx=15)
Label(row, text = 'Peak Size').pack(side=LEFT, expand=NO, fill=X, padx=15)
hyster = DoubleVar()
hyster.set(5.0)
Entry(row, textvariable = hyster).pack(side=LEFT, expand=YES, fill=X, padx=15)
# event handler
def new_plot():
temp = get_data(inputFileName.get())
plot_data(range(len(temp)), temp, hyster.get(), smooth_iter.get())
#canvas.show()
# plot button
Button(row, fg ="blue" , text = "Plot", width = 10, command = new_plot).pack(side=LEFT, expand=NO, fill=X, padx=15)
#set_plot_params()
root.mainloop()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
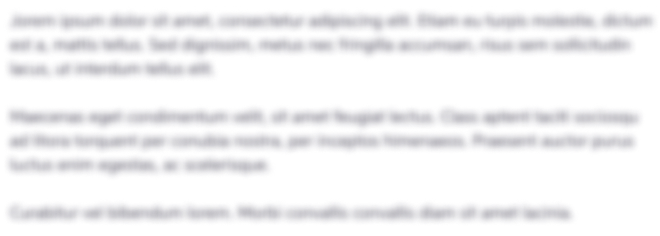
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started