Question
Download thesecode files download for a program that deals poker hands. You'll need to understand the logic and data structures in the program to correct
Download thesecode files
download
for a program that deals poker hands. You'll need to understand the logic and data structures in the program to correct some errors and make the following modifications.
The executable file is included (run it at the Windows command prompt) so you know how the correctly debugged program works.
WHAT TO DO:
1) Update the comment boxes at the top of each function in the .cpp files.
2) Fix the syntax and logic errors in the program.
3) Modify the console output to include ASCII art representations of the cards dealt instead of text output messages. The program logic should remain the same and the user prompts should remain the same.
Card.cpp
#include "card.h"
/************************************************************
*FunctionName*
* Function description*
**
**
************************************************************/
// get() --- Get card value
// - return the value from the member variable
void Card::get()
{
return m_cardValue;
}
/************************************************************
*FunctionName*
* Function description*
**
**
************************************************************/
// set() --- Set card value
// - set the value of the member variable
void Card::set(int newCardVal)
{
m_cardValue = newCardVal;
}
/************************************************************
*FunctionName*
* Function description*
**
**
************************************************************/
string Card::getPip()
{
return pips[m_cardValue % 13];
}
/************************************************************
*FunctionName*
* Function description*
**
**
************************************************************/
string Card::getSuit()
{
return suits[m_cardValue / 13];
}
/************************************************************
*FunctionName*
* Function description*
**
**
************************************************************/
// print() --- Print card value
// - print the card's value
// - Example display if member variable m_cardValue is 0
// - it should print "Ace of Hearts"
void Card::print()
{
int suit_number = m_cardValue / 13;
int pip_number = m_cardValue % 13;
cout << pips[pip_number] << " of " << suits[suit_number];
}
Main.cpp
#include
#include
#include
#include
#include
#include "card.h"
#include "deck.h"
#include "hand.h"
using namespace std;
/************************************************************
*FunctionName*
* Function description*
**
**
************************************************************/
int main()
{
string repeat = "Y";
Deck myDeck;
Hand myHand;
string exchangeCards;
while (repeat == "Y" || repeat == "y")
{
cout << endl;
myHand.newHand(myDeck);
myHand.print();
cout << endl;
cout << "Would you like to exchange any cards? [Y / N]: ";
getline(cin, exchangeCards);
while (exchangeCards != "Y" && exchangeCards != "y" && exchangeCards != "X" && exchangeCards != "n")
{
cout << "Please enter Y or N only: ";
getline(cin, exchangeCards);
}
if (exchangeCards = "Y" || exchangeCards = "y")
{
myHand.exchangeCards(myDeck);
}
cout << endl;
myHand.print();
cout << endl;
myDeck.reset();// Resets the deck for a new game
cout << "Play again? [Y / N]: ";
getline(cin, repeat);
while (repeat != "Y" && repeat != "y" && repeat != "N" && repeat != "n")
{
cout << "Please enter Y or N only: ";
getline(cin, repeat);
}
}
return 0;
}
Hand.cpp
#include "pokerHand.h"
/************************************************************
*FunctionName*
* Function description*
**
**
************************************************************/
// newCard() --- Put new card from deck into given location in the hand
// 1. deal a card from the deck
// 2. assign the card to the location in the hand array
void Hand::newCard(Deck& deck, int location)
{
Card new_card = deck.dealCard();
m_hand[location] = new_card;
}
/************************************************************
*FunctionName*
* Function description*
**
**
************************************************************/
// newHand() --- Get all new cards
// 1. loop for zero to the number of cards on hand (NUM_CARDS_ON_HAND)
// a. get a new card by calling another member function using the loop counter as the location
void Hand::newHand(Deck& deck)
{
for (int i=0; i < NUM_CARDS_ON_HAND; i++)
{
Hand::newCard(deck, i);
}
}
/************************************************************
*FunctionName*
* Function description*
**
**
************************************************************/
// exchangeCards() --- Determine cards to exchange and exchange them
// 1. declare an integer variable to store number of exchanges
// 2. Prompt and ask the user for how many cards to exchange between [1 to 5] (make sure to validate user input)
// 3. loop based on the number of exchanges to make
// declare integer position
// if user wants all card replaced, then
// position = loop counter
// otherwise,
// Prompt and ask the user for the position of the card on hand to exchange (make sure to validate user input)
// get a new card and replace the card at the identified position
void Hand::exchangeCards(Deck& deck)
{
int num_of_exchanges;
cout << "How many cards would you like to exchange (1-5): "; // gets number of cards to be exchanged
cin >> num_of_exchanges;
while (num_of_exchanges > 5 || num_of_exchanges < 1) // validates input
{
cout << "Please enter a number (1-5): ";
cin >> num_of_exchanges;
}
for (int i=0; i < num_of_exchanges; i++) // looping through number of exchanges
{
int position = i; // positioned to be changed is i
if (num_of_exchanges != NUM_CARDS_ON_HAND) // if number of exchanges is less than total hand count
{
cout << "Enter a position in hand of card to exchange: ";
cin >> position; // gets position to be replaced
while (position > 5 || position < 1) // validates input
{
cout << "Please enter a number (1-5): ";
cin >> position;
}
position--;
}
Hand::newCard(deck, position); // replaces card at position
}
}
/************************************************************
*FunctionName*
* Function description*
**
**
************************************************************/
// print() --- print the hand
// 1. loop for zero to the number of cards on hand
// a. print out location of the card on hand followed by
// b. print card pip value and card suit value
void Hand::print()
{
for (int i=0; i < NUM_CARDS_ON_HAND; i++)
{
cout << "Card " << i+1 << " is the ";
m_hand[i].print();
cout << endl;
}
}
Hand.h
#ifndef HAND_H
#define HAND_H
#include
#include "card.h"// Include card header file here
#include "deck.h"// Include deck header file here
using namespace std;
const int NUM_CARDS_ON_HAND = 5;
class Hand
{
public:
// Put new card from deck into given location in a hand
void newCard(Deck& deck, int location);
// Get all new cards
void newHand(Deck& deck);
// Determine cards to exchange and exchange them in a hand
void exchangeCards(Deck& deck);
// Print the hand
void print();
private:
Card m_hand[NUM_CARDS_ON_HAND];// A hand consists of 5 cards
};
#endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
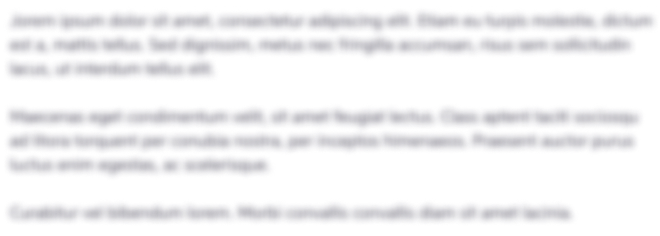
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started